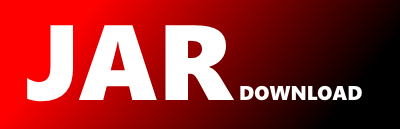
com.pulumi.awsnative.ec2.kotlin.Volume.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.ec2.kotlin
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [Volume].
*/
@PulumiTagMarker
public class VolumeResourceBuilder internal constructor() {
public var name: String? = null
public var args: VolumeArgs = VolumeArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend VolumeArgsBuilder.() -> Unit) {
val builder = VolumeArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Volume {
val builtJavaResource = com.pulumi.awsnative.ec2.Volume(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Volume(builtJavaResource)
}
}
/**
* Specifies an Amazon Elastic Block Store (Amazon EBS) volume.
* When you use AWS CloudFormation to update an Amazon EBS volume that modifies `Iops` , `Size` , or `VolumeType` , there is a cooldown period before another operation can occur. This can cause your stack to report being in `UPDATE_IN_PROGRESS` or `UPDATE_ROLLBACK_IN_PROGRESS` for long periods of time.
* Amazon EBS does not support sizing down an Amazon EBS volume. AWS CloudFormation does not attempt to modify an Amazon EBS volume to a smaller size on rollback.
* Some common scenarios when you might encounter a cooldown period for Amazon EBS include:
* - You successfully update an Amazon EBS volume and the update succeeds. When you attempt another update within the cooldown window, that update will be subject to a cooldown period.
* - You successfully update an Amazon EBS volume and the update succeeds but another change in your `update-stack` call fails. The rollback will be subject to a cooldown period.
* For more information, see [Requirements for EBS volume modifications](https://docs.aws.amazon.com/ebs/latest/userguide/modify-volume-requirements.html) .
* *DeletionPolicy attribute*
* To control how AWS CloudFormation handles the volume when the stack is deleted, set a deletion policy for your volume. You can choose to retain the volume, to delete the volume, or to create a snapshot of the volume. For more information, see [DeletionPolicy attribute](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-attribute-deletionpolicy.html) .
* > If you set a deletion policy that creates a snapshot, all tags on the volume are included in the snapshot.
*/
public class Volume internal constructor(
override val javaResource: com.pulumi.awsnative.ec2.Volume,
) : KotlinCustomResource(javaResource, VolumeMapper) {
/**
* Indicates whether the volume is auto-enabled for I/O operations. By default, Amazon EBS disables I/O to the volume from attached EC2 instances when it determines that a volume's data is potentially inconsistent. If the consistency of the volume is not a concern, and you prefer that the volume be made available immediately if it's impaired, you can configure the volume to automatically enable I/O.
*/
public val autoEnableIo: Output?
get() = javaResource.autoEnableIo().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The ID of the Availability Zone in which to create the volume. For example, ``us-east-1a``.
*/
public val availabilityZone: Output
get() = javaResource.availabilityZone().applyValue({ args0 -> args0 })
/**
* Indicates whether the volume should be encrypted. The effect of setting the encryption state to ``true`` depends on the volume origin (new or from a snapshot), starting encryption state, ownership, and whether encryption by default is enabled. For more information, see [Encryption by default](https://docs.aws.amazon.com/ebs/latest/userguide/work-with-ebs-encr.html#encryption-by-default) in the *Amazon EBS User Guide*.
* Encrypted Amazon EBS volumes must be attached to instances that support Amazon EBS encryption. For more information, see [Supported instance types](https://docs.aws.amazon.com/ebs/latest/userguide/ebs-encryption-requirements.html#ebs-encryption_supported_instances).
*/
public val encrypted: Output?
get() = javaResource.encrypted().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The number of I/O operations per second (IOPS). For ``gp3``, ``io1``, and ``io2`` volumes, this represents the number of IOPS that are provisioned for the volume. For ``gp2`` volumes, this represents the baseline performance of the volume and the rate at which the volume accumulates I/O credits for bursting.
* The following are the supported values for each volume type:
* + ``gp3``: 3,000 - 16,000 IOPS
* + ``io1``: 100 - 64,000 IOPS
* + ``io2``: 100 - 256,000 IOPS
* For ``io2`` volumes, you can achieve up to 256,000 IOPS on [instances built on the Nitro System](https://docs.aws.amazon.com/ec2/latest/instancetypes/ec2-nitro-instances.html). On other instances, you can achieve performance up to 32,000 IOPS.
* This parameter is required for ``io1`` and ``io2`` volumes. The default for ``gp3`` volumes is 3,000 IOPS. This parameter is not supported for ``gp2``, ``st1``, ``sc1``, or ``standard`` volumes.
*/
public val iops: Output?
get() = javaResource.iops().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The identifier of the kms-key-long to use for Amazon EBS encryption. If ``KmsKeyId`` is specified, the encrypted state must be ``true``.
* If you omit this property and your account is enabled for encryption by default, or *Encrypted* is set to ``true``, then the volume is encrypted using the default key specified for your account. If your account does not have a default key, then the volume is encrypted using the aws-managed-key.
* Alternatively, if you want to specify a different key, you can specify one of the following:
* + Key ID. For example, 1234abcd-12ab-34cd-56ef-1234567890ab.
* + Key alias. Specify the alias for the key, prefixed with ``alias/``. For example, for a key with the alias ``my_cmk``, use ``alias/my_cmk``. Or to specify the aws-managed-key, use ``alias/aws/ebs``.
* + Key ARN. For example, arn:aws:kms:us-east-1:012345678910:key/1234abcd-12ab-34cd-56ef-1234567890ab.
* + Alias ARN. For example, arn:aws:kms:us-east-1:012345678910:alias/ExampleAlias.
*/
public val kmsKeyId: Output?
get() = javaResource.kmsKeyId().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Indicates whether Amazon EBS Multi-Attach is enabled.
* CFNlong does not currently support updating a single-attach volume to be multi-attach enabled, updating a multi-attach enabled volume to be single-attach, or updating the size or number of I/O operations per second (IOPS) of a multi-attach enabled volume.
*/
public val multiAttachEnabled: Output?
get() = javaResource.multiAttachEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The Amazon Resource Name (ARN) of the Outpost.
*/
public val outpostArn: Output?
get() = javaResource.outpostArn().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The size of the volume, in GiBs. You must specify either a snapshot ID or a volume size. If you specify a snapshot, the default is the snapshot size. You can specify a volume size that is equal to or larger than the snapshot size.
* The following are the supported volumes sizes for each volume type:
* + ``gp2`` and ``gp3``: 1 - 16,384 GiB
* + ``io1``: 4 - 16,384 GiB
* + ``io2``: 4 - 65,536 GiB
* + ``st1`` and ``sc1``: 125 - 16,384 GiB
* + ``standard``: 1 - 1024 GiB
*/
public val size: Output?
get() = javaResource.size().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The snapshot from which to create the volume. You must specify either a snapshot ID or a volume size.
*/
public val snapshotId: Output?
get() = javaResource.snapshotId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The tags to apply to the volume during creation.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> toKotlin(args0) })
})
}).orElse(null)
})
/**
* The throughput to provision for a volume, with a maximum of 1,000 MiB/s.
* This parameter is valid only for ``gp3`` volumes. The default value is 125.
* Valid Range: Minimum value of 125. Maximum value of 1000.
*/
public val throughput: Output?
get() = javaResource.throughput().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The ID of the volume.
*/
public val volumeId: Output
get() = javaResource.volumeId().applyValue({ args0 -> args0 })
/**
* The volume type. This parameter can be one of the following values:
* + General Purpose SSD: ``gp2`` | ``gp3``
* + Provisioned IOPS SSD: ``io1`` | ``io2``
* + Throughput Optimized HDD: ``st1``
* + Cold HDD: ``sc1``
* + Magnetic: ``standard``
* For more information, see [Amazon EBS volume types](https://docs.aws.amazon.com/ebs/latest/userguide/ebs-volume-types.html).
* Default: ``gp2``
*/
public val volumeType: Output?
get() = javaResource.volumeType().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
}
public object VolumeMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.ec2.Volume::class == javaResource::class
override fun map(javaResource: Resource): Volume = Volume(
javaResource as
com.pulumi.awsnative.ec2.Volume,
)
}
/**
* @see [Volume].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Volume].
*/
public suspend fun volume(name: String, block: suspend VolumeResourceBuilder.() -> Unit): Volume {
val builder = VolumeResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Volume].
* @param name The _unique_ name of the resulting resource.
*/
public fun volume(name: String): Volume {
val builder = VolumeResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy