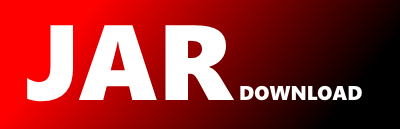
com.pulumi.awsnative.ec2.kotlin.inputs.LaunchTemplateEbsArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.ec2.kotlin.inputs
import com.pulumi.awsnative.ec2.inputs.LaunchTemplateEbsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Parameters for a block device for an EBS volume in an Amazon EC2 launch template.
* ``Ebs`` is a property of [AWS::EC2::LaunchTemplate BlockDeviceMapping](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ec2-launchtemplate-blockdevicemapping.html).
* @property deleteOnTermination Indicates whether the EBS volume is deleted on instance termination.
* @property encrypted Indicates whether the EBS volume is encrypted. Encrypted volumes can only be attached to instances that support Amazon EBS encryption. If you are creating a volume from a snapshot, you can't specify an encryption value.
* @property iops The number of I/O operations per second (IOPS). For ``gp3``, ``io1``, and ``io2`` volumes, this represents the number of IOPS that are provisioned for the volume. For ``gp2`` volumes, this represents the baseline performance of the volume and the rate at which the volume accumulates I/O credits for bursting.
* The following are the supported values for each volume type:
* + ``gp3``: 3,000 - 16,000 IOPS
* + ``io1``: 100 - 64,000 IOPS
* + ``io2``: 100 - 256,000 IOPS
* For ``io2`` volumes, you can achieve up to 256,000 IOPS on [instances built on the Nitro System](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/instance-types.html#ec2-nitro-instances). On other instances, you can achieve performance up to 32,000 IOPS.
* This parameter is supported for ``io1``, ``io2``, and ``gp3`` volumes only.
* @property kmsKeyId The ARN of the symmetric KMSlong (KMS) CMK used for encryption.
* @property snapshotId The ID of the snapshot.
* @property throughput The throughput to provision for a ``gp3`` volume, with a maximum of 1,000 MiB/s.
* Valid Range: Minimum value of 125. Maximum value of 1000.
* @property volumeSize The size of the volume, in GiBs. You must specify either a snapshot ID or a volume size. The following are the supported volumes sizes for each volume type:
* + ``gp2`` and ``gp3``: 1 - 16,384 GiB
* + ``io1``: 4 - 16,384 GiB
* + ``io2``: 4 - 65,536 GiB
* + ``st1`` and ``sc1``: 125 - 16,384 GiB
* + ``standard``: 1 - 1024 GiB
* @property volumeType The volume type. For more information, see [Amazon EBS volume types](https://docs.aws.amazon.com/ebs/latest/userguide/ebs-volume-types.html) in the *Amazon EBS User Guide*.
*/
public data class LaunchTemplateEbsArgs(
public val deleteOnTermination: Output? = null,
public val encrypted: Output? = null,
public val iops: Output? = null,
public val kmsKeyId: Output? = null,
public val snapshotId: Output? = null,
public val throughput: Output? = null,
public val volumeSize: Output? = null,
public val volumeType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.ec2.inputs.LaunchTemplateEbsArgs =
com.pulumi.awsnative.ec2.inputs.LaunchTemplateEbsArgs.builder()
.deleteOnTermination(deleteOnTermination?.applyValue({ args0 -> args0 }))
.encrypted(encrypted?.applyValue({ args0 -> args0 }))
.iops(iops?.applyValue({ args0 -> args0 }))
.kmsKeyId(kmsKeyId?.applyValue({ args0 -> args0 }))
.snapshotId(snapshotId?.applyValue({ args0 -> args0 }))
.throughput(throughput?.applyValue({ args0 -> args0 }))
.volumeSize(volumeSize?.applyValue({ args0 -> args0 }))
.volumeType(volumeType?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LaunchTemplateEbsArgs].
*/
@PulumiTagMarker
public class LaunchTemplateEbsArgsBuilder internal constructor() {
private var deleteOnTermination: Output? = null
private var encrypted: Output? = null
private var iops: Output? = null
private var kmsKeyId: Output? = null
private var snapshotId: Output? = null
private var throughput: Output? = null
private var volumeSize: Output? = null
private var volumeType: Output? = null
/**
* @param value Indicates whether the EBS volume is deleted on instance termination.
*/
@JvmName("chwdbrnqjvckybru")
public suspend fun deleteOnTermination(`value`: Output) {
this.deleteOnTermination = value
}
/**
* @param value Indicates whether the EBS volume is encrypted. Encrypted volumes can only be attached to instances that support Amazon EBS encryption. If you are creating a volume from a snapshot, you can't specify an encryption value.
*/
@JvmName("gthjxcpyednsffdj")
public suspend fun encrypted(`value`: Output) {
this.encrypted = value
}
/**
* @param value The number of I/O operations per second (IOPS). For ``gp3``, ``io1``, and ``io2`` volumes, this represents the number of IOPS that are provisioned for the volume. For ``gp2`` volumes, this represents the baseline performance of the volume and the rate at which the volume accumulates I/O credits for bursting.
* The following are the supported values for each volume type:
* + ``gp3``: 3,000 - 16,000 IOPS
* + ``io1``: 100 - 64,000 IOPS
* + ``io2``: 100 - 256,000 IOPS
* For ``io2`` volumes, you can achieve up to 256,000 IOPS on [instances built on the Nitro System](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/instance-types.html#ec2-nitro-instances). On other instances, you can achieve performance up to 32,000 IOPS.
* This parameter is supported for ``io1``, ``io2``, and ``gp3`` volumes only.
*/
@JvmName("qbeqccbjcidkbuap")
public suspend fun iops(`value`: Output) {
this.iops = value
}
/**
* @param value The ARN of the symmetric KMSlong (KMS) CMK used for encryption.
*/
@JvmName("ndwohvpeaextkssn")
public suspend fun kmsKeyId(`value`: Output) {
this.kmsKeyId = value
}
/**
* @param value The ID of the snapshot.
*/
@JvmName("ipyxdlyftaaajqtw")
public suspend fun snapshotId(`value`: Output) {
this.snapshotId = value
}
/**
* @param value The throughput to provision for a ``gp3`` volume, with a maximum of 1,000 MiB/s.
* Valid Range: Minimum value of 125. Maximum value of 1000.
*/
@JvmName("ldhusbppbrnocvyt")
public suspend fun throughput(`value`: Output) {
this.throughput = value
}
/**
* @param value The size of the volume, in GiBs. You must specify either a snapshot ID or a volume size. The following are the supported volumes sizes for each volume type:
* + ``gp2`` and ``gp3``: 1 - 16,384 GiB
* + ``io1``: 4 - 16,384 GiB
* + ``io2``: 4 - 65,536 GiB
* + ``st1`` and ``sc1``: 125 - 16,384 GiB
* + ``standard``: 1 - 1024 GiB
*/
@JvmName("aijyycnvvoupgkdh")
public suspend fun volumeSize(`value`: Output) {
this.volumeSize = value
}
/**
* @param value The volume type. For more information, see [Amazon EBS volume types](https://docs.aws.amazon.com/ebs/latest/userguide/ebs-volume-types.html) in the *Amazon EBS User Guide*.
*/
@JvmName("akerntalywxwgvgr")
public suspend fun volumeType(`value`: Output) {
this.volumeType = value
}
/**
* @param value Indicates whether the EBS volume is deleted on instance termination.
*/
@JvmName("grhsjmtxnnmdqypr")
public suspend fun deleteOnTermination(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deleteOnTermination = mapped
}
/**
* @param value Indicates whether the EBS volume is encrypted. Encrypted volumes can only be attached to instances that support Amazon EBS encryption. If you are creating a volume from a snapshot, you can't specify an encryption value.
*/
@JvmName("qysrjbidntcpvjks")
public suspend fun encrypted(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encrypted = mapped
}
/**
* @param value The number of I/O operations per second (IOPS). For ``gp3``, ``io1``, and ``io2`` volumes, this represents the number of IOPS that are provisioned for the volume. For ``gp2`` volumes, this represents the baseline performance of the volume and the rate at which the volume accumulates I/O credits for bursting.
* The following are the supported values for each volume type:
* + ``gp3``: 3,000 - 16,000 IOPS
* + ``io1``: 100 - 64,000 IOPS
* + ``io2``: 100 - 256,000 IOPS
* For ``io2`` volumes, you can achieve up to 256,000 IOPS on [instances built on the Nitro System](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/instance-types.html#ec2-nitro-instances). On other instances, you can achieve performance up to 32,000 IOPS.
* This parameter is supported for ``io1``, ``io2``, and ``gp3`` volumes only.
*/
@JvmName("pfwqaclbmwefvygc")
public suspend fun iops(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.iops = mapped
}
/**
* @param value The ARN of the symmetric KMSlong (KMS) CMK used for encryption.
*/
@JvmName("jwfugqdpgiecqdyo")
public suspend fun kmsKeyId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kmsKeyId = mapped
}
/**
* @param value The ID of the snapshot.
*/
@JvmName("fmotdtqjgtrwbbhu")
public suspend fun snapshotId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.snapshotId = mapped
}
/**
* @param value The throughput to provision for a ``gp3`` volume, with a maximum of 1,000 MiB/s.
* Valid Range: Minimum value of 125. Maximum value of 1000.
*/
@JvmName("djfqfwiuddeqfpce")
public suspend fun throughput(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.throughput = mapped
}
/**
* @param value The size of the volume, in GiBs. You must specify either a snapshot ID or a volume size. The following are the supported volumes sizes for each volume type:
* + ``gp2`` and ``gp3``: 1 - 16,384 GiB
* + ``io1``: 4 - 16,384 GiB
* + ``io2``: 4 - 65,536 GiB
* + ``st1`` and ``sc1``: 125 - 16,384 GiB
* + ``standard``: 1 - 1024 GiB
*/
@JvmName("dfnlkgeodnybjrsy")
public suspend fun volumeSize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.volumeSize = mapped
}
/**
* @param value The volume type. For more information, see [Amazon EBS volume types](https://docs.aws.amazon.com/ebs/latest/userguide/ebs-volume-types.html) in the *Amazon EBS User Guide*.
*/
@JvmName("hxvmnwdupubososp")
public suspend fun volumeType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.volumeType = mapped
}
internal fun build(): LaunchTemplateEbsArgs = LaunchTemplateEbsArgs(
deleteOnTermination = deleteOnTermination,
encrypted = encrypted,
iops = iops,
kmsKeyId = kmsKeyId,
snapshotId = snapshotId,
throughput = throughput,
volumeSize = volumeSize,
volumeType = volumeType,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy