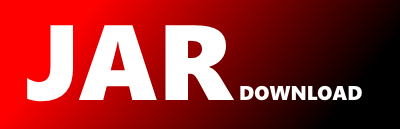
com.pulumi.awsnative.deadline.kotlin.outputs.GetFleetResult.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.deadline.kotlin.outputs
import com.pulumi.awsnative.deadline.kotlin.enums.FleetStatus
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.core.Either
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property arn The Amazon Resource Name (ARN) assigned to the fleet.
* @property capabilities
* @property configuration The configuration details for the fleet.
* @property description A description that helps identify what the fleet is used for.
* @property displayName The display name of the fleet summary to update.
* > This field can store any content. Escape or encode this content before displaying it on a webpage or any other system that might interpret the content of this field.
* @property fleetId The fleet ID.
* @property maxWorkerCount The maximum number of workers specified in the fleet.
* @property minWorkerCount The minimum number of workers in the fleet.
* @property roleArn The IAM role that workers in the fleet use when processing jobs.
* @property status The status of the fleet.
* @property tags An array of key-value pairs to apply to this resource.
* @property workerCount The number of workers in the fleet summary.
*/
public data class GetFleetResult(
public val arn: String? = null,
public val capabilities: FleetCapabilities? = null,
public val configuration: Either? =
null,
public val description: String? = null,
public val displayName: String? = null,
public val fleetId: String? = null,
public val maxWorkerCount: Int? = null,
public val minWorkerCount: Int? = null,
public val roleArn: String? = null,
public val status: FleetStatus? = null,
public val tags: List? = null,
public val workerCount: Int? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.awsnative.deadline.outputs.GetFleetResult): GetFleetResult = GetFleetResult(
arn = javaType.arn().map({ args0 -> args0 }).orElse(null),
capabilities = javaType.capabilities().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.deadline.kotlin.outputs.FleetCapabilities.Companion.toKotlin(args0)
})
}).orElse(null),
configuration = javaType.configuration().map({ args0 ->
args0.transform(
{ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.deadline.kotlin.outputs.FleetConfiguration0Properties.Companion.toKotlin(args0)
})
},
{ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.deadline.kotlin.outputs.FleetConfiguration1Properties.Companion.toKotlin(args0)
})
},
)
}).orElse(null),
description = javaType.description().map({ args0 -> args0 }).orElse(null),
displayName = javaType.displayName().map({ args0 -> args0 }).orElse(null),
fleetId = javaType.fleetId().map({ args0 -> args0 }).orElse(null),
maxWorkerCount = javaType.maxWorkerCount().map({ args0 -> args0 }).orElse(null),
minWorkerCount = javaType.minWorkerCount().map({ args0 -> args0 }).orElse(null),
roleArn = javaType.roleArn().map({ args0 -> args0 }).orElse(null),
status = javaType.status().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.deadline.kotlin.enums.FleetStatus.Companion.toKotlin(args0)
})
}).orElse(null),
tags = javaType.tags().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin(args0)
})
}),
workerCount = javaType.workerCount().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy