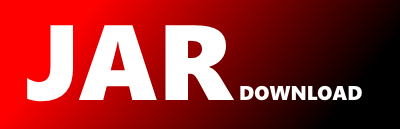
com.pulumi.awsnative.datasync.kotlin.LocationHdfs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.datasync.kotlin
import com.pulumi.awsnative.datasync.kotlin.enums.LocationHdfsAuthenticationType
import com.pulumi.awsnative.datasync.kotlin.outputs.LocationHdfsNameNode
import com.pulumi.awsnative.datasync.kotlin.outputs.LocationHdfsQopConfiguration
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.datasync.kotlin.enums.LocationHdfsAuthenticationType.Companion.toKotlin as locationHdfsAuthenticationTypeToKotlin
import com.pulumi.awsnative.datasync.kotlin.outputs.LocationHdfsNameNode.Companion.toKotlin as locationHdfsNameNodeToKotlin
import com.pulumi.awsnative.datasync.kotlin.outputs.LocationHdfsQopConfiguration.Companion.toKotlin as locationHdfsQopConfigurationToKotlin
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin as tagToKotlin
/**
* Builder for [LocationHdfs].
*/
@PulumiTagMarker
public class LocationHdfsResourceBuilder internal constructor() {
public var name: String? = null
public var args: LocationHdfsArgs = LocationHdfsArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend LocationHdfsArgsBuilder.() -> Unit) {
val builder = LocationHdfsArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): LocationHdfs {
val builtJavaResource = com.pulumi.awsnative.datasync.LocationHdfs(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return LocationHdfs(builtJavaResource)
}
}
/**
* Resource schema for AWS::DataSync::LocationHDFS.
*/
public class LocationHdfs internal constructor(
override val javaResource: com.pulumi.awsnative.datasync.LocationHdfs,
) : KotlinCustomResource(javaResource, LocationHdfsMapper) {
/**
* ARN(s) of the agent(s) to use for an HDFS location.
*/
public val agentArns: Output>
get() = javaResource.agentArns().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* The authentication mode used to determine identity of user.
*/
public val authenticationType: Output
get() = javaResource.authenticationType().applyValue({ args0 ->
args0.let({ args0 ->
locationHdfsAuthenticationTypeToKotlin(args0)
})
})
/**
* Size of chunks (blocks) in bytes that the data is divided into when stored in the HDFS cluster.
*/
public val blockSize: Output?
get() = javaResource.blockSize().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The Base64 string representation of the Keytab file.
*/
public val kerberosKeytab: Output?
get() = javaResource.kerberosKeytab().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The string representation of the Krb5Conf file, or the presigned URL to access the Krb5.conf file within an S3 bucket.
*/
public val kerberosKrb5Conf: Output?
get() = javaResource.kerberosKrb5Conf().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The unique identity, or principal, to which Kerberos can assign tickets.
*/
public val kerberosPrincipal: Output?
get() = javaResource.kerberosPrincipal().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The identifier for the Key Management Server where the encryption keys that encrypt data inside HDFS clusters are stored.
*/
public val kmsKeyProviderUri: Output?
get() = javaResource.kmsKeyProviderUri().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The Amazon Resource Name (ARN) of the HDFS location.
*/
public val locationArn: Output
get() = javaResource.locationArn().applyValue({ args0 -> args0 })
/**
* The URL of the HDFS location that was described.
*/
public val locationUri: Output
get() = javaResource.locationUri().applyValue({ args0 -> args0 })
/**
* An array of Name Node(s) of the HDFS location.
*/
public val nameNodes: Output>
get() = javaResource.nameNodes().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
locationHdfsNameNodeToKotlin(args0)
})
})
})
/**
* The Quality of Protection (QOP) configuration specifies the Remote Procedure Call (RPC) and data transfer protection settings configured on the Hadoop Distributed File System (HDFS) cluster. If `QopConfiguration` isn't specified, `RpcProtection` and `DataTransferProtection` default to `PRIVACY` . If you set `RpcProtection` or `DataTransferProtection` , the other parameter assumes the same value.
*/
public val qopConfiguration: Output?
get() = javaResource.qopConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> locationHdfsQopConfigurationToKotlin(args0) })
}).orElse(null)
})
/**
* Number of copies of each block that exists inside the HDFS cluster.
*/
public val replicationFactor: Output?
get() = javaResource.replicationFactor().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The user name that has read and write permissions on the specified HDFS cluster.
*/
public val simpleUser: Output?
get() = javaResource.simpleUser().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The subdirectory in HDFS that is used to read data from the HDFS source location or write data to the HDFS destination.
*/
public val subdirectory: Output?
get() = javaResource.subdirectory().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* An array of key-value pairs to apply to this resource.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> tagToKotlin(args0) })
})
}).orElse(null)
})
}
public object LocationHdfsMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.datasync.LocationHdfs::class == javaResource::class
override fun map(javaResource: Resource): LocationHdfs = LocationHdfs(
javaResource as
com.pulumi.awsnative.datasync.LocationHdfs,
)
}
/**
* @see [LocationHdfs].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [LocationHdfs].
*/
public suspend fun locationHdfs(
name: String,
block: suspend LocationHdfsResourceBuilder.() -> Unit,
): LocationHdfs {
val builder = LocationHdfsResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [LocationHdfs].
* @param name The _unique_ name of the resulting resource.
*/
public fun locationHdfs(name: String): LocationHdfs {
val builder = LocationHdfsResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy