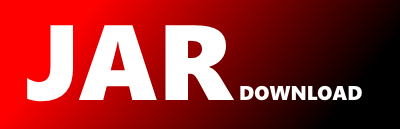
com.pulumi.awsnative.ec2.kotlin.LaunchTemplateArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.ec2.kotlin
import com.pulumi.awsnative.ec2.LaunchTemplateArgs.builder
import com.pulumi.awsnative.ec2.kotlin.inputs.LaunchTemplateDataArgs
import com.pulumi.awsnative.ec2.kotlin.inputs.LaunchTemplateDataArgsBuilder
import com.pulumi.awsnative.ec2.kotlin.inputs.LaunchTemplateTagSpecificationArgs
import com.pulumi.awsnative.ec2.kotlin.inputs.LaunchTemplateTagSpecificationArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Specifies the properties for creating a launch template.
* The minimum required properties for specifying a launch template are as follows:
* + You must specify at least one property for the launch template data.
* + You can optionally specify a name for the launch template. If you do not specify a name, CFN creates a name for you.
* A launch template can contain some or all of the configuration information to launch an instance. When you launch an instance using a launch template, instance properties that are not specified in the launch template use default values, except the ``ImageId`` property, which has no default value. If you do not specify an AMI ID for the launch template ``ImageId`` property, you must specify an AMI ID for the instance ``ImageId`` property.
* For more information, see [Launch an instance from a launch template](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/ec2-launch-templates.html) in the *Amazon EC2 User Guide*.
* @property launchTemplateData The information for the launch template.
* @property launchTemplateName A name for the launch template.
* @property tagSpecifications The tags to apply to the launch template on creation. To tag the launch template, the resource type must be ``launch-template``.
* To specify the tags for the resources that are created when an instance is launched, you must use [TagSpecifications](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ec2-launchtemplate-launchtemplatedata.html#cfn-ec2-launchtemplate-launchtemplatedata-tagspecifications).
* @property versionDescription A description for the first version of the launch template.
*/
public data class LaunchTemplateArgs(
public val launchTemplateData: Output? = null,
public val launchTemplateName: Output? = null,
public val tagSpecifications: Output>? = null,
public val versionDescription: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.ec2.LaunchTemplateArgs =
com.pulumi.awsnative.ec2.LaunchTemplateArgs.builder()
.launchTemplateData(
launchTemplateData?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.launchTemplateName(launchTemplateName?.applyValue({ args0 -> args0 }))
.tagSpecifications(
tagSpecifications?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.versionDescription(versionDescription?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LaunchTemplateArgs].
*/
@PulumiTagMarker
public class LaunchTemplateArgsBuilder internal constructor() {
private var launchTemplateData: Output? = null
private var launchTemplateName: Output? = null
private var tagSpecifications: Output>? = null
private var versionDescription: Output? = null
/**
* @param value The information for the launch template.
*/
@JvmName("haupcjplvlqqgrst")
public suspend fun launchTemplateData(`value`: Output) {
this.launchTemplateData = value
}
/**
* @param value A name for the launch template.
*/
@JvmName("jjasvvbbsjfawnqc")
public suspend fun launchTemplateName(`value`: Output) {
this.launchTemplateName = value
}
/**
* @param value The tags to apply to the launch template on creation. To tag the launch template, the resource type must be ``launch-template``.
* To specify the tags for the resources that are created when an instance is launched, you must use [TagSpecifications](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ec2-launchtemplate-launchtemplatedata.html#cfn-ec2-launchtemplate-launchtemplatedata-tagspecifications).
*/
@JvmName("qqcjossjirggtctc")
public suspend fun tagSpecifications(`value`: Output>) {
this.tagSpecifications = value
}
@JvmName("jylmwciddogkdxdn")
public suspend fun tagSpecifications(vararg values: Output) {
this.tagSpecifications = Output.all(values.asList())
}
/**
* @param values The tags to apply to the launch template on creation. To tag the launch template, the resource type must be ``launch-template``.
* To specify the tags for the resources that are created when an instance is launched, you must use [TagSpecifications](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ec2-launchtemplate-launchtemplatedata.html#cfn-ec2-launchtemplate-launchtemplatedata-tagspecifications).
*/
@JvmName("ligetwwckyxhfwgq")
public suspend fun tagSpecifications(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy