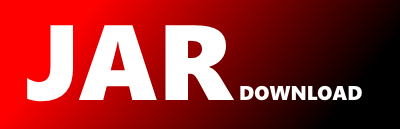
com.pulumi.awsnative.efs.kotlin.inputs.FileSystemLifecyclePolicyArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.efs.kotlin.inputs
import com.pulumi.awsnative.efs.inputs.FileSystemLifecyclePolicyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Describes a policy used by Lifecycle management that specifies when to transition files into and out of the EFS storage classes. For more information, see [Managing file system storage](https://docs.aws.amazon.com/efs/latest/ug/lifecycle-management-efs.html).
* + Each ``LifecyclePolicy`` object can have only a single transition. This means that in a request body, ``LifecyclePolicies`` must be structured as an array of ``LifecyclePolicy`` objects, one object for each transition, ``TransitionToIA``, ``TransitionToArchive``, ``TransitionToPrimaryStorageClass``.
* + See the AWS::EFS::FileSystem examples for the correct ``LifecyclePolicy`` structure. Do not use the syntax shown on this page.
* @property transitionToArchive The number of days after files were last accessed in primary storage (the Standard storage class) at which to move them to Archive storage. Metadata operations such as listing the contents of a directory don't count as file access events.
* @property transitionToIa The number of days after files were last accessed in primary storage (the Standard storage class) at which to move them to Infrequent Access (IA) storage. Metadata operations such as listing the contents of a directory don't count as file access events.
* @property transitionToPrimaryStorageClass Whether to move files back to primary (Standard) storage after they are accessed in IA or Archive storage. Metadata operations such as listing the contents of a directory don't count as file access events.
*/
public data class FileSystemLifecyclePolicyArgs(
public val transitionToArchive: Output? = null,
public val transitionToIa: Output? = null,
public val transitionToPrimaryStorageClass: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.efs.inputs.FileSystemLifecyclePolicyArgs =
com.pulumi.awsnative.efs.inputs.FileSystemLifecyclePolicyArgs.builder()
.transitionToArchive(transitionToArchive?.applyValue({ args0 -> args0 }))
.transitionToIa(transitionToIa?.applyValue({ args0 -> args0 }))
.transitionToPrimaryStorageClass(
transitionToPrimaryStorageClass?.applyValue({ args0 ->
args0
}),
).build()
}
/**
* Builder for [FileSystemLifecyclePolicyArgs].
*/
@PulumiTagMarker
public class FileSystemLifecyclePolicyArgsBuilder internal constructor() {
private var transitionToArchive: Output? = null
private var transitionToIa: Output? = null
private var transitionToPrimaryStorageClass: Output? = null
/**
* @param value The number of days after files were last accessed in primary storage (the Standard storage class) at which to move them to Archive storage. Metadata operations such as listing the contents of a directory don't count as file access events.
*/
@JvmName("bwbbpkupfxpinvxm")
public suspend fun transitionToArchive(`value`: Output) {
this.transitionToArchive = value
}
/**
* @param value The number of days after files were last accessed in primary storage (the Standard storage class) at which to move them to Infrequent Access (IA) storage. Metadata operations such as listing the contents of a directory don't count as file access events.
*/
@JvmName("qnwtnjkgkvvplndv")
public suspend fun transitionToIa(`value`: Output) {
this.transitionToIa = value
}
/**
* @param value Whether to move files back to primary (Standard) storage after they are accessed in IA or Archive storage. Metadata operations such as listing the contents of a directory don't count as file access events.
*/
@JvmName("fgfstgxpqnfxfqvg")
public suspend fun transitionToPrimaryStorageClass(`value`: Output) {
this.transitionToPrimaryStorageClass = value
}
/**
* @param value The number of days after files were last accessed in primary storage (the Standard storage class) at which to move them to Archive storage. Metadata operations such as listing the contents of a directory don't count as file access events.
*/
@JvmName("mtpnhbcuvxqkwtyr")
public suspend fun transitionToArchive(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.transitionToArchive = mapped
}
/**
* @param value The number of days after files were last accessed in primary storage (the Standard storage class) at which to move them to Infrequent Access (IA) storage. Metadata operations such as listing the contents of a directory don't count as file access events.
*/
@JvmName("fgdvrmudpndpgacy")
public suspend fun transitionToIa(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.transitionToIa = mapped
}
/**
* @param value Whether to move files back to primary (Standard) storage after they are accessed in IA or Archive storage. Metadata operations such as listing the contents of a directory don't count as file access events.
*/
@JvmName("irakektidpxguxxo")
public suspend fun transitionToPrimaryStorageClass(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.transitionToPrimaryStorageClass = mapped
}
internal fun build(): FileSystemLifecyclePolicyArgs = FileSystemLifecyclePolicyArgs(
transitionToArchive = transitionToArchive,
transitionToIa = transitionToIa,
transitionToPrimaryStorageClass = transitionToPrimaryStorageClass,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy