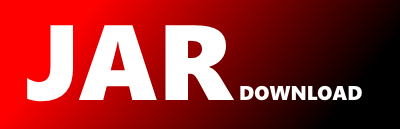
com.pulumi.awsnative.fis.kotlin.ExperimentTemplateArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.fis.kotlin
import com.pulumi.awsnative.fis.ExperimentTemplateArgs.builder
import com.pulumi.awsnative.fis.kotlin.inputs.ExperimentTemplateActionArgs
import com.pulumi.awsnative.fis.kotlin.inputs.ExperimentTemplateActionArgsBuilder
import com.pulumi.awsnative.fis.kotlin.inputs.ExperimentTemplateExperimentOptionsArgs
import com.pulumi.awsnative.fis.kotlin.inputs.ExperimentTemplateExperimentOptionsArgsBuilder
import com.pulumi.awsnative.fis.kotlin.inputs.ExperimentTemplateLogConfigurationArgs
import com.pulumi.awsnative.fis.kotlin.inputs.ExperimentTemplateLogConfigurationArgsBuilder
import com.pulumi.awsnative.fis.kotlin.inputs.ExperimentTemplateStopConditionArgs
import com.pulumi.awsnative.fis.kotlin.inputs.ExperimentTemplateStopConditionArgsBuilder
import com.pulumi.awsnative.fis.kotlin.inputs.ExperimentTemplateTargetArgs
import com.pulumi.awsnative.fis.kotlin.inputs.ExperimentTemplateTargetArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Resource schema for AWS::FIS::ExperimentTemplate
* ## Example Usage
* ### Example
* No Java example available.
* @property actions The actions for the experiment.
* @property description The description for the experiment template.
* @property experimentOptions The experiment options for an experiment template.
* @property logConfiguration The configuration for experiment logging.
* @property roleArn The Amazon Resource Name (ARN) of an IAM role.
* @property stopConditions The stop conditions for the experiment.
* @property tags The tags for the experiment template.
* @property targets The targets for the experiment.
*/
public data class ExperimentTemplateArgs(
public val actions: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy