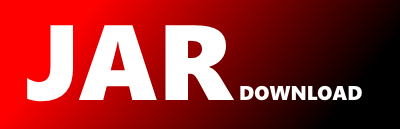
com.pulumi.awsnative.ec2.kotlin.inputs.LaunchTemplateSpotOptionsArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.ec2.kotlin.inputs
import com.pulumi.awsnative.ec2.inputs.LaunchTemplateSpotOptionsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Specifies options for Spot Instances.
* ``SpotOptions`` is a property of [AWS::EC2::LaunchTemplate InstanceMarketOptions](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ec2-launchtemplate-launchtemplatedata-instancemarketoptions.html).
* @property blockDurationMinutes Deprecated.
* @property instanceInterruptionBehavior The behavior when a Spot Instance is interrupted. The default is ``terminate``.
* @property maxPrice The maximum hourly price you're willing to pay for the Spot Instances. We do not recommend using this parameter because it can lead to increased interruptions. If you do not specify this parameter, you will pay the current Spot price.
* If you specify a maximum price, your Spot Instances will be interrupted more frequently than if you do not specify this parameter.
* @property spotInstanceType The Spot Instance request type.
* If you are using Spot Instances with an Auto Scaling group, use ``one-time`` requests, as the ASlong service handles requesting new Spot Instances whenever the group is below its desired capacity.
* @property validUntil The end date of the request, in UTC format (*YYYY-MM-DD*T*HH:MM:SS*Z). Supported only for persistent requests.
* + For a persistent request, the request remains active until the ``ValidUntil`` date and time is reached. Otherwise, the request remains active until you cancel it.
* + For a one-time request, ``ValidUntil`` is not supported. The request remains active until all instances launch or you cancel the request.
* Default: 7 days from the current date
*/
public data class LaunchTemplateSpotOptionsArgs(
public val blockDurationMinutes: Output? = null,
public val instanceInterruptionBehavior: Output? = null,
public val maxPrice: Output? = null,
public val spotInstanceType: Output? = null,
public val validUntil: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.ec2.inputs.LaunchTemplateSpotOptionsArgs =
com.pulumi.awsnative.ec2.inputs.LaunchTemplateSpotOptionsArgs.builder()
.blockDurationMinutes(blockDurationMinutes?.applyValue({ args0 -> args0 }))
.instanceInterruptionBehavior(instanceInterruptionBehavior?.applyValue({ args0 -> args0 }))
.maxPrice(maxPrice?.applyValue({ args0 -> args0 }))
.spotInstanceType(spotInstanceType?.applyValue({ args0 -> args0 }))
.validUntil(validUntil?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LaunchTemplateSpotOptionsArgs].
*/
@PulumiTagMarker
public class LaunchTemplateSpotOptionsArgsBuilder internal constructor() {
private var blockDurationMinutes: Output? = null
private var instanceInterruptionBehavior: Output? = null
private var maxPrice: Output? = null
private var spotInstanceType: Output? = null
private var validUntil: Output? = null
/**
* @param value Deprecated.
*/
@JvmName("ndqbhgudmhdlrmde")
public suspend fun blockDurationMinutes(`value`: Output) {
this.blockDurationMinutes = value
}
/**
* @param value The behavior when a Spot Instance is interrupted. The default is ``terminate``.
*/
@JvmName("lvogvtnbmrqjqnmk")
public suspend fun instanceInterruptionBehavior(`value`: Output) {
this.instanceInterruptionBehavior = value
}
/**
* @param value The maximum hourly price you're willing to pay for the Spot Instances. We do not recommend using this parameter because it can lead to increased interruptions. If you do not specify this parameter, you will pay the current Spot price.
* If you specify a maximum price, your Spot Instances will be interrupted more frequently than if you do not specify this parameter.
*/
@JvmName("rpyyruvtslnnbcra")
public suspend fun maxPrice(`value`: Output) {
this.maxPrice = value
}
/**
* @param value The Spot Instance request type.
* If you are using Spot Instances with an Auto Scaling group, use ``one-time`` requests, as the ASlong service handles requesting new Spot Instances whenever the group is below its desired capacity.
*/
@JvmName("fckcghucvghvhqdn")
public suspend fun spotInstanceType(`value`: Output) {
this.spotInstanceType = value
}
/**
* @param value The end date of the request, in UTC format (*YYYY-MM-DD*T*HH:MM:SS*Z). Supported only for persistent requests.
* + For a persistent request, the request remains active until the ``ValidUntil`` date and time is reached. Otherwise, the request remains active until you cancel it.
* + For a one-time request, ``ValidUntil`` is not supported. The request remains active until all instances launch or you cancel the request.
* Default: 7 days from the current date
*/
@JvmName("gvroearmrrrlmoap")
public suspend fun validUntil(`value`: Output) {
this.validUntil = value
}
/**
* @param value Deprecated.
*/
@JvmName("ulxltwgsmsammqmv")
public suspend fun blockDurationMinutes(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.blockDurationMinutes = mapped
}
/**
* @param value The behavior when a Spot Instance is interrupted. The default is ``terminate``.
*/
@JvmName("vsurqebpcnryywnj")
public suspend fun instanceInterruptionBehavior(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.instanceInterruptionBehavior = mapped
}
/**
* @param value The maximum hourly price you're willing to pay for the Spot Instances. We do not recommend using this parameter because it can lead to increased interruptions. If you do not specify this parameter, you will pay the current Spot price.
* If you specify a maximum price, your Spot Instances will be interrupted more frequently than if you do not specify this parameter.
*/
@JvmName("htkvhrwrsbkvpejt")
public suspend fun maxPrice(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxPrice = mapped
}
/**
* @param value The Spot Instance request type.
* If you are using Spot Instances with an Auto Scaling group, use ``one-time`` requests, as the ASlong service handles requesting new Spot Instances whenever the group is below its desired capacity.
*/
@JvmName("ejbajbetagixceuj")
public suspend fun spotInstanceType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.spotInstanceType = mapped
}
/**
* @param value The end date of the request, in UTC format (*YYYY-MM-DD*T*HH:MM:SS*Z). Supported only for persistent requests.
* + For a persistent request, the request remains active until the ``ValidUntil`` date and time is reached. Otherwise, the request remains active until you cancel it.
* + For a one-time request, ``ValidUntil`` is not supported. The request remains active until all instances launch or you cancel the request.
* Default: 7 days from the current date
*/
@JvmName("cnviapjssikokfve")
public suspend fun validUntil(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.validUntil = mapped
}
internal fun build(): LaunchTemplateSpotOptionsArgs = LaunchTemplateSpotOptionsArgs(
blockDurationMinutes = blockDurationMinutes,
instanceInterruptionBehavior = instanceInterruptionBehavior,
maxPrice = maxPrice,
spotInstanceType = spotInstanceType,
validUntil = validUntil,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy