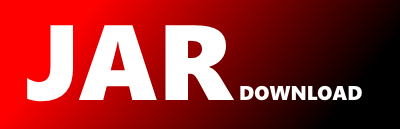
com.pulumi.awsnative.ec2.kotlin.GatewayRouteTableAssociation.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.ec2.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [GatewayRouteTableAssociation].
*/
@PulumiTagMarker
public class GatewayRouteTableAssociationResourceBuilder internal constructor() {
public var name: String? = null
public var args: GatewayRouteTableAssociationArgs = GatewayRouteTableAssociationArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend GatewayRouteTableAssociationArgsBuilder.() -> Unit) {
val builder = GatewayRouteTableAssociationArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): GatewayRouteTableAssociation {
val builtJavaResource =
com.pulumi.awsnative.ec2.GatewayRouteTableAssociation(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return GatewayRouteTableAssociation(builtJavaResource)
}
}
/**
* Associates a gateway with a route table. The gateway and route table must be in the same VPC. This association causes the incoming traffic to the gateway to be routed according to the routes in the route table.
*/
public class GatewayRouteTableAssociation internal constructor(
override val javaResource: com.pulumi.awsnative.ec2.GatewayRouteTableAssociation,
) : KotlinCustomResource(javaResource, GatewayRouteTableAssociationMapper) {
/**
* The route table association ID.
*/
public val associationId: Output
get() = javaResource.associationId().applyValue({ args0 -> args0 })
/**
* The ID of the gateway.
*/
public val gatewayId: Output
get() = javaResource.gatewayId().applyValue({ args0 -> args0 })
/**
* The ID of the route table.
*/
public val routeTableId: Output
get() = javaResource.routeTableId().applyValue({ args0 -> args0 })
}
public object GatewayRouteTableAssociationMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.ec2.GatewayRouteTableAssociation::class == javaResource::class
override fun map(javaResource: Resource): GatewayRouteTableAssociation =
GatewayRouteTableAssociation(
javaResource as
com.pulumi.awsnative.ec2.GatewayRouteTableAssociation,
)
}
/**
* @see [GatewayRouteTableAssociation].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [GatewayRouteTableAssociation].
*/
public suspend fun gatewayRouteTableAssociation(
name: String,
block: suspend GatewayRouteTableAssociationResourceBuilder.() -> Unit,
): GatewayRouteTableAssociation {
val builder = GatewayRouteTableAssociationResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [GatewayRouteTableAssociation].
* @param name The _unique_ name of the resulting resource.
*/
public fun gatewayRouteTableAssociation(name: String): GatewayRouteTableAssociation {
val builder = GatewayRouteTableAssociationResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy