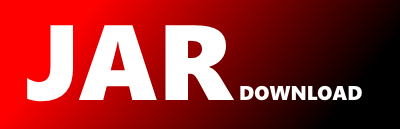
com.pulumi.awsnative.eks.kotlin.Cluster.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.eks.kotlin
import com.pulumi.awsnative.eks.kotlin.outputs.ClusterAccessConfig
import com.pulumi.awsnative.eks.kotlin.outputs.ClusterEncryptionConfig
import com.pulumi.awsnative.eks.kotlin.outputs.ClusterKubernetesNetworkConfig
import com.pulumi.awsnative.eks.kotlin.outputs.ClusterOutpostConfig
import com.pulumi.awsnative.eks.kotlin.outputs.ClusterResourcesVpcConfig
import com.pulumi.awsnative.eks.kotlin.outputs.ClusterUpgradePolicy
import com.pulumi.awsnative.eks.kotlin.outputs.Logging
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.eks.kotlin.outputs.ClusterAccessConfig.Companion.toKotlin as clusterAccessConfigToKotlin
import com.pulumi.awsnative.eks.kotlin.outputs.ClusterEncryptionConfig.Companion.toKotlin as clusterEncryptionConfigToKotlin
import com.pulumi.awsnative.eks.kotlin.outputs.ClusterKubernetesNetworkConfig.Companion.toKotlin as clusterKubernetesNetworkConfigToKotlin
import com.pulumi.awsnative.eks.kotlin.outputs.ClusterOutpostConfig.Companion.toKotlin as clusterOutpostConfigToKotlin
import com.pulumi.awsnative.eks.kotlin.outputs.ClusterResourcesVpcConfig.Companion.toKotlin as clusterResourcesVpcConfigToKotlin
import com.pulumi.awsnative.eks.kotlin.outputs.ClusterUpgradePolicy.Companion.toKotlin as clusterUpgradePolicyToKotlin
import com.pulumi.awsnative.eks.kotlin.outputs.Logging.Companion.toKotlin as loggingToKotlin
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin as tagToKotlin
/**
* Builder for [Cluster].
*/
@PulumiTagMarker
public class ClusterResourceBuilder internal constructor() {
public var name: String? = null
public var args: ClusterArgs = ClusterArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ClusterArgsBuilder.() -> Unit) {
val builder = ClusterArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Cluster {
val builtJavaResource = com.pulumi.awsnative.eks.Cluster(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Cluster(builtJavaResource)
}
}
/**
* An object representing an Amazon EKS cluster.
*/
public class Cluster internal constructor(
override val javaResource: com.pulumi.awsnative.eks.Cluster,
) : KotlinCustomResource(javaResource, ClusterMapper) {
/**
* The access configuration for the cluster.
*/
public val accessConfig: Output?
get() = javaResource.accessConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
clusterAccessConfigToKotlin(args0)
})
}).orElse(null)
})
/**
* The ARN of the cluster, such as arn:aws:eks:us-west-2:666666666666:cluster/prod.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* The unique ID given to your cluster.
*/
public val awsId: Output
get() = javaResource.awsId().applyValue({ args0 -> args0 })
/**
* Set this value to false to avoid creating the default networking addons when the cluster is created.
*/
public val bootstrapSelfManagedAddons: Output?
get() = javaResource.bootstrapSelfManagedAddons().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The certificate-authority-data for your cluster.
*/
public val certificateAuthorityData: Output
get() = javaResource.certificateAuthorityData().applyValue({ args0 -> args0 })
/**
* The cluster security group that was created by Amazon EKS for the cluster. Managed node groups use this security group for control plane to data plane communication.
*/
public val clusterSecurityGroupId: Output
get() = javaResource.clusterSecurityGroupId().applyValue({ args0 -> args0 })
/**
* The encryption configuration for the cluster.
*/
public val encryptionConfig: Output>?
get() = javaResource.encryptionConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
clusterEncryptionConfigToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Amazon Resource Name (ARN) or alias of the customer master key (CMK).
*/
public val encryptionConfigKeyArn: Output
get() = javaResource.encryptionConfigKeyArn().applyValue({ args0 -> args0 })
/**
* The endpoint for your Kubernetes API server, such as https://5E1D0CEXAMPLEA591B746AFC5AB30262.yl4.us-west-2.eks.amazonaws.com.
*/
public val endpoint: Output
get() = javaResource.endpoint().applyValue({ args0 -> args0 })
/**
* The Kubernetes network configuration for the cluster.
*/
public val kubernetesNetworkConfig: Output?
get() = javaResource.kubernetesNetworkConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> clusterKubernetesNetworkConfigToKotlin(args0) })
}).orElse(null)
})
/**
* The logging configuration for your cluster.
*/
public val logging: Output?
get() = javaResource.logging().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
loggingToKotlin(args0)
})
}).orElse(null)
})
/**
* The unique name to give to your cluster.
*/
public val name: Output?
get() = javaResource.name().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The issuer URL for the cluster's OIDC identity provider, such as https://oidc.eks.us-west-2.amazonaws.com/id/EXAMPLED539D4633E53DE1B716D3041E. If you need to remove https:// from this output value, you can include the following code in your template.
*/
public val openIdConnectIssuerUrl: Output
get() = javaResource.openIdConnectIssuerUrl().applyValue({ args0 -> args0 })
/**
* An object representing the configuration of your local Amazon EKS cluster on an AWS Outpost. This object isn't available for clusters on the AWS cloud.
*/
public val outpostConfig: Output?
get() = javaResource.outpostConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> clusterOutpostConfigToKotlin(args0) })
}).orElse(null)
})
/**
* The VPC configuration that's used by the cluster control plane. Amazon EKS VPC resources have specific requirements to work properly with Kubernetes. For more information, see [Cluster VPC Considerations](https://docs.aws.amazon.com/eks/latest/userguide/network_reqs.html) and [Cluster Security Group Considerations](https://docs.aws.amazon.com/eks/latest/userguide/sec-group-reqs.html) in the *Amazon EKS User Guide* . You must specify at least two subnets. You can specify up to five security groups, but we recommend that you use a dedicated security group for your cluster control plane.
*/
public val resourcesVpcConfig: Output
get() = javaResource.resourcesVpcConfig().applyValue({ args0 ->
args0.let({ args0 ->
clusterResourcesVpcConfigToKotlin(args0)
})
})
/**
* The Amazon Resource Name (ARN) of the IAM role that provides permissions for the Kubernetes control plane to make calls to AWS API operations on your behalf.
*/
public val roleArn: Output
get() = javaResource.roleArn().applyValue({ args0 -> args0 })
/**
* An array of key-value pairs to apply to this resource.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> tagToKotlin(args0) })
})
}).orElse(null)
})
/**
* This value indicates if extended support is enabled or disabled for the cluster.
* [Learn more about EKS Extended Support in the EKS User Guide.](https://docs.aws.amazon.com/eks/latest/userguide/extended-support-control.html)
*/
public val upgradePolicy: Output?
get() = javaResource.upgradePolicy().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> clusterUpgradePolicyToKotlin(args0) })
}).orElse(null)
})
/**
* The desired Kubernetes version for your cluster. If you don't specify a value here, the latest version available in Amazon EKS is used.
*/
public val version: Output?
get() = javaResource.version().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
}
public object ClusterMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.eks.Cluster::class == javaResource::class
override fun map(javaResource: Resource): Cluster = Cluster(
javaResource as
com.pulumi.awsnative.eks.Cluster,
)
}
/**
* @see [Cluster].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Cluster].
*/
public suspend fun cluster(name: String, block: suspend ClusterResourceBuilder.() -> Unit): Cluster {
val builder = ClusterResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Cluster].
* @param name The _unique_ name of the resulting resource.
*/
public fun cluster(name: String): Cluster {
val builder = ClusterResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy