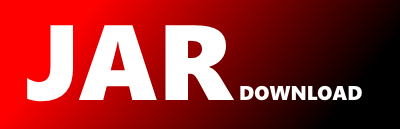
com.pulumi.awsnative.ec2.kotlin.Instance.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.ec2.kotlin
import com.pulumi.awsnative.ec2.kotlin.enums.InstanceAffinity
import com.pulumi.awsnative.ec2.kotlin.outputs.CpuOptionsProperties
import com.pulumi.awsnative.ec2.kotlin.outputs.CreditSpecificationProperties
import com.pulumi.awsnative.ec2.kotlin.outputs.EnclaveOptionsProperties
import com.pulumi.awsnative.ec2.kotlin.outputs.HibernationOptionsProperties
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceBlockDeviceMapping
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceElasticGpuSpecification
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceElasticInferenceAccelerator
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceIpv6Address
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceLaunchTemplateSpecification
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceLicenseSpecification
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceNetworkInterface
import com.pulumi.awsnative.ec2.kotlin.outputs.InstancePrivateDnsNameOptions
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceSsmAssociation
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceState
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceVolume
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.ec2.kotlin.enums.InstanceAffinity.Companion.toKotlin as instanceAffinityToKotlin
import com.pulumi.awsnative.ec2.kotlin.outputs.CpuOptionsProperties.Companion.toKotlin as cpuOptionsPropertiesToKotlin
import com.pulumi.awsnative.ec2.kotlin.outputs.CreditSpecificationProperties.Companion.toKotlin as creditSpecificationPropertiesToKotlin
import com.pulumi.awsnative.ec2.kotlin.outputs.EnclaveOptionsProperties.Companion.toKotlin as enclaveOptionsPropertiesToKotlin
import com.pulumi.awsnative.ec2.kotlin.outputs.HibernationOptionsProperties.Companion.toKotlin as hibernationOptionsPropertiesToKotlin
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceBlockDeviceMapping.Companion.toKotlin as instanceBlockDeviceMappingToKotlin
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceElasticGpuSpecification.Companion.toKotlin as instanceElasticGpuSpecificationToKotlin
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceElasticInferenceAccelerator.Companion.toKotlin as instanceElasticInferenceAcceleratorToKotlin
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceIpv6Address.Companion.toKotlin as instanceIpv6AddressToKotlin
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceLaunchTemplateSpecification.Companion.toKotlin as instanceLaunchTemplateSpecificationToKotlin
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceLicenseSpecification.Companion.toKotlin as instanceLicenseSpecificationToKotlin
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceNetworkInterface.Companion.toKotlin as instanceNetworkInterfaceToKotlin
import com.pulumi.awsnative.ec2.kotlin.outputs.InstancePrivateDnsNameOptions.Companion.toKotlin as instancePrivateDnsNameOptionsToKotlin
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceSsmAssociation.Companion.toKotlin as instanceSsmAssociationToKotlin
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceState.Companion.toKotlin as instanceStateToKotlin
import com.pulumi.awsnative.ec2.kotlin.outputs.InstanceVolume.Companion.toKotlin as instanceVolumeToKotlin
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin as tagToKotlin
/**
* Builder for [Instance].
*/
@PulumiTagMarker
public class InstanceResourceBuilder internal constructor() {
public var name: String? = null
public var args: InstanceArgs = InstanceArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend InstanceArgsBuilder.() -> Unit) {
val builder = InstanceArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Instance {
val builtJavaResource = com.pulumi.awsnative.ec2.Instance(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Instance(builtJavaResource)
}
}
/**
* Resource Type definition for AWS::EC2::Instance
*/
public class Instance internal constructor(
override val javaResource: com.pulumi.awsnative.ec2.Instance,
) : KotlinCustomResource(javaResource, InstanceMapper) {
/**
* This property is reserved for internal use. If you use it, the stack fails with this error: Bad property set: [Testing this property] (Service: AmazonEC2; Status Code: 400; Error Code: InvalidParameterCombination; Request ID: 0XXXXXX-49c7-4b40-8bcc-76885dcXXXXX).
*/
public val additionalInfo: Output?
get() = javaResource.additionalInfo().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Indicates whether the instance is associated with a dedicated host. If you want the instance to always restart on the same host on which it was launched, specify host. If you want the instance to restart on any available host, but try to launch onto the last host it ran on (on a best-effort basis), specify default.
*/
public val affinity: Output?
get() = javaResource.affinity().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
instanceAffinityToKotlin(args0)
})
}).orElse(null)
})
/**
* The Availability Zone of the instance.
*/
public val availabilityZone: Output?
get() = javaResource.availabilityZone().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The block device mapping entries that defines the block devices to attach to the instance at launch.
*/
public val blockDeviceMappings: Output>?
get() = javaResource.blockDeviceMappings().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
instanceBlockDeviceMappingToKotlin(args0)
})
})
}).orElse(null)
})
/**
* The CPU options for the instance.
*/
public val cpuOptions: Output?
get() = javaResource.cpuOptions().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
cpuOptionsPropertiesToKotlin(args0)
})
}).orElse(null)
})
/**
* The credit option for CPU usage of the burstable performance instance. Valid values are standard and unlimited.
*/
public val creditSpecification: Output?
get() = javaResource.creditSpecification().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> creditSpecificationPropertiesToKotlin(args0) })
}).orElse(null)
})
/**
* If you set this parameter to true, you can't terminate the instance using the Amazon EC2 console, CLI, or API; otherwise, you can.
*/
public val disableApiTermination: Output?
get() = javaResource.disableApiTermination().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Indicates whether the instance is optimized for Amazon EBS I/O.
*/
public val ebsOptimized: Output?
get() = javaResource.ebsOptimized().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* An elastic GPU to associate with the instance.
*/
public val elasticGpuSpecifications: Output>?
get() = javaResource.elasticGpuSpecifications().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
instanceElasticGpuSpecificationToKotlin(args0)
})
})
}).orElse(null)
})
/**
* An elastic inference accelerator to associate with the instance.
*/
public val elasticInferenceAccelerators: Output>?
get() = javaResource.elasticInferenceAccelerators().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
instanceElasticInferenceAcceleratorToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Indicates whether the instance is enabled for AWS Nitro Enclaves.
*/
public val enclaveOptions: Output?
get() = javaResource.enclaveOptions().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> enclaveOptionsPropertiesToKotlin(args0) })
}).orElse(null)
})
/**
* Indicates whether an instance is enabled for hibernation.
*/
public val hibernationOptions: Output?
get() = javaResource.hibernationOptions().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> hibernationOptionsPropertiesToKotlin(args0) })
}).orElse(null)
})
/**
* If you specify host for the Affinity property, the ID of a dedicated host that the instance is associated with. If you don't specify an ID, Amazon EC2 launches the instance onto any available, compatible dedicated host in your account.
*/
public val hostId: Output?
get() = javaResource.hostId().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The ARN of the host resource group in which to launch the instances. If you specify a host resource group ARN, omit the Tenancy parameter or set it to host.
*/
public val hostResourceGroupArn: Output?
get() = javaResource.hostResourceGroupArn().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The IAM instance profile.
*/
public val iamInstanceProfile: Output?
get() = javaResource.iamInstanceProfile().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The ID of the AMI. An AMI ID is required to launch an instance and must be specified here or in a launch template.
*/
public val imageId: Output?
get() = javaResource.imageId().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The EC2 Instance ID.
*/
public val instanceId: Output
get() = javaResource.instanceId().applyValue({ args0 -> args0 })
/**
* Indicates whether an instance stops or terminates when you initiate shutdown from the instance (using the operating system command for system shutdown).
*/
public val instanceInitiatedShutdownBehavior: Output?
get() = javaResource.instanceInitiatedShutdownBehavior().applyValue({ args0 ->
args0.map({ args0 -> args0 }).orElse(null)
})
/**
* The instance type.
*/
public val instanceType: Output?
get() = javaResource.instanceType().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* [EC2-VPC] The number of IPv6 addresses to associate with the primary network interface. Amazon EC2 chooses the IPv6 addresses from the range of your subnet.
*/
public val ipv6AddressCount: Output?
get() = javaResource.ipv6AddressCount().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* [EC2-VPC] The IPv6 addresses from the range of the subnet to associate with the primary network interface.
*/
public val ipv6Addresses: Output>?
get() = javaResource.ipv6Addresses().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
instanceIpv6AddressToKotlin(args0)
})
})
}).orElse(null)
})
/**
* The ID of the kernel.
*/
public val kernelId: Output?
get() = javaResource.kernelId().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The name of the key pair.
*/
public val keyName: Output?
get() = javaResource.keyName().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The launch template to use to launch the instances.
*/
public val launchTemplate: Output?
get() = javaResource.launchTemplate().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> instanceLaunchTemplateSpecificationToKotlin(args0) })
}).orElse(null)
})
/**
* The license configurations.
*/
public val licenseSpecifications: Output>?
get() = javaResource.licenseSpecifications().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
instanceLicenseSpecificationToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Specifies whether detailed monitoring is enabled for the instance.
*/
public val monitoring: Output?
get() = javaResource.monitoring().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The network interfaces to associate with the instance.
*/
public val networkInterfaces: Output>?
get() = javaResource.networkInterfaces().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
instanceNetworkInterfaceToKotlin(args0)
})
})
}).orElse(null)
})
/**
* The name of an existing placement group that you want to launch the instance into (cluster | partition | spread).
*/
public val placementGroupName: Output?
get() = javaResource.placementGroupName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The private DNS name of the specified instance. For example: ip-10-24-34-0.ec2.internal.
*/
public val privateDnsName: Output
get() = javaResource.privateDnsName().applyValue({ args0 -> args0 })
/**
* The options for the instance hostname.
*/
public val privateDnsNameOptions: Output?
get() = javaResource.privateDnsNameOptions().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> instancePrivateDnsNameOptionsToKotlin(args0) })
}).orElse(null)
})
/**
* The private IP address of the specified instance. For example: 10.24.34.0.
*/
public val privateIp: Output
get() = javaResource.privateIp().applyValue({ args0 -> args0 })
/**
* [EC2-VPC] The primary IPv4 address. You must specify a value from the IPv4 address range of the subnet.
*/
public val privateIpAddress: Output?
get() = javaResource.privateIpAddress().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Indicates whether to assign the tags from the instance to all of the volumes attached to the instance at launch. If you specify true and you assign tags to the instance, those tags are automatically assigned to all of the volumes that you attach to the instance at launch. If you specify false, those tags are not assigned to the attached volumes.
*/
public val propagateTagsToVolumeOnCreation: Output?
get() = javaResource.propagateTagsToVolumeOnCreation().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The public DNS name of the specified instance. For example: ec2-107-20-50-45.compute-1.amazonaws.com.
*/
public val publicDnsName: Output
get() = javaResource.publicDnsName().applyValue({ args0 -> args0 })
/**
* The public IP address of the specified instance. For example: 192.0.2.0.
*/
public val publicIp: Output
get() = javaResource.publicIp().applyValue({ args0 -> args0 })
/**
* The ID of the RAM disk to select.
*/
public val ramdiskId: Output?
get() = javaResource.ramdiskId().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The IDs of the security groups.
*/
public val securityGroupIds: Output>?
get() = javaResource.securityGroupIds().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* the names of the security groups. For a nondefault VPC, you must use security group IDs instead.
*/
public val securityGroups: Output>?
get() = javaResource.securityGroups().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* Specifies whether to enable an instance launched in a VPC to perform NAT.
*/
public val sourceDestCheck: Output?
get() = javaResource.sourceDestCheck().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The SSM document and parameter values in AWS Systems Manager to associate with this instance.
*/
public val ssmAssociations: Output>?
get() = javaResource.ssmAssociations().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
instanceSsmAssociationToKotlin(args0)
})
})
}).orElse(null)
})
/**
* The current state of the instance.
*/
public val state: Output
get() = javaResource.state().applyValue({ args0 ->
args0.let({ args0 ->
instanceStateToKotlin(args0)
})
})
/**
* [EC2-VPC] The ID of the subnet to launch the instance into.
*/
public val subnetId: Output?
get() = javaResource.subnetId().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The tags to add to the instance.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> tagToKotlin(args0) })
})
}).orElse(null)
})
/**
* The tenancy of the instance (if the instance is running in a VPC). An instance with a tenancy of dedicated runs on single-tenant hardware.
*/
public val tenancy: Output?
get() = javaResource.tenancy().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The user data to make available to the instance.
*/
public val userData: Output?
get() = javaResource.userData().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The volumes to attach to the instance.
*/
public val volumes: Output>?
get() = javaResource.volumes().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> instanceVolumeToKotlin(args0) })
})
}).orElse(null)
})
/**
* The ID of the VPC that the instance is running in.
*/
public val vpcId: Output
get() = javaResource.vpcId().applyValue({ args0 -> args0 })
}
public object InstanceMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.ec2.Instance::class == javaResource::class
override fun map(javaResource: Resource): Instance = Instance(
javaResource as
com.pulumi.awsnative.ec2.Instance,
)
}
/**
* @see [Instance].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Instance].
*/
public suspend fun instance(name: String, block: suspend InstanceResourceBuilder.() -> Unit): Instance {
val builder = InstanceResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Instance].
* @param name The _unique_ name of the resulting resource.
*/
public fun instance(name: String): Instance {
val builder = InstanceResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy