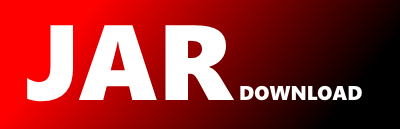
com.pulumi.awsnative.efs.kotlin.EfsFunctions.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.efs.kotlin
import com.pulumi.awsnative.efs.EfsFunctions.getAccessPointPlain
import com.pulumi.awsnative.efs.EfsFunctions.getFileSystemPlain
import com.pulumi.awsnative.efs.EfsFunctions.getMountTargetPlain
import com.pulumi.awsnative.efs.kotlin.inputs.GetAccessPointPlainArgs
import com.pulumi.awsnative.efs.kotlin.inputs.GetAccessPointPlainArgsBuilder
import com.pulumi.awsnative.efs.kotlin.inputs.GetFileSystemPlainArgs
import com.pulumi.awsnative.efs.kotlin.inputs.GetFileSystemPlainArgsBuilder
import com.pulumi.awsnative.efs.kotlin.inputs.GetMountTargetPlainArgs
import com.pulumi.awsnative.efs.kotlin.inputs.GetMountTargetPlainArgsBuilder
import com.pulumi.awsnative.efs.kotlin.outputs.GetAccessPointResult
import com.pulumi.awsnative.efs.kotlin.outputs.GetFileSystemResult
import com.pulumi.awsnative.efs.kotlin.outputs.GetMountTargetResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.efs.kotlin.outputs.GetAccessPointResult.Companion.toKotlin as getAccessPointResultToKotlin
import com.pulumi.awsnative.efs.kotlin.outputs.GetFileSystemResult.Companion.toKotlin as getFileSystemResultToKotlin
import com.pulumi.awsnative.efs.kotlin.outputs.GetMountTargetResult.Companion.toKotlin as getMountTargetResultToKotlin
public object EfsFunctions {
/**
* The ``AWS::EFS::AccessPoint`` resource creates an EFS access point. An access point is an application-specific view into an EFS file system that applies an operating system user and group, and a file system path, to any file system request made through the access point. The operating system user and group override any identity information provided by the NFS client. The file system path is exposed as the access point's root directory. Applications using the access point can only access data in its own directory and below. To learn more, see [Mounting a file system using EFS access points](https://docs.aws.amazon.com/efs/latest/ug/efs-access-points.html).
* This operation requires permissions for the ``elasticfilesystem:CreateAccessPoint`` action.
* @param argument null
* @return null
*/
public suspend fun getAccessPoint(argument: GetAccessPointPlainArgs): GetAccessPointResult =
getAccessPointResultToKotlin(getAccessPointPlain(argument.toJava()).await())
/**
* @see [getAccessPoint].
* @param accessPointId The ID of the EFS access point.
* @return null
*/
public suspend fun getAccessPoint(accessPointId: String): GetAccessPointResult {
val argument = GetAccessPointPlainArgs(
accessPointId = accessPointId,
)
return getAccessPointResultToKotlin(getAccessPointPlain(argument.toJava()).await())
}
/**
* @see [getAccessPoint].
* @param argument Builder for [com.pulumi.awsnative.efs.kotlin.inputs.GetAccessPointPlainArgs].
* @return null
*/
public suspend fun getAccessPoint(argument: suspend GetAccessPointPlainArgsBuilder.() -> Unit): GetAccessPointResult {
val builder = GetAccessPointPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAccessPointResultToKotlin(getAccessPointPlain(builtArgument.toJava()).await())
}
/**
* The ``AWS::EFS::FileSystem`` resource creates a new, empty file system in EFSlong (EFS). You must create a mount target ([AWS::EFS::MountTarget](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-efs-mounttarget.html)) to mount your EFS file system on an EC2 or other AWS cloud compute resource.
* @param argument null
* @return null
*/
public suspend fun getFileSystem(argument: GetFileSystemPlainArgs): GetFileSystemResult =
getFileSystemResultToKotlin(getFileSystemPlain(argument.toJava()).await())
/**
* @see [getFileSystem].
* @param fileSystemId The ID of the EFS file system. For example: `fs-abcdef0123456789a`
* @return null
*/
public suspend fun getFileSystem(fileSystemId: String): GetFileSystemResult {
val argument = GetFileSystemPlainArgs(
fileSystemId = fileSystemId,
)
return getFileSystemResultToKotlin(getFileSystemPlain(argument.toJava()).await())
}
/**
* @see [getFileSystem].
* @param argument Builder for [com.pulumi.awsnative.efs.kotlin.inputs.GetFileSystemPlainArgs].
* @return null
*/
public suspend fun getFileSystem(argument: suspend GetFileSystemPlainArgsBuilder.() -> Unit): GetFileSystemResult {
val builder = GetFileSystemPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getFileSystemResultToKotlin(getFileSystemPlain(builtArgument.toJava()).await())
}
/**
* The ``AWS::EFS::MountTarget`` resource is an Amazon EFS resource that creates a mount target for an EFS file system. You can then mount the file system on Amazon EC2 instances or other resources by using the mount target.
* @param argument null
* @return null
*/
public suspend fun getMountTarget(argument: GetMountTargetPlainArgs): GetMountTargetResult =
getMountTargetResultToKotlin(getMountTargetPlain(argument.toJava()).await())
/**
* @see [getMountTarget].
* @param id The ID of the Amazon EFS file system that the mount target provides access to.
* Example: `fs-0123456789111222a`
* @return null
*/
public suspend fun getMountTarget(id: String): GetMountTargetResult {
val argument = GetMountTargetPlainArgs(
id = id,
)
return getMountTargetResultToKotlin(getMountTargetPlain(argument.toJava()).await())
}
/**
* @see [getMountTarget].
* @param argument Builder for [com.pulumi.awsnative.efs.kotlin.inputs.GetMountTargetPlainArgs].
* @return null
*/
public suspend fun getMountTarget(argument: suspend GetMountTargetPlainArgsBuilder.() -> Unit): GetMountTargetResult {
val builder = GetMountTargetPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getMountTargetResultToKotlin(getMountTargetPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy