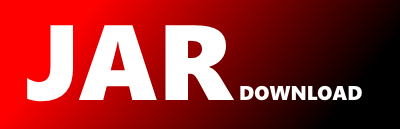
com.pulumi.awsnative.ec2.kotlin.outputs.SecurityGroupIngress.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.ec2.kotlin.outputs
import kotlin.Int
import kotlin.String
import kotlin.Suppress
/**
*
* @property cidrIp The IPv4 address range, in CIDR format.
* You must specify exactly one of the following: `CidrIp` , `CidrIpv6` , `SourcePrefixListId` , or `SourceSecurityGroupId` .
* For examples of rules that you can add to security groups for specific access scenarios, see [Security group rules for different use cases](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/security-group-rules-reference.html) in the *Amazon EC2 User Guide* .
* @property cidrIpv6 The IPv6 address range, in CIDR format.
* You must specify exactly one of the following: `CidrIp` , `CidrIpv6` , `SourcePrefixListId` , or `SourceSecurityGroupId` .
* For examples of rules that you can add to security groups for specific access scenarios, see [Security group rules for different use cases](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/security-group-rules-reference.html) in the *Amazon EC2 User Guide* .
* @property description Updates the description of an ingress (inbound) security group rule. You can replace an existing description, or add a description to a rule that did not have one previously.
* Constraints: Up to 255 characters in length. Allowed characters are a-z, A-Z, 0-9, spaces, and ._-:/()#,@[]+=;{}!$*
* @property fromPort If the protocol is TCP or UDP, this is the start of the port range. If the protocol is ICMP or ICMPv6, this is the ICMP type or -1 (all ICMP types).
* @property ipProtocol The IP protocol name ( `tcp` , `udp` , `icmp` , `icmpv6` ) or number (see [Protocol Numbers](https://docs.aws.amazon.com/http://www.iana.org/assignments/protocol-numbers/protocol-numbers.xhtml) ).
* Use `-1` to specify all protocols. When authorizing security group rules, specifying `-1` or a protocol number other than `tcp` , `udp` , `icmp` , or `icmpv6` allows traffic on all ports, regardless of any port range you specify. For `tcp` , `udp` , and `icmp` , you must specify a port range. For `icmpv6` , the port range is optional; if you omit the port range, traffic for all types and codes is allowed.
* @property sourcePrefixListId The ID of a prefix list.
* @property sourceSecurityGroupId The ID of the security group.
* @property sourceSecurityGroupName [Default VPC] The name of the source security group. You must specify either the security group ID or the security group name. You can't specify the group name in combination with an IP address range. Creates rules that grant full ICMP, UDP, and TCP access.
* For security groups in a nondefault VPC, you must specify the group ID.
* @property sourceSecurityGroupOwnerId [nondefault VPC] The AWS account ID for the source security group, if the source security group is in a different account. You can't specify this property with an IP address range. Creates rules that grant full ICMP, UDP, and TCP access.
* If you specify `SourceSecurityGroupName` or `SourceSecurityGroupId` and that security group is owned by a different account than the account creating the stack, you must specify the `SourceSecurityGroupOwnerId` ; otherwise, this property is optional.
* @property toPort If the protocol is TCP or UDP, this is the end of the port range. If the protocol is ICMP or ICMPv6, this is the ICMP code or -1 (all ICMP codes). If the start port is -1 (all ICMP types), then the end port must be -1 (all ICMP codes).
*/
public data class SecurityGroupIngress(
public val cidrIp: String? = null,
public val cidrIpv6: String? = null,
public val description: String? = null,
public val fromPort: Int? = null,
public val ipProtocol: String,
public val sourcePrefixListId: String? = null,
public val sourceSecurityGroupId: String? = null,
public val sourceSecurityGroupName: String? = null,
public val sourceSecurityGroupOwnerId: String? = null,
public val toPort: Int? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.awsnative.ec2.outputs.SecurityGroupIngress): SecurityGroupIngress = SecurityGroupIngress(
cidrIp = javaType.cidrIp().map({ args0 -> args0 }).orElse(null),
cidrIpv6 = javaType.cidrIpv6().map({ args0 -> args0 }).orElse(null),
description = javaType.description().map({ args0 -> args0 }).orElse(null),
fromPort = javaType.fromPort().map({ args0 -> args0 }).orElse(null),
ipProtocol = javaType.ipProtocol(),
sourcePrefixListId = javaType.sourcePrefixListId().map({ args0 -> args0 }).orElse(null),
sourceSecurityGroupId = javaType.sourceSecurityGroupId().map({ args0 -> args0 }).orElse(null),
sourceSecurityGroupName = javaType.sourceSecurityGroupName().map({ args0 -> args0 }).orElse(null),
sourceSecurityGroupOwnerId = javaType.sourceSecurityGroupOwnerId().map({ args0 ->
args0
}).orElse(null),
toPort = javaType.toPort().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy