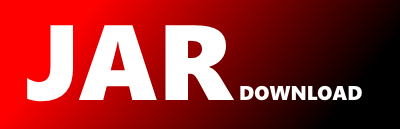
com.pulumi.awsnative.devopsguru.kotlin.DevopsguruFunctions.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.devopsguru.kotlin
import com.pulumi.awsnative.devopsguru.DevopsguruFunctions.getLogAnomalyDetectionIntegrationPlain
import com.pulumi.awsnative.devopsguru.DevopsguruFunctions.getNotificationChannelPlain
import com.pulumi.awsnative.devopsguru.DevopsguruFunctions.getResourceCollectionPlain
import com.pulumi.awsnative.devopsguru.kotlin.enums.ResourceCollectionType
import com.pulumi.awsnative.devopsguru.kotlin.inputs.GetLogAnomalyDetectionIntegrationPlainArgs
import com.pulumi.awsnative.devopsguru.kotlin.inputs.GetLogAnomalyDetectionIntegrationPlainArgsBuilder
import com.pulumi.awsnative.devopsguru.kotlin.inputs.GetNotificationChannelPlainArgs
import com.pulumi.awsnative.devopsguru.kotlin.inputs.GetNotificationChannelPlainArgsBuilder
import com.pulumi.awsnative.devopsguru.kotlin.inputs.GetResourceCollectionPlainArgs
import com.pulumi.awsnative.devopsguru.kotlin.inputs.GetResourceCollectionPlainArgsBuilder
import com.pulumi.awsnative.devopsguru.kotlin.outputs.GetLogAnomalyDetectionIntegrationResult
import com.pulumi.awsnative.devopsguru.kotlin.outputs.GetNotificationChannelResult
import com.pulumi.awsnative.devopsguru.kotlin.outputs.GetResourceCollectionResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.devopsguru.kotlin.outputs.GetLogAnomalyDetectionIntegrationResult.Companion.toKotlin as getLogAnomalyDetectionIntegrationResultToKotlin
import com.pulumi.awsnative.devopsguru.kotlin.outputs.GetNotificationChannelResult.Companion.toKotlin as getNotificationChannelResultToKotlin
import com.pulumi.awsnative.devopsguru.kotlin.outputs.GetResourceCollectionResult.Companion.toKotlin as getResourceCollectionResultToKotlin
public object DevopsguruFunctions {
/**
* This resource schema represents the LogAnomalyDetectionIntegration resource in the Amazon DevOps Guru.
* @param argument null
* @return null
*/
public suspend fun getLogAnomalyDetectionIntegration(argument: GetLogAnomalyDetectionIntegrationPlainArgs): GetLogAnomalyDetectionIntegrationResult =
getLogAnomalyDetectionIntegrationResultToKotlin(getLogAnomalyDetectionIntegrationPlain(argument.toJava()).await())
/**
* @see [getLogAnomalyDetectionIntegration].
* @param accountId The account ID associated with the integration of DevOps Guru with CloudWatch log groups for log anomaly detection.
* @return null
*/
public suspend fun getLogAnomalyDetectionIntegration(accountId: String): GetLogAnomalyDetectionIntegrationResult {
val argument = GetLogAnomalyDetectionIntegrationPlainArgs(
accountId = accountId,
)
return getLogAnomalyDetectionIntegrationResultToKotlin(getLogAnomalyDetectionIntegrationPlain(argument.toJava()).await())
}
/**
* @see [getLogAnomalyDetectionIntegration].
* @param argument Builder for [com.pulumi.awsnative.devopsguru.kotlin.inputs.GetLogAnomalyDetectionIntegrationPlainArgs].
* @return null
*/
public suspend fun getLogAnomalyDetectionIntegration(argument: suspend GetLogAnomalyDetectionIntegrationPlainArgsBuilder.() -> Unit): GetLogAnomalyDetectionIntegrationResult {
val builder = GetLogAnomalyDetectionIntegrationPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getLogAnomalyDetectionIntegrationResultToKotlin(getLogAnomalyDetectionIntegrationPlain(builtArgument.toJava()).await())
}
/**
* This resource schema represents the NotificationChannel resource in the Amazon DevOps Guru.
* @param argument null
* @return null
*/
public suspend fun getNotificationChannel(argument: GetNotificationChannelPlainArgs): GetNotificationChannelResult =
getNotificationChannelResultToKotlin(getNotificationChannelPlain(argument.toJava()).await())
/**
* @see [getNotificationChannel].
* @param id The ID of a notification channel.
* @return null
*/
public suspend fun getNotificationChannel(id: String): GetNotificationChannelResult {
val argument = GetNotificationChannelPlainArgs(
id = id,
)
return getNotificationChannelResultToKotlin(getNotificationChannelPlain(argument.toJava()).await())
}
/**
* @see [getNotificationChannel].
* @param argument Builder for [com.pulumi.awsnative.devopsguru.kotlin.inputs.GetNotificationChannelPlainArgs].
* @return null
*/
public suspend fun getNotificationChannel(argument: suspend GetNotificationChannelPlainArgsBuilder.() -> Unit): GetNotificationChannelResult {
val builder = GetNotificationChannelPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getNotificationChannelResultToKotlin(getNotificationChannelPlain(builtArgument.toJava()).await())
}
/**
* This resource schema represents the ResourceCollection resource in the Amazon DevOps Guru.
* @param argument null
* @return null
*/
public suspend fun getResourceCollection(argument: GetResourceCollectionPlainArgs): GetResourceCollectionResult =
getResourceCollectionResultToKotlin(getResourceCollectionPlain(argument.toJava()).await())
/**
* @see [getResourceCollection].
* @param resourceCollectionType The type of ResourceCollection
* @return null
*/
public suspend fun getResourceCollection(resourceCollectionType: ResourceCollectionType): GetResourceCollectionResult {
val argument = GetResourceCollectionPlainArgs(
resourceCollectionType = resourceCollectionType,
)
return getResourceCollectionResultToKotlin(getResourceCollectionPlain(argument.toJava()).await())
}
/**
* @see [getResourceCollection].
* @param argument Builder for [com.pulumi.awsnative.devopsguru.kotlin.inputs.GetResourceCollectionPlainArgs].
* @return null
*/
public suspend fun getResourceCollection(argument: suspend GetResourceCollectionPlainArgsBuilder.() -> Unit): GetResourceCollectionResult {
val builder = GetResourceCollectionPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getResourceCollectionResultToKotlin(getResourceCollectionPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy