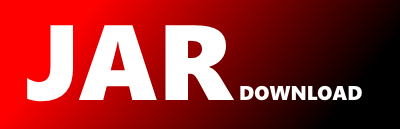
com.pulumi.awsnative.ec2.kotlin.inputs.Ec2FleetPlacementArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.ec2.kotlin.inputs
import com.pulumi.awsnative.ec2.inputs.Ec2FleetPlacementArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property affinity The affinity setting for the instance on the Dedicated Host.
* This parameter is not supported for [CreateFleet](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateFleet) or [ImportInstance](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_ImportInstance.html) .
* @property availabilityZone The Availability Zone of the instance.
* If not specified, an Availability Zone will be automatically chosen for you based on the load balancing criteria for the Region.
* This parameter is not supported for [CreateFleet](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateFleet) .
* @property groupName The name of the placement group that the instance is in. If you specify `GroupName` , you can't specify `GroupId` .
* @property hostId The ID of the Dedicated Host on which the instance resides.
* This parameter is not supported for [CreateFleet](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateFleet) or [ImportInstance](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_ImportInstance.html) .
* @property hostResourceGroupArn The ARN of the host resource group in which to launch the instances.
* If you specify this parameter, either omit the *Tenancy* parameter or set it to `host` .
* This parameter is not supported for [CreateFleet](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateFleet) .
* @property partitionNumber The number of the partition that the instance is in. Valid only if the placement group strategy is set to `partition` .
* This parameter is not supported for [CreateFleet](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateFleet) .
* @property spreadDomain Reserved for future use.
* @property tenancy The tenancy of the instance. An instance with a tenancy of `dedicated` runs on single-tenant hardware.
* This parameter is not supported for [CreateFleet](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateFleet) . The `host` tenancy is not supported for [ImportInstance](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_ImportInstance.html) or for T3 instances that are configured for the `unlimited` CPU credit option.
*/
public data class Ec2FleetPlacementArgs(
public val affinity: Output? = null,
public val availabilityZone: Output? = null,
public val groupName: Output? = null,
public val hostId: Output? = null,
public val hostResourceGroupArn: Output? = null,
public val partitionNumber: Output? = null,
public val spreadDomain: Output? = null,
public val tenancy: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.ec2.inputs.Ec2FleetPlacementArgs =
com.pulumi.awsnative.ec2.inputs.Ec2FleetPlacementArgs.builder()
.affinity(affinity?.applyValue({ args0 -> args0 }))
.availabilityZone(availabilityZone?.applyValue({ args0 -> args0 }))
.groupName(groupName?.applyValue({ args0 -> args0 }))
.hostId(hostId?.applyValue({ args0 -> args0 }))
.hostResourceGroupArn(hostResourceGroupArn?.applyValue({ args0 -> args0 }))
.partitionNumber(partitionNumber?.applyValue({ args0 -> args0 }))
.spreadDomain(spreadDomain?.applyValue({ args0 -> args0 }))
.tenancy(tenancy?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [Ec2FleetPlacementArgs].
*/
@PulumiTagMarker
public class Ec2FleetPlacementArgsBuilder internal constructor() {
private var affinity: Output? = null
private var availabilityZone: Output? = null
private var groupName: Output? = null
private var hostId: Output? = null
private var hostResourceGroupArn: Output? = null
private var partitionNumber: Output? = null
private var spreadDomain: Output? = null
private var tenancy: Output? = null
/**
* @param value The affinity setting for the instance on the Dedicated Host.
* This parameter is not supported for [CreateFleet](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateFleet) or [ImportInstance](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_ImportInstance.html) .
*/
@JvmName("jleojmsvjpjsqgwc")
public suspend fun affinity(`value`: Output) {
this.affinity = value
}
/**
* @param value The Availability Zone of the instance.
* If not specified, an Availability Zone will be automatically chosen for you based on the load balancing criteria for the Region.
* This parameter is not supported for [CreateFleet](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateFleet) .
*/
@JvmName("vvhffcfbjhlhiejh")
public suspend fun availabilityZone(`value`: Output) {
this.availabilityZone = value
}
/**
* @param value The name of the placement group that the instance is in. If you specify `GroupName` , you can't specify `GroupId` .
*/
@JvmName("smxgicqoylebdvdc")
public suspend fun groupName(`value`: Output) {
this.groupName = value
}
/**
* @param value The ID of the Dedicated Host on which the instance resides.
* This parameter is not supported for [CreateFleet](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateFleet) or [ImportInstance](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_ImportInstance.html) .
*/
@JvmName("dacujhvoijifvmjq")
public suspend fun hostId(`value`: Output) {
this.hostId = value
}
/**
* @param value The ARN of the host resource group in which to launch the instances.
* If you specify this parameter, either omit the *Tenancy* parameter or set it to `host` .
* This parameter is not supported for [CreateFleet](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateFleet) .
*/
@JvmName("bpylcxqbjntnxbec")
public suspend fun hostResourceGroupArn(`value`: Output) {
this.hostResourceGroupArn = value
}
/**
* @param value The number of the partition that the instance is in. Valid only if the placement group strategy is set to `partition` .
* This parameter is not supported for [CreateFleet](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateFleet) .
*/
@JvmName("opnntyfaoludgljo")
public suspend fun partitionNumber(`value`: Output) {
this.partitionNumber = value
}
/**
* @param value Reserved for future use.
*/
@JvmName("shimjgeuwakffvsl")
public suspend fun spreadDomain(`value`: Output) {
this.spreadDomain = value
}
/**
* @param value The tenancy of the instance. An instance with a tenancy of `dedicated` runs on single-tenant hardware.
* This parameter is not supported for [CreateFleet](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateFleet) . The `host` tenancy is not supported for [ImportInstance](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_ImportInstance.html) or for T3 instances that are configured for the `unlimited` CPU credit option.
*/
@JvmName("aebeoarkanpwfpci")
public suspend fun tenancy(`value`: Output) {
this.tenancy = value
}
/**
* @param value The affinity setting for the instance on the Dedicated Host.
* This parameter is not supported for [CreateFleet](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateFleet) or [ImportInstance](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_ImportInstance.html) .
*/
@JvmName("qugolqmpaubebpqd")
public suspend fun affinity(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.affinity = mapped
}
/**
* @param value The Availability Zone of the instance.
* If not specified, an Availability Zone will be automatically chosen for you based on the load balancing criteria for the Region.
* This parameter is not supported for [CreateFleet](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateFleet) .
*/
@JvmName("ohgfhssowfurlaaf")
public suspend fun availabilityZone(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.availabilityZone = mapped
}
/**
* @param value The name of the placement group that the instance is in. If you specify `GroupName` , you can't specify `GroupId` .
*/
@JvmName("wqinrfpilfuvvvuq")
public suspend fun groupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.groupName = mapped
}
/**
* @param value The ID of the Dedicated Host on which the instance resides.
* This parameter is not supported for [CreateFleet](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateFleet) or [ImportInstance](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_ImportInstance.html) .
*/
@JvmName("xfshehfgsenmjqdy")
public suspend fun hostId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.hostId = mapped
}
/**
* @param value The ARN of the host resource group in which to launch the instances.
* If you specify this parameter, either omit the *Tenancy* parameter or set it to `host` .
* This parameter is not supported for [CreateFleet](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateFleet) .
*/
@JvmName("sldhkhglmxjevnkm")
public suspend fun hostResourceGroupArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.hostResourceGroupArn = mapped
}
/**
* @param value The number of the partition that the instance is in. Valid only if the placement group strategy is set to `partition` .
* This parameter is not supported for [CreateFleet](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateFleet) .
*/
@JvmName("oohxmdjbvbxncutp")
public suspend fun partitionNumber(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.partitionNumber = mapped
}
/**
* @param value Reserved for future use.
*/
@JvmName("mrggiivsuqneavvh")
public suspend fun spreadDomain(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.spreadDomain = mapped
}
/**
* @param value The tenancy of the instance. An instance with a tenancy of `dedicated` runs on single-tenant hardware.
* This parameter is not supported for [CreateFleet](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateFleet) . The `host` tenancy is not supported for [ImportInstance](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_ImportInstance.html) or for T3 instances that are configured for the `unlimited` CPU credit option.
*/
@JvmName("nnccrtyokgnrvkxp")
public suspend fun tenancy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tenancy = mapped
}
internal fun build(): Ec2FleetPlacementArgs = Ec2FleetPlacementArgs(
affinity = affinity,
availabilityZone = availabilityZone,
groupName = groupName,
hostId = hostId,
hostResourceGroupArn = hostResourceGroupArn,
partitionNumber = partitionNumber,
spreadDomain = spreadDomain,
tenancy = tenancy,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy