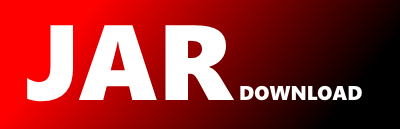
com.pulumi.awsnative.evidently.kotlin.EvidentlyFunctions.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.evidently.kotlin
import com.pulumi.awsnative.evidently.EvidentlyFunctions.getExperimentPlain
import com.pulumi.awsnative.evidently.EvidentlyFunctions.getFeaturePlain
import com.pulumi.awsnative.evidently.EvidentlyFunctions.getLaunchPlain
import com.pulumi.awsnative.evidently.EvidentlyFunctions.getProjectPlain
import com.pulumi.awsnative.evidently.EvidentlyFunctions.getSegmentPlain
import com.pulumi.awsnative.evidently.kotlin.inputs.GetExperimentPlainArgs
import com.pulumi.awsnative.evidently.kotlin.inputs.GetExperimentPlainArgsBuilder
import com.pulumi.awsnative.evidently.kotlin.inputs.GetFeaturePlainArgs
import com.pulumi.awsnative.evidently.kotlin.inputs.GetFeaturePlainArgsBuilder
import com.pulumi.awsnative.evidently.kotlin.inputs.GetLaunchPlainArgs
import com.pulumi.awsnative.evidently.kotlin.inputs.GetLaunchPlainArgsBuilder
import com.pulumi.awsnative.evidently.kotlin.inputs.GetProjectPlainArgs
import com.pulumi.awsnative.evidently.kotlin.inputs.GetProjectPlainArgsBuilder
import com.pulumi.awsnative.evidently.kotlin.inputs.GetSegmentPlainArgs
import com.pulumi.awsnative.evidently.kotlin.inputs.GetSegmentPlainArgsBuilder
import com.pulumi.awsnative.evidently.kotlin.outputs.GetExperimentResult
import com.pulumi.awsnative.evidently.kotlin.outputs.GetFeatureResult
import com.pulumi.awsnative.evidently.kotlin.outputs.GetLaunchResult
import com.pulumi.awsnative.evidently.kotlin.outputs.GetProjectResult
import com.pulumi.awsnative.evidently.kotlin.outputs.GetSegmentResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.evidently.kotlin.outputs.GetExperimentResult.Companion.toKotlin as getExperimentResultToKotlin
import com.pulumi.awsnative.evidently.kotlin.outputs.GetFeatureResult.Companion.toKotlin as getFeatureResultToKotlin
import com.pulumi.awsnative.evidently.kotlin.outputs.GetLaunchResult.Companion.toKotlin as getLaunchResultToKotlin
import com.pulumi.awsnative.evidently.kotlin.outputs.GetProjectResult.Companion.toKotlin as getProjectResultToKotlin
import com.pulumi.awsnative.evidently.kotlin.outputs.GetSegmentResult.Companion.toKotlin as getSegmentResultToKotlin
public object EvidentlyFunctions {
/**
* Resource Type definition for AWS::Evidently::Experiment.
* @param argument null
* @return null
*/
public suspend fun getExperiment(argument: GetExperimentPlainArgs): GetExperimentResult =
getExperimentResultToKotlin(getExperimentPlain(argument.toJava()).await())
/**
* @see [getExperiment].
* @param arn The ARN of the experiment. For example, `arn:aws:evidently:us-west-2:0123455678912:project/myProject/experiment/myExperiment`
* @return null
*/
public suspend fun getExperiment(arn: String): GetExperimentResult {
val argument = GetExperimentPlainArgs(
arn = arn,
)
return getExperimentResultToKotlin(getExperimentPlain(argument.toJava()).await())
}
/**
* @see [getExperiment].
* @param argument Builder for [com.pulumi.awsnative.evidently.kotlin.inputs.GetExperimentPlainArgs].
* @return null
*/
public suspend fun getExperiment(argument: suspend GetExperimentPlainArgsBuilder.() -> Unit): GetExperimentResult {
val builder = GetExperimentPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getExperimentResultToKotlin(getExperimentPlain(builtArgument.toJava()).await())
}
/**
* Resource Type definition for AWS::Evidently::Feature.
* @param argument null
* @return null
*/
public suspend fun getFeature(argument: GetFeaturePlainArgs): GetFeatureResult =
getFeatureResultToKotlin(getFeaturePlain(argument.toJava()).await())
/**
* @see [getFeature].
* @param arn The ARN of the feature. For example, `arn:aws:evidently:us-west-2:0123455678912:project/myProject/feature/myFeature` .
* @return null
*/
public suspend fun getFeature(arn: String): GetFeatureResult {
val argument = GetFeaturePlainArgs(
arn = arn,
)
return getFeatureResultToKotlin(getFeaturePlain(argument.toJava()).await())
}
/**
* @see [getFeature].
* @param argument Builder for [com.pulumi.awsnative.evidently.kotlin.inputs.GetFeaturePlainArgs].
* @return null
*/
public suspend fun getFeature(argument: suspend GetFeaturePlainArgsBuilder.() -> Unit): GetFeatureResult {
val builder = GetFeaturePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getFeatureResultToKotlin(getFeaturePlain(builtArgument.toJava()).await())
}
/**
* Resource Type definition for AWS::Evidently::Launch.
* @param argument null
* @return null
*/
public suspend fun getLaunch(argument: GetLaunchPlainArgs): GetLaunchResult =
getLaunchResultToKotlin(getLaunchPlain(argument.toJava()).await())
/**
* @see [getLaunch].
* @param arn The ARN of the launch. For example, `arn:aws:evidently:us-west-2:0123455678912:project/myProject/launch/myLaunch`
* @return null
*/
public suspend fun getLaunch(arn: String): GetLaunchResult {
val argument = GetLaunchPlainArgs(
arn = arn,
)
return getLaunchResultToKotlin(getLaunchPlain(argument.toJava()).await())
}
/**
* @see [getLaunch].
* @param argument Builder for [com.pulumi.awsnative.evidently.kotlin.inputs.GetLaunchPlainArgs].
* @return null
*/
public suspend fun getLaunch(argument: suspend GetLaunchPlainArgsBuilder.() -> Unit): GetLaunchResult {
val builder = GetLaunchPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getLaunchResultToKotlin(getLaunchPlain(builtArgument.toJava()).await())
}
/**
* Resource Type definition for AWS::Evidently::Project
* @param argument null
* @return null
*/
public suspend fun getProject(argument: GetProjectPlainArgs): GetProjectResult =
getProjectResultToKotlin(getProjectPlain(argument.toJava()).await())
/**
* @see [getProject].
* @param arn The ARN of the project. For example, `arn:aws:evidently:us-west-2:0123455678912:project/myProject`
* @return null
*/
public suspend fun getProject(arn: String): GetProjectResult {
val argument = GetProjectPlainArgs(
arn = arn,
)
return getProjectResultToKotlin(getProjectPlain(argument.toJava()).await())
}
/**
* @see [getProject].
* @param argument Builder for [com.pulumi.awsnative.evidently.kotlin.inputs.GetProjectPlainArgs].
* @return null
*/
public suspend fun getProject(argument: suspend GetProjectPlainArgsBuilder.() -> Unit): GetProjectResult {
val builder = GetProjectPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getProjectResultToKotlin(getProjectPlain(builtArgument.toJava()).await())
}
/**
* Resource Type definition for AWS::Evidently::Segment
* @param argument null
* @return null
*/
public suspend fun getSegment(argument: GetSegmentPlainArgs): GetSegmentResult =
getSegmentResultToKotlin(getSegmentPlain(argument.toJava()).await())
/**
* @see [getSegment].
* @param arn The ARN of the segment. For example, `arn:aws:evidently:us-west-2:123456789012:segment/australiaSegment`
* @return null
*/
public suspend fun getSegment(arn: String): GetSegmentResult {
val argument = GetSegmentPlainArgs(
arn = arn,
)
return getSegmentResultToKotlin(getSegmentPlain(argument.toJava()).await())
}
/**
* @see [getSegment].
* @param argument Builder for [com.pulumi.awsnative.evidently.kotlin.inputs.GetSegmentPlainArgs].
* @return null
*/
public suspend fun getSegment(argument: suspend GetSegmentPlainArgsBuilder.() -> Unit): GetSegmentResult {
val builder = GetSegmentPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getSegmentResultToKotlin(getSegmentPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy