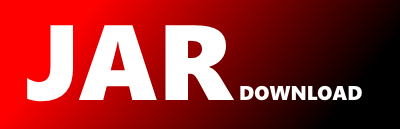
com.pulumi.awsnative.efs.kotlin.inputs.AccessPointCreationInfoArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.efs.kotlin.inputs
import com.pulumi.awsnative.efs.inputs.AccessPointCreationInfoArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Required if the ``RootDirectory`` > ``Path`` specified does not exist. Specifies the POSIX IDs and permissions to apply to the access point's ``RootDirectory`` > ``Path``. If the access point root directory does not exist, EFS creates it with these settings when a client connects to the access point. When specifying ``CreationInfo``, you must include values for all properties.
* Amazon EFS creates a root directory only if you have provided the CreationInfo: OwnUid, OwnGID, and permissions for the directory. If you do not provide this information, Amazon EFS does not create the root directory. If the root directory does not exist, attempts to mount using the access point will fail.
* If you do not provide ``CreationInfo`` and the specified ``RootDirectory`` does not exist, attempts to mount the file system using the access point will fail.
* @property ownerGid Specifies the POSIX group ID to apply to the ``RootDirectory``. Accepts values from 0 to 2^32 (4294967295).
* @property ownerUid Specifies the POSIX user ID to apply to the ``RootDirectory``. Accepts values from 0 to 2^32 (4294967295).
* @property permissions Specifies the POSIX permissions to apply to the ``RootDirectory``, in the format of an octal number representing the file's mode bits.
*/
public data class AccessPointCreationInfoArgs(
public val ownerGid: Output,
public val ownerUid: Output,
public val permissions: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.efs.inputs.AccessPointCreationInfoArgs =
com.pulumi.awsnative.efs.inputs.AccessPointCreationInfoArgs.builder()
.ownerGid(ownerGid.applyValue({ args0 -> args0 }))
.ownerUid(ownerUid.applyValue({ args0 -> args0 }))
.permissions(permissions.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AccessPointCreationInfoArgs].
*/
@PulumiTagMarker
public class AccessPointCreationInfoArgsBuilder internal constructor() {
private var ownerGid: Output? = null
private var ownerUid: Output? = null
private var permissions: Output? = null
/**
* @param value Specifies the POSIX group ID to apply to the ``RootDirectory``. Accepts values from 0 to 2^32 (4294967295).
*/
@JvmName("vpqnorklmyikbesc")
public suspend fun ownerGid(`value`: Output) {
this.ownerGid = value
}
/**
* @param value Specifies the POSIX user ID to apply to the ``RootDirectory``. Accepts values from 0 to 2^32 (4294967295).
*/
@JvmName("wnrwnetwjvirembo")
public suspend fun ownerUid(`value`: Output) {
this.ownerUid = value
}
/**
* @param value Specifies the POSIX permissions to apply to the ``RootDirectory``, in the format of an octal number representing the file's mode bits.
*/
@JvmName("hflktlceexymxomd")
public suspend fun permissions(`value`: Output) {
this.permissions = value
}
/**
* @param value Specifies the POSIX group ID to apply to the ``RootDirectory``. Accepts values from 0 to 2^32 (4294967295).
*/
@JvmName("yldnaictglqufnmq")
public suspend fun ownerGid(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.ownerGid = mapped
}
/**
* @param value Specifies the POSIX user ID to apply to the ``RootDirectory``. Accepts values from 0 to 2^32 (4294967295).
*/
@JvmName("iluufjjbiqtrvsyy")
public suspend fun ownerUid(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.ownerUid = mapped
}
/**
* @param value Specifies the POSIX permissions to apply to the ``RootDirectory``, in the format of an octal number representing the file's mode bits.
*/
@JvmName("dahwegrfdyllfnfv")
public suspend fun permissions(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.permissions = mapped
}
internal fun build(): AccessPointCreationInfoArgs = AccessPointCreationInfoArgs(
ownerGid = ownerGid ?: throw PulumiNullFieldException("ownerGid"),
ownerUid = ownerUid ?: throw PulumiNullFieldException("ownerUid"),
permissions = permissions ?: throw PulumiNullFieldException("permissions"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy