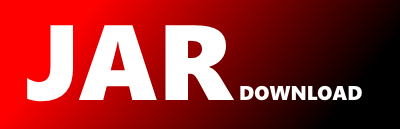
com.pulumi.awsnative.efs.kotlin.outputs.GetFileSystemResult.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.efs.kotlin.outputs
import com.pulumi.awsnative.kotlin.outputs.Tag
import kotlin.Any
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property arn The Amazon Resource Name (ARN) of the EFS file system.
* Example: `arn:aws:elasticfilesystem:us-west-2:1111333322228888:file-system/fs-0123456789abcdef8`
* @property backupPolicy Use the ``BackupPolicy`` to turn automatic backups on or off for the file system.
* @property fileSystemId The ID of the EFS file system. For example: `fs-abcdef0123456789a`
* @property fileSystemPolicy The ``FileSystemPolicy`` for the EFS file system. A file system policy is an IAM resource policy used to control NFS access to an EFS file system. For more information, see [Using to control NFS access to Amazon EFS](https://docs.aws.amazon.com/efs/latest/ug/iam-access-control-nfs-efs.html) in the *Amazon EFS User Guide*.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::EFS::FileSystem` for more information about the expected schema for this property.
* @property fileSystemProtection Describes the protection on the file system.
* @property fileSystemTags Use to create one or more tags associated with the file system. Each tag is a user-defined key-value pair. Name your file system on creation by including a ``"Key":"Name","Value":"{value}"`` key-value pair. Each key must be unique. For more information, see [Tagging resources](https://docs.aws.amazon.com/general/latest/gr/aws_tagging.html) in the *General Reference Guide*.
* @property lifecyclePolicies An array of ``LifecyclePolicy`` objects that define the file system's ``LifecycleConfiguration`` object. A ``LifecycleConfiguration`` object informs Lifecycle management of the following:
* + When to move files in the file system from primary storage to IA storage.
* + When to move files in the file system from primary storage or IA storage to Archive storage.
* + When to move files that are in IA or Archive storage to primary storage.
* EFS requires that each ``LifecyclePolicy`` object have only a single transition. This means that in a request body, ``LifecyclePolicies`` needs to be structured as an array of ``LifecyclePolicy`` objects, one object for each transition, ``TransitionToIA``, ``TransitionToArchive`` ``TransitionToPrimaryStorageClass``. See the example requests in the following section for more information.
* @property provisionedThroughputInMibps The throughput, measured in mebibytes per second (MiBps), that you want to provision for a file system that you're creating. Required if ``ThroughputMode`` is set to ``provisioned``. Valid values are 1-3414 MiBps, with the upper limit depending on Region. To increase this limit, contact SUP. For more information, see [Amazon EFS quotas that you can increase](https://docs.aws.amazon.com/efs/latest/ug/limits.html#soft-limits) in the *Amazon EFS User Guide*.
* @property replicationConfiguration Describes the replication configuration for a specific file system.
* @property throughputMode Specifies the throughput mode for the file system. The mode can be ``bursting``, ``provisioned``, or ``elastic``. If you set ``ThroughputMode`` to ``provisioned``, you must also set a value for ``ProvisionedThroughputInMibps``. After you create the file system, you can decrease your file system's Provisioned throughput or change between the throughput modes, with certain time restrictions. For more information, see [Specifying throughput with provisioned mode](https://docs.aws.amazon.com/efs/latest/ug/performance.html#provisioned-throughput) in the *Amazon EFS User Guide*.
* Default is ``bursting``.
*/
public data class GetFileSystemResult(
public val arn: String? = null,
public val backupPolicy: FileSystemBackupPolicy? = null,
public val fileSystemId: String? = null,
public val fileSystemPolicy: Any? = null,
public val fileSystemProtection: FileSystemProtection? = null,
public val fileSystemTags: List? = null,
public val lifecyclePolicies: List? = null,
public val provisionedThroughputInMibps: Double? = null,
public val replicationConfiguration: FileSystemReplicationConfiguration? = null,
public val throughputMode: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.awsnative.efs.outputs.GetFileSystemResult): GetFileSystemResult = GetFileSystemResult(
arn = javaType.arn().map({ args0 -> args0 }).orElse(null),
backupPolicy = javaType.backupPolicy().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.efs.kotlin.outputs.FileSystemBackupPolicy.Companion.toKotlin(args0)
})
}).orElse(null),
fileSystemId = javaType.fileSystemId().map({ args0 -> args0 }).orElse(null),
fileSystemPolicy = javaType.fileSystemPolicy().map({ args0 -> args0 }).orElse(null),
fileSystemProtection = javaType.fileSystemProtection().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.efs.kotlin.outputs.FileSystemProtection.Companion.toKotlin(args0)
})
}).orElse(null),
fileSystemTags = javaType.fileSystemTags().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin(args0)
})
}),
lifecyclePolicies = javaType.lifecyclePolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.efs.kotlin.outputs.FileSystemLifecyclePolicy.Companion.toKotlin(args0)
})
}),
provisionedThroughputInMibps = javaType.provisionedThroughputInMibps().map({ args0 ->
args0
}).orElse(null),
replicationConfiguration = javaType.replicationConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.efs.kotlin.outputs.FileSystemReplicationConfiguration.Companion.toKotlin(args0)
})
}).orElse(null),
throughputMode = javaType.throughputMode().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy