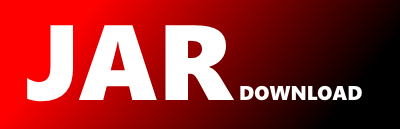
com.pulumi.awsnative.datasync.kotlin.LocationObjectStorageArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.datasync.kotlin
import com.pulumi.awsnative.datasync.LocationObjectStorageArgs.builder
import com.pulumi.awsnative.datasync.kotlin.enums.LocationObjectStorageServerProtocol
import com.pulumi.awsnative.kotlin.inputs.TagArgs
import com.pulumi.awsnative.kotlin.inputs.TagArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Resource schema for AWS::DataSync::LocationObjectStorage.
* ## Example Usage
* ### Example
* No Java example available.
* @property accessKey Optional. The access key is used if credentials are required to access the self-managed object storage server.
* @property agentArns The Amazon Resource Name (ARN) of the agents associated with the self-managed object storage server location.
* @property bucketName The name of the bucket on the self-managed object storage server.
* @property secretKey Optional. The secret key is used if credentials are required to access the self-managed object storage server.
* @property serverCertificate X.509 PEM content containing a certificate authority or chain to trust.
* @property serverHostname The name of the self-managed object storage server. This value is the IP address or Domain Name Service (DNS) name of the object storage server.
* @property serverPort The port that your self-managed server accepts inbound network traffic on.
* @property serverProtocol The protocol that the object storage server uses to communicate.
* @property subdirectory The subdirectory in the self-managed object storage server that is used to read data from.
* @property tags An array of key-value pairs to apply to this resource.
*/
public data class LocationObjectStorageArgs(
public val accessKey: Output? = null,
public val agentArns: Output>? = null,
public val bucketName: Output? = null,
public val secretKey: Output? = null,
public val serverCertificate: Output? = null,
public val serverHostname: Output? = null,
public val serverPort: Output? = null,
public val serverProtocol: Output? = null,
public val subdirectory: Output? = null,
public val tags: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.datasync.LocationObjectStorageArgs =
com.pulumi.awsnative.datasync.LocationObjectStorageArgs.builder()
.accessKey(accessKey?.applyValue({ args0 -> args0 }))
.agentArns(agentArns?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.bucketName(bucketName?.applyValue({ args0 -> args0 }))
.secretKey(secretKey?.applyValue({ args0 -> args0 }))
.serverCertificate(serverCertificate?.applyValue({ args0 -> args0 }))
.serverHostname(serverHostname?.applyValue({ args0 -> args0 }))
.serverPort(serverPort?.applyValue({ args0 -> args0 }))
.serverProtocol(serverProtocol?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.subdirectory(subdirectory?.applyValue({ args0 -> args0 }))
.tags(
tags?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [LocationObjectStorageArgs].
*/
@PulumiTagMarker
public class LocationObjectStorageArgsBuilder internal constructor() {
private var accessKey: Output? = null
private var agentArns: Output>? = null
private var bucketName: Output? = null
private var secretKey: Output? = null
private var serverCertificate: Output? = null
private var serverHostname: Output? = null
private var serverPort: Output? = null
private var serverProtocol: Output? = null
private var subdirectory: Output? = null
private var tags: Output>? = null
/**
* @param value Optional. The access key is used if credentials are required to access the self-managed object storage server.
*/
@JvmName("nmtbnfygvlyqpicy")
public suspend fun accessKey(`value`: Output) {
this.accessKey = value
}
/**
* @param value The Amazon Resource Name (ARN) of the agents associated with the self-managed object storage server location.
*/
@JvmName("lwtpdphykuwpfqgq")
public suspend fun agentArns(`value`: Output>) {
this.agentArns = value
}
@JvmName("fkncctjewtplrxvv")
public suspend fun agentArns(vararg values: Output) {
this.agentArns = Output.all(values.asList())
}
/**
* @param values The Amazon Resource Name (ARN) of the agents associated with the self-managed object storage server location.
*/
@JvmName("hwyaikpywlvalpgl")
public suspend fun agentArns(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy