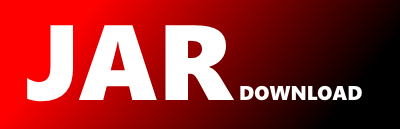
com.pulumi.awsnative.eks.kotlin.EksFunctions.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.eks.kotlin
import com.pulumi.awsnative.eks.EksFunctions.getAccessEntryPlain
import com.pulumi.awsnative.eks.EksFunctions.getAddonPlain
import com.pulumi.awsnative.eks.EksFunctions.getClusterPlain
import com.pulumi.awsnative.eks.EksFunctions.getFargateProfilePlain
import com.pulumi.awsnative.eks.EksFunctions.getIdentityProviderConfigPlain
import com.pulumi.awsnative.eks.EksFunctions.getNodegroupPlain
import com.pulumi.awsnative.eks.EksFunctions.getPodIdentityAssociationPlain
import com.pulumi.awsnative.eks.kotlin.enums.IdentityProviderConfigType
import com.pulumi.awsnative.eks.kotlin.inputs.GetAccessEntryPlainArgs
import com.pulumi.awsnative.eks.kotlin.inputs.GetAccessEntryPlainArgsBuilder
import com.pulumi.awsnative.eks.kotlin.inputs.GetAddonPlainArgs
import com.pulumi.awsnative.eks.kotlin.inputs.GetAddonPlainArgsBuilder
import com.pulumi.awsnative.eks.kotlin.inputs.GetClusterPlainArgs
import com.pulumi.awsnative.eks.kotlin.inputs.GetClusterPlainArgsBuilder
import com.pulumi.awsnative.eks.kotlin.inputs.GetFargateProfilePlainArgs
import com.pulumi.awsnative.eks.kotlin.inputs.GetFargateProfilePlainArgsBuilder
import com.pulumi.awsnative.eks.kotlin.inputs.GetIdentityProviderConfigPlainArgs
import com.pulumi.awsnative.eks.kotlin.inputs.GetIdentityProviderConfigPlainArgsBuilder
import com.pulumi.awsnative.eks.kotlin.inputs.GetNodegroupPlainArgs
import com.pulumi.awsnative.eks.kotlin.inputs.GetNodegroupPlainArgsBuilder
import com.pulumi.awsnative.eks.kotlin.inputs.GetPodIdentityAssociationPlainArgs
import com.pulumi.awsnative.eks.kotlin.inputs.GetPodIdentityAssociationPlainArgsBuilder
import com.pulumi.awsnative.eks.kotlin.outputs.GetAccessEntryResult
import com.pulumi.awsnative.eks.kotlin.outputs.GetAddonResult
import com.pulumi.awsnative.eks.kotlin.outputs.GetClusterResult
import com.pulumi.awsnative.eks.kotlin.outputs.GetFargateProfileResult
import com.pulumi.awsnative.eks.kotlin.outputs.GetIdentityProviderConfigResult
import com.pulumi.awsnative.eks.kotlin.outputs.GetNodegroupResult
import com.pulumi.awsnative.eks.kotlin.outputs.GetPodIdentityAssociationResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.eks.kotlin.outputs.GetAccessEntryResult.Companion.toKotlin as getAccessEntryResultToKotlin
import com.pulumi.awsnative.eks.kotlin.outputs.GetAddonResult.Companion.toKotlin as getAddonResultToKotlin
import com.pulumi.awsnative.eks.kotlin.outputs.GetClusterResult.Companion.toKotlin as getClusterResultToKotlin
import com.pulumi.awsnative.eks.kotlin.outputs.GetFargateProfileResult.Companion.toKotlin as getFargateProfileResultToKotlin
import com.pulumi.awsnative.eks.kotlin.outputs.GetIdentityProviderConfigResult.Companion.toKotlin as getIdentityProviderConfigResultToKotlin
import com.pulumi.awsnative.eks.kotlin.outputs.GetNodegroupResult.Companion.toKotlin as getNodegroupResultToKotlin
import com.pulumi.awsnative.eks.kotlin.outputs.GetPodIdentityAssociationResult.Companion.toKotlin as getPodIdentityAssociationResultToKotlin
public object EksFunctions {
/**
* An object representing an Amazon EKS AccessEntry.
* @param argument null
* @return null
*/
public suspend fun getAccessEntry(argument: GetAccessEntryPlainArgs): GetAccessEntryResult =
getAccessEntryResultToKotlin(getAccessEntryPlain(argument.toJava()).await())
/**
* @see [getAccessEntry].
* @param clusterName The cluster that the access entry is created for.
* @param principalArn The principal ARN that the access entry is created for.
* @return null
*/
public suspend fun getAccessEntry(clusterName: String, principalArn: String): GetAccessEntryResult {
val argument = GetAccessEntryPlainArgs(
clusterName = clusterName,
principalArn = principalArn,
)
return getAccessEntryResultToKotlin(getAccessEntryPlain(argument.toJava()).await())
}
/**
* @see [getAccessEntry].
* @param argument Builder for [com.pulumi.awsnative.eks.kotlin.inputs.GetAccessEntryPlainArgs].
* @return null
*/
public suspend fun getAccessEntry(argument: suspend GetAccessEntryPlainArgsBuilder.() -> Unit): GetAccessEntryResult {
val builder = GetAccessEntryPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAccessEntryResultToKotlin(getAccessEntryPlain(builtArgument.toJava()).await())
}
/**
* Resource Schema for AWS::EKS::Addon
* @param argument null
* @return null
*/
public suspend fun getAddon(argument: GetAddonPlainArgs): GetAddonResult =
getAddonResultToKotlin(getAddonPlain(argument.toJava()).await())
/**
* @see [getAddon].
* @param addonName Name of Addon
* @param clusterName Name of Cluster
* @return null
*/
public suspend fun getAddon(addonName: String, clusterName: String): GetAddonResult {
val argument = GetAddonPlainArgs(
addonName = addonName,
clusterName = clusterName,
)
return getAddonResultToKotlin(getAddonPlain(argument.toJava()).await())
}
/**
* @see [getAddon].
* @param argument Builder for [com.pulumi.awsnative.eks.kotlin.inputs.GetAddonPlainArgs].
* @return null
*/
public suspend fun getAddon(argument: suspend GetAddonPlainArgsBuilder.() -> Unit): GetAddonResult {
val builder = GetAddonPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAddonResultToKotlin(getAddonPlain(builtArgument.toJava()).await())
}
/**
* An object representing an Amazon EKS cluster.
* @param argument null
* @return null
*/
public suspend fun getCluster(argument: GetClusterPlainArgs): GetClusterResult =
getClusterResultToKotlin(getClusterPlain(argument.toJava()).await())
/**
* @see [getCluster].
* @param name The unique name to give to your cluster.
* @return null
*/
public suspend fun getCluster(name: String): GetClusterResult {
val argument = GetClusterPlainArgs(
name = name,
)
return getClusterResultToKotlin(getClusterPlain(argument.toJava()).await())
}
/**
* @see [getCluster].
* @param argument Builder for [com.pulumi.awsnative.eks.kotlin.inputs.GetClusterPlainArgs].
* @return null
*/
public suspend fun getCluster(argument: suspend GetClusterPlainArgsBuilder.() -> Unit): GetClusterResult {
val builder = GetClusterPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getClusterResultToKotlin(getClusterPlain(builtArgument.toJava()).await())
}
/**
* Resource Schema for AWS::EKS::FargateProfile
* @param argument null
* @return null
*/
public suspend fun getFargateProfile(argument: GetFargateProfilePlainArgs): GetFargateProfileResult =
getFargateProfileResultToKotlin(getFargateProfilePlain(argument.toJava()).await())
/**
* @see [getFargateProfile].
* @param clusterName Name of the Cluster
* @param fargateProfileName Name of FargateProfile
* @return null
*/
public suspend fun getFargateProfile(clusterName: String, fargateProfileName: String): GetFargateProfileResult {
val argument = GetFargateProfilePlainArgs(
clusterName = clusterName,
fargateProfileName = fargateProfileName,
)
return getFargateProfileResultToKotlin(getFargateProfilePlain(argument.toJava()).await())
}
/**
* @see [getFargateProfile].
* @param argument Builder for [com.pulumi.awsnative.eks.kotlin.inputs.GetFargateProfilePlainArgs].
* @return null
*/
public suspend fun getFargateProfile(argument: suspend GetFargateProfilePlainArgsBuilder.() -> Unit): GetFargateProfileResult {
val builder = GetFargateProfilePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getFargateProfileResultToKotlin(getFargateProfilePlain(builtArgument.toJava()).await())
}
/**
* An object representing an Amazon EKS IdentityProviderConfig.
* @param argument null
* @return null
*/
public suspend fun getIdentityProviderConfig(argument: GetIdentityProviderConfigPlainArgs): GetIdentityProviderConfigResult =
getIdentityProviderConfigResultToKotlin(getIdentityProviderConfigPlain(argument.toJava()).await())
/**
* @see [getIdentityProviderConfig].
* @param clusterName The name of the identity provider configuration.
* @param identityProviderConfigName The name of the OIDC provider configuration.
* @param type The type of the identity provider configuration.
* @return null
*/
public suspend fun getIdentityProviderConfig(
clusterName: String,
identityProviderConfigName: String,
type: IdentityProviderConfigType,
): GetIdentityProviderConfigResult {
val argument = GetIdentityProviderConfigPlainArgs(
clusterName = clusterName,
identityProviderConfigName = identityProviderConfigName,
type = type,
)
return getIdentityProviderConfigResultToKotlin(getIdentityProviderConfigPlain(argument.toJava()).await())
}
/**
* @see [getIdentityProviderConfig].
* @param argument Builder for [com.pulumi.awsnative.eks.kotlin.inputs.GetIdentityProviderConfigPlainArgs].
* @return null
*/
public suspend fun getIdentityProviderConfig(argument: suspend GetIdentityProviderConfigPlainArgsBuilder.() -> Unit): GetIdentityProviderConfigResult {
val builder = GetIdentityProviderConfigPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getIdentityProviderConfigResultToKotlin(getIdentityProviderConfigPlain(builtArgument.toJava()).await())
}
/**
* Resource schema for AWS::EKS::Nodegroup
* @param argument null
* @return null
*/
public suspend fun getNodegroup(argument: GetNodegroupPlainArgs): GetNodegroupResult =
getNodegroupResultToKotlin(getNodegroupPlain(argument.toJava()).await())
/**
* @see [getNodegroup].
* @param id
* @return null
*/
public suspend fun getNodegroup(id: String): GetNodegroupResult {
val argument = GetNodegroupPlainArgs(
id = id,
)
return getNodegroupResultToKotlin(getNodegroupPlain(argument.toJava()).await())
}
/**
* @see [getNodegroup].
* @param argument Builder for [com.pulumi.awsnative.eks.kotlin.inputs.GetNodegroupPlainArgs].
* @return null
*/
public suspend fun getNodegroup(argument: suspend GetNodegroupPlainArgsBuilder.() -> Unit): GetNodegroupResult {
val builder = GetNodegroupPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getNodegroupResultToKotlin(getNodegroupPlain(builtArgument.toJava()).await())
}
/**
* An object representing an Amazon EKS PodIdentityAssociation.
* @param argument null
* @return null
*/
public suspend fun getPodIdentityAssociation(argument: GetPodIdentityAssociationPlainArgs): GetPodIdentityAssociationResult =
getPodIdentityAssociationResultToKotlin(getPodIdentityAssociationPlain(argument.toJava()).await())
/**
* @see [getPodIdentityAssociation].
* @param associationArn The ARN of the pod identity association.
* @return null
*/
public suspend fun getPodIdentityAssociation(associationArn: String): GetPodIdentityAssociationResult {
val argument = GetPodIdentityAssociationPlainArgs(
associationArn = associationArn,
)
return getPodIdentityAssociationResultToKotlin(getPodIdentityAssociationPlain(argument.toJava()).await())
}
/**
* @see [getPodIdentityAssociation].
* @param argument Builder for [com.pulumi.awsnative.eks.kotlin.inputs.GetPodIdentityAssociationPlainArgs].
* @return null
*/
public suspend fun getPodIdentityAssociation(argument: suspend GetPodIdentityAssociationPlainArgsBuilder.() -> Unit): GetPodIdentityAssociationResult {
val builder = GetPodIdentityAssociationPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getPodIdentityAssociationResultToKotlin(getPodIdentityAssociationPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy