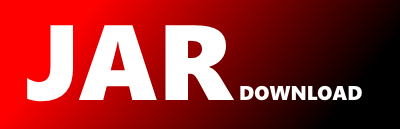
com.pulumi.awsnative.devicefarm.kotlin.NetworkProfileArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.devicefarm.kotlin
import com.pulumi.awsnative.devicefarm.NetworkProfileArgs.builder
import com.pulumi.awsnative.kotlin.inputs.TagArgs
import com.pulumi.awsnative.kotlin.inputs.TagArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* AWS::DeviceFarm::NetworkProfile creates a new DF Network Profile
* @property description The description of the network profile.
* @property downlinkBandwidthBits The data throughput rate in bits per second, as an integer from 0 to 104857600.
* @property downlinkDelayMs Delay time for all packets to destination in milliseconds as an integer from 0 to 2000.
* @property downlinkJitterMs Time variation in the delay of received packets in milliseconds as an integer from 0 to 2000.
* @property downlinkLossPercent Proportion of received packets that fail to arrive from 0 to 100 percent.
* @property name The name of the network profile.
* @property projectArn The Amazon Resource Name (ARN) of the specified project.
* @property tags An array of key-value pairs to apply to this resource.
* For more information, see [Tag](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-resource-tags.html) in the *guide* .
* @property uplinkBandwidthBits The data throughput rate in bits per second, as an integer from 0 to 104857600.
* @property uplinkDelayMs Delay time for all packets to destination in milliseconds as an integer from 0 to 2000.
* @property uplinkJitterMs Time variation in the delay of received packets in milliseconds as an integer from 0 to 2000.
* @property uplinkLossPercent Proportion of transmitted packets that fail to arrive from 0 to 100 percent.
*/
public data class NetworkProfileArgs(
public val description: Output? = null,
public val downlinkBandwidthBits: Output? = null,
public val downlinkDelayMs: Output? = null,
public val downlinkJitterMs: Output? = null,
public val downlinkLossPercent: Output? = null,
public val name: Output? = null,
public val projectArn: Output? = null,
public val tags: Output>? = null,
public val uplinkBandwidthBits: Output? = null,
public val uplinkDelayMs: Output? = null,
public val uplinkJitterMs: Output? = null,
public val uplinkLossPercent: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.devicefarm.NetworkProfileArgs =
com.pulumi.awsnative.devicefarm.NetworkProfileArgs.builder()
.description(description?.applyValue({ args0 -> args0 }))
.downlinkBandwidthBits(downlinkBandwidthBits?.applyValue({ args0 -> args0 }))
.downlinkDelayMs(downlinkDelayMs?.applyValue({ args0 -> args0 }))
.downlinkJitterMs(downlinkJitterMs?.applyValue({ args0 -> args0 }))
.downlinkLossPercent(downlinkLossPercent?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.projectArn(projectArn?.applyValue({ args0 -> args0 }))
.tags(tags?.applyValue({ args0 -> args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) }) }))
.uplinkBandwidthBits(uplinkBandwidthBits?.applyValue({ args0 -> args0 }))
.uplinkDelayMs(uplinkDelayMs?.applyValue({ args0 -> args0 }))
.uplinkJitterMs(uplinkJitterMs?.applyValue({ args0 -> args0 }))
.uplinkLossPercent(uplinkLossPercent?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [NetworkProfileArgs].
*/
@PulumiTagMarker
public class NetworkProfileArgsBuilder internal constructor() {
private var description: Output? = null
private var downlinkBandwidthBits: Output? = null
private var downlinkDelayMs: Output? = null
private var downlinkJitterMs: Output? = null
private var downlinkLossPercent: Output? = null
private var name: Output? = null
private var projectArn: Output? = null
private var tags: Output>? = null
private var uplinkBandwidthBits: Output? = null
private var uplinkDelayMs: Output? = null
private var uplinkJitterMs: Output? = null
private var uplinkLossPercent: Output? = null
/**
* @param value The description of the network profile.
*/
@JvmName("ednxawthsvhsbbck")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The data throughput rate in bits per second, as an integer from 0 to 104857600.
*/
@JvmName("ijkcyhlrxahydakn")
public suspend fun downlinkBandwidthBits(`value`: Output) {
this.downlinkBandwidthBits = value
}
/**
* @param value Delay time for all packets to destination in milliseconds as an integer from 0 to 2000.
*/
@JvmName("rbyheydalvoveknp")
public suspend fun downlinkDelayMs(`value`: Output) {
this.downlinkDelayMs = value
}
/**
* @param value Time variation in the delay of received packets in milliseconds as an integer from 0 to 2000.
*/
@JvmName("rwakppyvbmquespw")
public suspend fun downlinkJitterMs(`value`: Output) {
this.downlinkJitterMs = value
}
/**
* @param value Proportion of received packets that fail to arrive from 0 to 100 percent.
*/
@JvmName("bxwaumureunthtbg")
public suspend fun downlinkLossPercent(`value`: Output) {
this.downlinkLossPercent = value
}
/**
* @param value The name of the network profile.
*/
@JvmName("gtpcbhqtvussqvwv")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The Amazon Resource Name (ARN) of the specified project.
*/
@JvmName("tfooxpxiadtemyuj")
public suspend fun projectArn(`value`: Output) {
this.projectArn = value
}
/**
* @param value An array of key-value pairs to apply to this resource.
* For more information, see [Tag](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-resource-tags.html) in the *guide* .
*/
@JvmName("fmsvjrllyjmyopvx")
public suspend fun tags(`value`: Output>) {
this.tags = value
}
@JvmName("dbxqxkdjwdnongmd")
public suspend fun tags(vararg values: Output) {
this.tags = Output.all(values.asList())
}
/**
* @param values An array of key-value pairs to apply to this resource.
* For more information, see [Tag](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-resource-tags.html) in the *guide* .
*/
@JvmName("twtfsywrvfylabbn")
public suspend fun tags(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy