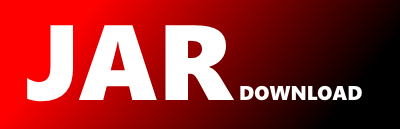
com.pulumi.awsnative.ec2.kotlin.inputs.InstanceEbsArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.ec2.kotlin.inputs
import com.pulumi.awsnative.ec2.inputs.InstanceEbsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property deleteOnTermination Indicates whether the EBS volume is deleted on instance termination.
* @property encrypted Indicates whether the volume should be encrypted.
* @property iops The number of I/O operations per second (IOPS). For gp3, io1, and io2 volumes, this represents the number of IOPS that are provisioned for the volume. For gp2 volumes, this represents the baseline performance of the volume and the rate at which the volume accumulates I/O credits for bursting.
* @property kmsKeyId The identifier of the AWS Key Management Service (AWS KMS) customer managed CMK to use for Amazon EBS encryption. If KmsKeyId is specified, the encrypted state must be true. If the encrypted state is true but you do not specify KmsKeyId, your AWS managed CMK for EBS is used.
* @property snapshotId The ID of the snapshot.
* @property volumeSize The size of the volume, in GiBs. You must specify either a snapshot ID or a volume size. If you specify a snapshot, the default is the snapshot size. You can specify a volume size that is equal to or larger than the snapshot size.
* @property volumeType The volume type.
*/
public data class InstanceEbsArgs(
public val deleteOnTermination: Output? = null,
public val encrypted: Output? = null,
public val iops: Output? = null,
public val kmsKeyId: Output? = null,
public val snapshotId: Output? = null,
public val volumeSize: Output? = null,
public val volumeType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.ec2.inputs.InstanceEbsArgs =
com.pulumi.awsnative.ec2.inputs.InstanceEbsArgs.builder()
.deleteOnTermination(deleteOnTermination?.applyValue({ args0 -> args0 }))
.encrypted(encrypted?.applyValue({ args0 -> args0 }))
.iops(iops?.applyValue({ args0 -> args0 }))
.kmsKeyId(kmsKeyId?.applyValue({ args0 -> args0 }))
.snapshotId(snapshotId?.applyValue({ args0 -> args0 }))
.volumeSize(volumeSize?.applyValue({ args0 -> args0 }))
.volumeType(volumeType?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [InstanceEbsArgs].
*/
@PulumiTagMarker
public class InstanceEbsArgsBuilder internal constructor() {
private var deleteOnTermination: Output? = null
private var encrypted: Output? = null
private var iops: Output? = null
private var kmsKeyId: Output? = null
private var snapshotId: Output? = null
private var volumeSize: Output? = null
private var volumeType: Output? = null
/**
* @param value Indicates whether the EBS volume is deleted on instance termination.
*/
@JvmName("bxfipckpssudyouq")
public suspend fun deleteOnTermination(`value`: Output) {
this.deleteOnTermination = value
}
/**
* @param value Indicates whether the volume should be encrypted.
*/
@JvmName("dgaqexgyonstljvr")
public suspend fun encrypted(`value`: Output) {
this.encrypted = value
}
/**
* @param value The number of I/O operations per second (IOPS). For gp3, io1, and io2 volumes, this represents the number of IOPS that are provisioned for the volume. For gp2 volumes, this represents the baseline performance of the volume and the rate at which the volume accumulates I/O credits for bursting.
*/
@JvmName("tnydhyjyshaobwpj")
public suspend fun iops(`value`: Output) {
this.iops = value
}
/**
* @param value The identifier of the AWS Key Management Service (AWS KMS) customer managed CMK to use for Amazon EBS encryption. If KmsKeyId is specified, the encrypted state must be true. If the encrypted state is true but you do not specify KmsKeyId, your AWS managed CMK for EBS is used.
*/
@JvmName("nlqjhlqhxhpbrgoa")
public suspend fun kmsKeyId(`value`: Output) {
this.kmsKeyId = value
}
/**
* @param value The ID of the snapshot.
*/
@JvmName("yxtleqqbgoijxwcx")
public suspend fun snapshotId(`value`: Output) {
this.snapshotId = value
}
/**
* @param value The size of the volume, in GiBs. You must specify either a snapshot ID or a volume size. If you specify a snapshot, the default is the snapshot size. You can specify a volume size that is equal to or larger than the snapshot size.
*/
@JvmName("axfaqyqkqgramqjt")
public suspend fun volumeSize(`value`: Output) {
this.volumeSize = value
}
/**
* @param value The volume type.
*/
@JvmName("ugaqlrarsupxywkf")
public suspend fun volumeType(`value`: Output) {
this.volumeType = value
}
/**
* @param value Indicates whether the EBS volume is deleted on instance termination.
*/
@JvmName("dsmekxkftjhyvvvl")
public suspend fun deleteOnTermination(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deleteOnTermination = mapped
}
/**
* @param value Indicates whether the volume should be encrypted.
*/
@JvmName("udiejfanywyuuwlj")
public suspend fun encrypted(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encrypted = mapped
}
/**
* @param value The number of I/O operations per second (IOPS). For gp3, io1, and io2 volumes, this represents the number of IOPS that are provisioned for the volume. For gp2 volumes, this represents the baseline performance of the volume and the rate at which the volume accumulates I/O credits for bursting.
*/
@JvmName("klnitbryxdpjxxkc")
public suspend fun iops(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.iops = mapped
}
/**
* @param value The identifier of the AWS Key Management Service (AWS KMS) customer managed CMK to use for Amazon EBS encryption. If KmsKeyId is specified, the encrypted state must be true. If the encrypted state is true but you do not specify KmsKeyId, your AWS managed CMK for EBS is used.
*/
@JvmName("wasrxvoihoyhlgub")
public suspend fun kmsKeyId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kmsKeyId = mapped
}
/**
* @param value The ID of the snapshot.
*/
@JvmName("ususjmhorjjotywe")
public suspend fun snapshotId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.snapshotId = mapped
}
/**
* @param value The size of the volume, in GiBs. You must specify either a snapshot ID or a volume size. If you specify a snapshot, the default is the snapshot size. You can specify a volume size that is equal to or larger than the snapshot size.
*/
@JvmName("umkiigqsygxbfcdt")
public suspend fun volumeSize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.volumeSize = mapped
}
/**
* @param value The volume type.
*/
@JvmName("ohoehpydemcrcehc")
public suspend fun volumeType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.volumeType = mapped
}
internal fun build(): InstanceEbsArgs = InstanceEbsArgs(
deleteOnTermination = deleteOnTermination,
encrypted = encrypted,
iops = iops,
kmsKeyId = kmsKeyId,
snapshotId = snapshotId,
volumeSize = volumeSize,
volumeType = volumeType,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy