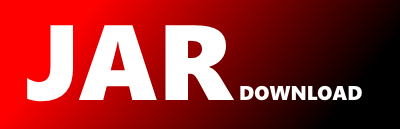
com.pulumi.awsnative.ec2.kotlin.inputs.SpotFleetEbsBlockDeviceArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.ec2.kotlin.inputs
import com.pulumi.awsnative.ec2.inputs.SpotFleetEbsBlockDeviceArgs.builder
import com.pulumi.awsnative.ec2.kotlin.enums.SpotFleetEbsBlockDeviceVolumeType
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property deleteOnTermination Indicates whether the EBS volume is deleted on instance termination. For more information, see [Preserving Amazon EBS volumes on instance termination](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/terminating-instances.html#preserving-volumes-on-termination) in the *Amazon EC2 User Guide* .
* @property encrypted Indicates whether the encryption state of an EBS volume is changed while being restored from a backing snapshot. The effect of setting the encryption state to `true` depends on the volume origin (new or from a snapshot), starting encryption state, ownership, and whether encryption by default is enabled. For more information, see [Amazon EBS Encryption](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/EBSEncryption.html#encryption-parameters) in the *Amazon EC2 User Guide* .
* In no case can you remove encryption from an encrypted volume.
* Encrypted volumes can only be attached to instances that support Amazon EBS encryption. For more information, see [Supported Instance Types](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/EBSEncryption.html#EBSEncryption_supported_instances) .
* This parameter is not returned by [DescribeImageAttribute](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_DescribeImageAttribute.html) .
* @property iops The number of I/O operations per second (IOPS). For `gp3` , `io1` , and `io2` volumes, this represents the number of IOPS that are provisioned for the volume. For `gp2` volumes, this represents the baseline performance of the volume and the rate at which the volume accumulates I/O credits for bursting.
* The following are the supported values for each volume type:
* - `gp3` : 3,000 - 16,000 IOPS
* - `io1` : 100 - 64,000 IOPS
* - `io2` : 100 - 256,000 IOPS
* For `io2` volumes, you can achieve up to 256,000 IOPS on [instances built on the Nitro System](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/instance-types.html#ec2-nitro-instances) . On other instances, you can achieve performance up to 32,000 IOPS.
* This parameter is required for `io1` and `io2` volumes. The default for `gp3` volumes is 3,000 IOPS.
* @property snapshotId The ID of the snapshot.
* @property volumeSize The size of the volume, in GiBs. You must specify either a snapshot ID or a volume size. If you specify a snapshot, the default is the snapshot size. You can specify a volume size that is equal to or larger than the snapshot size.
* The following are the supported sizes for each volume type:
* - `gp2` and `gp3` : 1 - 16,384 GiB
* - `io1` : 4 - 16,384 GiB
* - `io2` : 4 - 65,536 GiB
* - `st1` and `sc1` : 125 - 16,384 GiB
* - `standard` : 1 - 1024 GiB
* @property volumeType The volume type. For more information, see [Amazon EBS volume types](https://docs.aws.amazon.com/ebs/latest/userguide/ebs-volume-types.html) in the *Amazon EBS User Guide* .
*/
public data class SpotFleetEbsBlockDeviceArgs(
public val deleteOnTermination: Output? = null,
public val encrypted: Output? = null,
public val iops: Output? = null,
public val snapshotId: Output? = null,
public val volumeSize: Output? = null,
public val volumeType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.ec2.inputs.SpotFleetEbsBlockDeviceArgs =
com.pulumi.awsnative.ec2.inputs.SpotFleetEbsBlockDeviceArgs.builder()
.deleteOnTermination(deleteOnTermination?.applyValue({ args0 -> args0 }))
.encrypted(encrypted?.applyValue({ args0 -> args0 }))
.iops(iops?.applyValue({ args0 -> args0 }))
.snapshotId(snapshotId?.applyValue({ args0 -> args0 }))
.volumeSize(volumeSize?.applyValue({ args0 -> args0 }))
.volumeType(volumeType?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [SpotFleetEbsBlockDeviceArgs].
*/
@PulumiTagMarker
public class SpotFleetEbsBlockDeviceArgsBuilder internal constructor() {
private var deleteOnTermination: Output? = null
private var encrypted: Output? = null
private var iops: Output? = null
private var snapshotId: Output? = null
private var volumeSize: Output? = null
private var volumeType: Output? = null
/**
* @param value Indicates whether the EBS volume is deleted on instance termination. For more information, see [Preserving Amazon EBS volumes on instance termination](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/terminating-instances.html#preserving-volumes-on-termination) in the *Amazon EC2 User Guide* .
*/
@JvmName("riqxbvfxqeahqrxx")
public suspend fun deleteOnTermination(`value`: Output) {
this.deleteOnTermination = value
}
/**
* @param value Indicates whether the encryption state of an EBS volume is changed while being restored from a backing snapshot. The effect of setting the encryption state to `true` depends on the volume origin (new or from a snapshot), starting encryption state, ownership, and whether encryption by default is enabled. For more information, see [Amazon EBS Encryption](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/EBSEncryption.html#encryption-parameters) in the *Amazon EC2 User Guide* .
* In no case can you remove encryption from an encrypted volume.
* Encrypted volumes can only be attached to instances that support Amazon EBS encryption. For more information, see [Supported Instance Types](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/EBSEncryption.html#EBSEncryption_supported_instances) .
* This parameter is not returned by [DescribeImageAttribute](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_DescribeImageAttribute.html) .
*/
@JvmName("aumnneadwegiiqvs")
public suspend fun encrypted(`value`: Output) {
this.encrypted = value
}
/**
* @param value The number of I/O operations per second (IOPS). For `gp3` , `io1` , and `io2` volumes, this represents the number of IOPS that are provisioned for the volume. For `gp2` volumes, this represents the baseline performance of the volume and the rate at which the volume accumulates I/O credits for bursting.
* The following are the supported values for each volume type:
* - `gp3` : 3,000 - 16,000 IOPS
* - `io1` : 100 - 64,000 IOPS
* - `io2` : 100 - 256,000 IOPS
* For `io2` volumes, you can achieve up to 256,000 IOPS on [instances built on the Nitro System](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/instance-types.html#ec2-nitro-instances) . On other instances, you can achieve performance up to 32,000 IOPS.
* This parameter is required for `io1` and `io2` volumes. The default for `gp3` volumes is 3,000 IOPS.
*/
@JvmName("xascqsqgtabmbtem")
public suspend fun iops(`value`: Output) {
this.iops = value
}
/**
* @param value The ID of the snapshot.
*/
@JvmName("nknhnpfbshijydsq")
public suspend fun snapshotId(`value`: Output) {
this.snapshotId = value
}
/**
* @param value The size of the volume, in GiBs. You must specify either a snapshot ID or a volume size. If you specify a snapshot, the default is the snapshot size. You can specify a volume size that is equal to or larger than the snapshot size.
* The following are the supported sizes for each volume type:
* - `gp2` and `gp3` : 1 - 16,384 GiB
* - `io1` : 4 - 16,384 GiB
* - `io2` : 4 - 65,536 GiB
* - `st1` and `sc1` : 125 - 16,384 GiB
* - `standard` : 1 - 1024 GiB
*/
@JvmName("fnbdbhspmxkjcqpe")
public suspend fun volumeSize(`value`: Output) {
this.volumeSize = value
}
/**
* @param value The volume type. For more information, see [Amazon EBS volume types](https://docs.aws.amazon.com/ebs/latest/userguide/ebs-volume-types.html) in the *Amazon EBS User Guide* .
*/
@JvmName("yokyjkxkpfqevbwy")
public suspend fun volumeType(`value`: Output) {
this.volumeType = value
}
/**
* @param value Indicates whether the EBS volume is deleted on instance termination. For more information, see [Preserving Amazon EBS volumes on instance termination](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/terminating-instances.html#preserving-volumes-on-termination) in the *Amazon EC2 User Guide* .
*/
@JvmName("uviioghotgispigk")
public suspend fun deleteOnTermination(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deleteOnTermination = mapped
}
/**
* @param value Indicates whether the encryption state of an EBS volume is changed while being restored from a backing snapshot. The effect of setting the encryption state to `true` depends on the volume origin (new or from a snapshot), starting encryption state, ownership, and whether encryption by default is enabled. For more information, see [Amazon EBS Encryption](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/EBSEncryption.html#encryption-parameters) in the *Amazon EC2 User Guide* .
* In no case can you remove encryption from an encrypted volume.
* Encrypted volumes can only be attached to instances that support Amazon EBS encryption. For more information, see [Supported Instance Types](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/EBSEncryption.html#EBSEncryption_supported_instances) .
* This parameter is not returned by [DescribeImageAttribute](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_DescribeImageAttribute.html) .
*/
@JvmName("ffhgqounqywgbobt")
public suspend fun encrypted(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encrypted = mapped
}
/**
* @param value The number of I/O operations per second (IOPS). For `gp3` , `io1` , and `io2` volumes, this represents the number of IOPS that are provisioned for the volume. For `gp2` volumes, this represents the baseline performance of the volume and the rate at which the volume accumulates I/O credits for bursting.
* The following are the supported values for each volume type:
* - `gp3` : 3,000 - 16,000 IOPS
* - `io1` : 100 - 64,000 IOPS
* - `io2` : 100 - 256,000 IOPS
* For `io2` volumes, you can achieve up to 256,000 IOPS on [instances built on the Nitro System](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/instance-types.html#ec2-nitro-instances) . On other instances, you can achieve performance up to 32,000 IOPS.
* This parameter is required for `io1` and `io2` volumes. The default for `gp3` volumes is 3,000 IOPS.
*/
@JvmName("qjjywiipfdmwcxyx")
public suspend fun iops(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.iops = mapped
}
/**
* @param value The ID of the snapshot.
*/
@JvmName("gxtidamdhtnnyknc")
public suspend fun snapshotId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.snapshotId = mapped
}
/**
* @param value The size of the volume, in GiBs. You must specify either a snapshot ID or a volume size. If you specify a snapshot, the default is the snapshot size. You can specify a volume size that is equal to or larger than the snapshot size.
* The following are the supported sizes for each volume type:
* - `gp2` and `gp3` : 1 - 16,384 GiB
* - `io1` : 4 - 16,384 GiB
* - `io2` : 4 - 65,536 GiB
* - `st1` and `sc1` : 125 - 16,384 GiB
* - `standard` : 1 - 1024 GiB
*/
@JvmName("tvjlcchuiyvbrset")
public suspend fun volumeSize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.volumeSize = mapped
}
/**
* @param value The volume type. For more information, see [Amazon EBS volume types](https://docs.aws.amazon.com/ebs/latest/userguide/ebs-volume-types.html) in the *Amazon EBS User Guide* .
*/
@JvmName("yougfnsepueayasa")
public suspend fun volumeType(`value`: SpotFleetEbsBlockDeviceVolumeType?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.volumeType = mapped
}
internal fun build(): SpotFleetEbsBlockDeviceArgs = SpotFleetEbsBlockDeviceArgs(
deleteOnTermination = deleteOnTermination,
encrypted = encrypted,
iops = iops,
snapshotId = snapshotId,
volumeSize = volumeSize,
volumeType = volumeType,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy