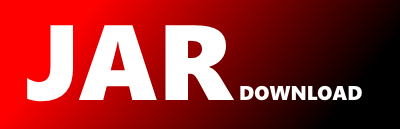
com.pulumi.awsnative.ec2.kotlin.SecurityGroupEgressArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.ec2.kotlin
import com.pulumi.awsnative.ec2.SecurityGroupEgressArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Adds the specified outbound (egress) rule to a security group.
* An outbound rule permits instances to send traffic to the specified IPv4 or IPv6 address range, the IP addresses that are specified by a prefix list, or the instances that are associated with a destination security group. For more information, see [Security group rules](https://docs.aws.amazon.com/vpc/latest/userguide/security-group-rules.html).
* You must specify exactly one of the following destinations: an IPv4 address range, an IPv6 address range, a prefix list, or a security group.
* You must specify a protocol for each rule (for example, TCP). If the protocol is TCP or UDP, you must also specify a port or port range. If the protocol is ICMP or ICMPv6, you must also specify the ICMP/ICMPv6 type and code. To specify all types or all codes, use -1.
* Rule changes are propagated to instances associated with the security group as quickly as possible. However, a small delay might occur.
* @property cidrIp The IPv4 address range, in CIDR format.
* You must specify exactly one of the following: ``CidrIp``, ``CidrIpv6``, ``DestinationPrefixListId``, or ``DestinationSecurityGroupId``.
* For examples of rules that you can add to security groups for specific access scenarios, see [Security group rules for different use cases](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/security-group-rules-reference.html) in the *User Guide*.
* @property cidrIpv6 The IPv6 address range, in CIDR format.
* You must specify exactly one of the following: ``CidrIp``, ``CidrIpv6``, ``DestinationPrefixListId``, or ``DestinationSecurityGroupId``.
* For examples of rules that you can add to security groups for specific access scenarios, see [Security group rules for different use cases](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/security-group-rules-reference.html) in the *User Guide*.
* @property description The description of an egress (outbound) security group rule.
* Constraints: Up to 255 characters in length. Allowed characters are a-z, A-Z, 0-9, spaces, and ._-:/()#,@[]+=;{}!$*
* @property destinationPrefixListId The prefix list IDs for an AWS service. This is the AWS service to access through a VPC endpoint from instances associated with the security group.
* You must specify exactly one of the following: ``CidrIp``, ``CidrIpv6``, ``DestinationPrefixListId``, or ``DestinationSecurityGroupId``.
* @property destinationSecurityGroupId The ID of the security group.
* You must specify exactly one of the following: ``CidrIp``, ``CidrIpv6``, ``DestinationPrefixListId``, or ``DestinationSecurityGroupId``.
* @property fromPort If the protocol is TCP or UDP, this is the start of the port range. If the protocol is ICMP or ICMPv6, this is the ICMP type or -1 (all ICMP types).
* @property groupId The ID of the security group. You must specify either the security group ID or the security group name in the request. For security groups in a nondefault VPC, you must specify the security group ID.
* @property ipProtocol The IP protocol name (``tcp``, ``udp``, ``icmp``, ``icmpv6``) or number (see [Protocol Numbers](https://docs.aws.amazon.com/http://www.iana.org/assignments/protocol-numbers/protocol-numbers.xhtml)).
* Use ``-1`` to specify all protocols. When authorizing security group rules, specifying ``-1`` or a protocol number other than ``tcp``, ``udp``, ``icmp``, or ``icmpv6`` allows traffic on all ports, regardless of any port range you specify. For ``tcp``, ``udp``, and ``icmp``, you must specify a port range. For ``icmpv6``, the port range is optional; if you omit the port range, traffic for all types and codes is allowed.
* @property toPort If the protocol is TCP or UDP, this is the end of the port range. If the protocol is ICMP or ICMPv6, this is the ICMP code or -1 (all ICMP codes). If the start port is -1 (all ICMP types), then the end port must be -1 (all ICMP codes).
*/
public data class SecurityGroupEgressArgs(
public val cidrIp: Output? = null,
public val cidrIpv6: Output? = null,
public val description: Output? = null,
public val destinationPrefixListId: Output? = null,
public val destinationSecurityGroupId: Output? = null,
public val fromPort: Output? = null,
public val groupId: Output? = null,
public val ipProtocol: Output? = null,
public val toPort: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.ec2.SecurityGroupEgressArgs =
com.pulumi.awsnative.ec2.SecurityGroupEgressArgs.builder()
.cidrIp(cidrIp?.applyValue({ args0 -> args0 }))
.cidrIpv6(cidrIpv6?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.destinationPrefixListId(destinationPrefixListId?.applyValue({ args0 -> args0 }))
.destinationSecurityGroupId(destinationSecurityGroupId?.applyValue({ args0 -> args0 }))
.fromPort(fromPort?.applyValue({ args0 -> args0 }))
.groupId(groupId?.applyValue({ args0 -> args0 }))
.ipProtocol(ipProtocol?.applyValue({ args0 -> args0 }))
.toPort(toPort?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SecurityGroupEgressArgs].
*/
@PulumiTagMarker
public class SecurityGroupEgressArgsBuilder internal constructor() {
private var cidrIp: Output? = null
private var cidrIpv6: Output? = null
private var description: Output? = null
private var destinationPrefixListId: Output? = null
private var destinationSecurityGroupId: Output? = null
private var fromPort: Output? = null
private var groupId: Output? = null
private var ipProtocol: Output? = null
private var toPort: Output? = null
/**
* @param value The IPv4 address range, in CIDR format.
* You must specify exactly one of the following: ``CidrIp``, ``CidrIpv6``, ``DestinationPrefixListId``, or ``DestinationSecurityGroupId``.
* For examples of rules that you can add to security groups for specific access scenarios, see [Security group rules for different use cases](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/security-group-rules-reference.html) in the *User Guide*.
*/
@JvmName("braviysxghdamlam")
public suspend fun cidrIp(`value`: Output) {
this.cidrIp = value
}
/**
* @param value The IPv6 address range, in CIDR format.
* You must specify exactly one of the following: ``CidrIp``, ``CidrIpv6``, ``DestinationPrefixListId``, or ``DestinationSecurityGroupId``.
* For examples of rules that you can add to security groups for specific access scenarios, see [Security group rules for different use cases](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/security-group-rules-reference.html) in the *User Guide*.
*/
@JvmName("wpqnmuaspwsulqfg")
public suspend fun cidrIpv6(`value`: Output) {
this.cidrIpv6 = value
}
/**
* @param value The description of an egress (outbound) security group rule.
* Constraints: Up to 255 characters in length. Allowed characters are a-z, A-Z, 0-9, spaces, and ._-:/()#,@[]+=;{}!$*
*/
@JvmName("wrrfapjcrplnngxp")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The prefix list IDs for an AWS service. This is the AWS service to access through a VPC endpoint from instances associated with the security group.
* You must specify exactly one of the following: ``CidrIp``, ``CidrIpv6``, ``DestinationPrefixListId``, or ``DestinationSecurityGroupId``.
*/
@JvmName("fhiowgcmtuknhwso")
public suspend fun destinationPrefixListId(`value`: Output) {
this.destinationPrefixListId = value
}
/**
* @param value The ID of the security group.
* You must specify exactly one of the following: ``CidrIp``, ``CidrIpv6``, ``DestinationPrefixListId``, or ``DestinationSecurityGroupId``.
*/
@JvmName("kxxehotsyhimvusx")
public suspend fun destinationSecurityGroupId(`value`: Output) {
this.destinationSecurityGroupId = value
}
/**
* @param value If the protocol is TCP or UDP, this is the start of the port range. If the protocol is ICMP or ICMPv6, this is the ICMP type or -1 (all ICMP types).
*/
@JvmName("dyeqvqqkwmbxrhvn")
public suspend fun fromPort(`value`: Output) {
this.fromPort = value
}
/**
* @param value The ID of the security group. You must specify either the security group ID or the security group name in the request. For security groups in a nondefault VPC, you must specify the security group ID.
*/
@JvmName("auxwysslbwabuthe")
public suspend fun groupId(`value`: Output) {
this.groupId = value
}
/**
* @param value The IP protocol name (``tcp``, ``udp``, ``icmp``, ``icmpv6``) or number (see [Protocol Numbers](https://docs.aws.amazon.com/http://www.iana.org/assignments/protocol-numbers/protocol-numbers.xhtml)).
* Use ``-1`` to specify all protocols. When authorizing security group rules, specifying ``-1`` or a protocol number other than ``tcp``, ``udp``, ``icmp``, or ``icmpv6`` allows traffic on all ports, regardless of any port range you specify. For ``tcp``, ``udp``, and ``icmp``, you must specify a port range. For ``icmpv6``, the port range is optional; if you omit the port range, traffic for all types and codes is allowed.
*/
@JvmName("begwiqpwheipotwe")
public suspend fun ipProtocol(`value`: Output) {
this.ipProtocol = value
}
/**
* @param value If the protocol is TCP or UDP, this is the end of the port range. If the protocol is ICMP or ICMPv6, this is the ICMP code or -1 (all ICMP codes). If the start port is -1 (all ICMP types), then the end port must be -1 (all ICMP codes).
*/
@JvmName("nyyqxvvbieegsjnk")
public suspend fun toPort(`value`: Output) {
this.toPort = value
}
/**
* @param value The IPv4 address range, in CIDR format.
* You must specify exactly one of the following: ``CidrIp``, ``CidrIpv6``, ``DestinationPrefixListId``, or ``DestinationSecurityGroupId``.
* For examples of rules that you can add to security groups for specific access scenarios, see [Security group rules for different use cases](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/security-group-rules-reference.html) in the *User Guide*.
*/
@JvmName("jwillxwqpuqebpay")
public suspend fun cidrIp(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cidrIp = mapped
}
/**
* @param value The IPv6 address range, in CIDR format.
* You must specify exactly one of the following: ``CidrIp``, ``CidrIpv6``, ``DestinationPrefixListId``, or ``DestinationSecurityGroupId``.
* For examples of rules that you can add to security groups for specific access scenarios, see [Security group rules for different use cases](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/security-group-rules-reference.html) in the *User Guide*.
*/
@JvmName("modvcakedehjrmin")
public suspend fun cidrIpv6(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cidrIpv6 = mapped
}
/**
* @param value The description of an egress (outbound) security group rule.
* Constraints: Up to 255 characters in length. Allowed characters are a-z, A-Z, 0-9, spaces, and ._-:/()#,@[]+=;{}!$*
*/
@JvmName("iqfrgatwdlkouxvm")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value The prefix list IDs for an AWS service. This is the AWS service to access through a VPC endpoint from instances associated with the security group.
* You must specify exactly one of the following: ``CidrIp``, ``CidrIpv6``, ``DestinationPrefixListId``, or ``DestinationSecurityGroupId``.
*/
@JvmName("ljnltkgfksqyegvd")
public suspend fun destinationPrefixListId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.destinationPrefixListId = mapped
}
/**
* @param value The ID of the security group.
* You must specify exactly one of the following: ``CidrIp``, ``CidrIpv6``, ``DestinationPrefixListId``, or ``DestinationSecurityGroupId``.
*/
@JvmName("rduwmpyxaqkrefht")
public suspend fun destinationSecurityGroupId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.destinationSecurityGroupId = mapped
}
/**
* @param value If the protocol is TCP or UDP, this is the start of the port range. If the protocol is ICMP or ICMPv6, this is the ICMP type or -1 (all ICMP types).
*/
@JvmName("jquxuamrvjssline")
public suspend fun fromPort(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fromPort = mapped
}
/**
* @param value The ID of the security group. You must specify either the security group ID or the security group name in the request. For security groups in a nondefault VPC, you must specify the security group ID.
*/
@JvmName("fkwjbwkndofuwaxk")
public suspend fun groupId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.groupId = mapped
}
/**
* @param value The IP protocol name (``tcp``, ``udp``, ``icmp``, ``icmpv6``) or number (see [Protocol Numbers](https://docs.aws.amazon.com/http://www.iana.org/assignments/protocol-numbers/protocol-numbers.xhtml)).
* Use ``-1`` to specify all protocols. When authorizing security group rules, specifying ``-1`` or a protocol number other than ``tcp``, ``udp``, ``icmp``, or ``icmpv6`` allows traffic on all ports, regardless of any port range you specify. For ``tcp``, ``udp``, and ``icmp``, you must specify a port range. For ``icmpv6``, the port range is optional; if you omit the port range, traffic for all types and codes is allowed.
*/
@JvmName("euidsbxvmejdkocd")
public suspend fun ipProtocol(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ipProtocol = mapped
}
/**
* @param value If the protocol is TCP or UDP, this is the end of the port range. If the protocol is ICMP or ICMPv6, this is the ICMP code or -1 (all ICMP codes). If the start port is -1 (all ICMP types), then the end port must be -1 (all ICMP codes).
*/
@JvmName("xierlvmammmjmnow")
public suspend fun toPort(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.toPort = mapped
}
internal fun build(): SecurityGroupEgressArgs = SecurityGroupEgressArgs(
cidrIp = cidrIp,
cidrIpv6 = cidrIpv6,
description = description,
destinationPrefixListId = destinationPrefixListId,
destinationSecurityGroupId = destinationSecurityGroupId,
fromPort = fromPort,
groupId = groupId,
ipProtocol = ipProtocol,
toPort = toPort,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy