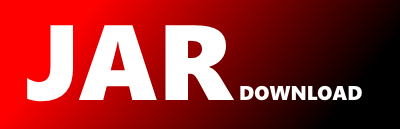
com.pulumi.awsnative.events.kotlin.inputs.RuleBatchParametersArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.events.kotlin.inputs
import com.pulumi.awsnative.events.inputs.RuleBatchParametersArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property arrayProperties The array properties for the submitted job, such as the size of the array. The array size can be between 2 and 10,000. If you specify array properties for a job, it becomes an array job. This parameter is used only if the target is an AWS Batch job.
* @property jobDefinition The ARN or name of the job definition to use if the event target is an AWS Batch job. This job definition must already exist.
* @property jobName The name to use for this execution of the job, if the target is an AWS Batch job.
* @property retryStrategy The retry strategy to use for failed jobs, if the target is an AWS Batch job. The retry strategy is the number of times to retry the failed job execution. Valid values are 1–10. When you specify a retry strategy here, it overrides the retry strategy defined in the job definition.
*/
public data class RuleBatchParametersArgs(
public val arrayProperties: Output? = null,
public val jobDefinition: Output,
public val jobName: Output,
public val retryStrategy: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.events.inputs.RuleBatchParametersArgs =
com.pulumi.awsnative.events.inputs.RuleBatchParametersArgs.builder()
.arrayProperties(arrayProperties?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.jobDefinition(jobDefinition.applyValue({ args0 -> args0 }))
.jobName(jobName.applyValue({ args0 -> args0 }))
.retryStrategy(retryStrategy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [RuleBatchParametersArgs].
*/
@PulumiTagMarker
public class RuleBatchParametersArgsBuilder internal constructor() {
private var arrayProperties: Output? = null
private var jobDefinition: Output? = null
private var jobName: Output? = null
private var retryStrategy: Output? = null
/**
* @param value The array properties for the submitted job, such as the size of the array. The array size can be between 2 and 10,000. If you specify array properties for a job, it becomes an array job. This parameter is used only if the target is an AWS Batch job.
*/
@JvmName("uhneawohgwbaawhm")
public suspend fun arrayProperties(`value`: Output) {
this.arrayProperties = value
}
/**
* @param value The ARN or name of the job definition to use if the event target is an AWS Batch job. This job definition must already exist.
*/
@JvmName("inovynnjwekurjve")
public suspend fun jobDefinition(`value`: Output) {
this.jobDefinition = value
}
/**
* @param value The name to use for this execution of the job, if the target is an AWS Batch job.
*/
@JvmName("jtltrjomnllihmss")
public suspend fun jobName(`value`: Output) {
this.jobName = value
}
/**
* @param value The retry strategy to use for failed jobs, if the target is an AWS Batch job. The retry strategy is the number of times to retry the failed job execution. Valid values are 1–10. When you specify a retry strategy here, it overrides the retry strategy defined in the job definition.
*/
@JvmName("ylptncctrhccvvln")
public suspend fun retryStrategy(`value`: Output) {
this.retryStrategy = value
}
/**
* @param value The array properties for the submitted job, such as the size of the array. The array size can be between 2 and 10,000. If you specify array properties for a job, it becomes an array job. This parameter is used only if the target is an AWS Batch job.
*/
@JvmName("yhomkwfvwfamwktm")
public suspend fun arrayProperties(`value`: RuleBatchArrayPropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.arrayProperties = mapped
}
/**
* @param argument The array properties for the submitted job, such as the size of the array. The array size can be between 2 and 10,000. If you specify array properties for a job, it becomes an array job. This parameter is used only if the target is an AWS Batch job.
*/
@JvmName("dgnifpvghdjoyjoh")
public suspend fun arrayProperties(argument: suspend RuleBatchArrayPropertiesArgsBuilder.() -> Unit) {
val toBeMapped = RuleBatchArrayPropertiesArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.arrayProperties = mapped
}
/**
* @param value The ARN or name of the job definition to use if the event target is an AWS Batch job. This job definition must already exist.
*/
@JvmName("cqtaswpwhgoyrwyv")
public suspend fun jobDefinition(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.jobDefinition = mapped
}
/**
* @param value The name to use for this execution of the job, if the target is an AWS Batch job.
*/
@JvmName("yboicoutpnuatibj")
public suspend fun jobName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.jobName = mapped
}
/**
* @param value The retry strategy to use for failed jobs, if the target is an AWS Batch job. The retry strategy is the number of times to retry the failed job execution. Valid values are 1–10. When you specify a retry strategy here, it overrides the retry strategy defined in the job definition.
*/
@JvmName("nymxqhmdcncnksyx")
public suspend fun retryStrategy(`value`: RuleBatchRetryStrategyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.retryStrategy = mapped
}
/**
* @param argument The retry strategy to use for failed jobs, if the target is an AWS Batch job. The retry strategy is the number of times to retry the failed job execution. Valid values are 1–10. When you specify a retry strategy here, it overrides the retry strategy defined in the job definition.
*/
@JvmName("lwmmolgbfxxtfqir")
public suspend fun retryStrategy(argument: suspend RuleBatchRetryStrategyArgsBuilder.() -> Unit) {
val toBeMapped = RuleBatchRetryStrategyArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.retryStrategy = mapped
}
internal fun build(): RuleBatchParametersArgs = RuleBatchParametersArgs(
arrayProperties = arrayProperties,
jobDefinition = jobDefinition ?: throw PulumiNullFieldException("jobDefinition"),
jobName = jobName ?: throw PulumiNullFieldException("jobName"),
retryStrategy = retryStrategy,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy