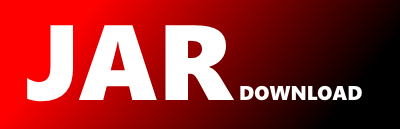
com.pulumi.awsnative.fis.kotlin.FisFunctions.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.fis.kotlin
import com.pulumi.awsnative.fis.FisFunctions.getExperimentTemplatePlain
import com.pulumi.awsnative.fis.FisFunctions.getTargetAccountConfigurationPlain
import com.pulumi.awsnative.fis.kotlin.inputs.GetExperimentTemplatePlainArgs
import com.pulumi.awsnative.fis.kotlin.inputs.GetExperimentTemplatePlainArgsBuilder
import com.pulumi.awsnative.fis.kotlin.inputs.GetTargetAccountConfigurationPlainArgs
import com.pulumi.awsnative.fis.kotlin.inputs.GetTargetAccountConfigurationPlainArgsBuilder
import com.pulumi.awsnative.fis.kotlin.outputs.GetExperimentTemplateResult
import com.pulumi.awsnative.fis.kotlin.outputs.GetTargetAccountConfigurationResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.fis.kotlin.outputs.GetExperimentTemplateResult.Companion.toKotlin as getExperimentTemplateResultToKotlin
import com.pulumi.awsnative.fis.kotlin.outputs.GetTargetAccountConfigurationResult.Companion.toKotlin as getTargetAccountConfigurationResultToKotlin
public object FisFunctions {
/**
* Resource schema for AWS::FIS::ExperimentTemplate
* @param argument null
* @return null
*/
public suspend fun getExperimentTemplate(argument: GetExperimentTemplatePlainArgs): GetExperimentTemplateResult =
getExperimentTemplateResultToKotlin(getExperimentTemplatePlain(argument.toJava()).await())
/**
* @see [getExperimentTemplate].
* @param id The ID of the experiment template.
* @return null
*/
public suspend fun getExperimentTemplate(id: String): GetExperimentTemplateResult {
val argument = GetExperimentTemplatePlainArgs(
id = id,
)
return getExperimentTemplateResultToKotlin(getExperimentTemplatePlain(argument.toJava()).await())
}
/**
* @see [getExperimentTemplate].
* @param argument Builder for [com.pulumi.awsnative.fis.kotlin.inputs.GetExperimentTemplatePlainArgs].
* @return null
*/
public suspend fun getExperimentTemplate(argument: suspend GetExperimentTemplatePlainArgsBuilder.() -> Unit): GetExperimentTemplateResult {
val builder = GetExperimentTemplatePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getExperimentTemplateResultToKotlin(getExperimentTemplatePlain(builtArgument.toJava()).await())
}
/**
* Resource schema for AWS::FIS::TargetAccountConfiguration
* @param argument null
* @return null
*/
public suspend fun getTargetAccountConfiguration(argument: GetTargetAccountConfigurationPlainArgs): GetTargetAccountConfigurationResult =
getTargetAccountConfigurationResultToKotlin(getTargetAccountConfigurationPlain(argument.toJava()).await())
/**
* @see [getTargetAccountConfiguration].
* @param accountId The AWS account ID of the target account.
* @param experimentTemplateId The ID of the experiment template.
* @return null
*/
public suspend fun getTargetAccountConfiguration(accountId: String, experimentTemplateId: String): GetTargetAccountConfigurationResult {
val argument = GetTargetAccountConfigurationPlainArgs(
accountId = accountId,
experimentTemplateId = experimentTemplateId,
)
return getTargetAccountConfigurationResultToKotlin(getTargetAccountConfigurationPlain(argument.toJava()).await())
}
/**
* @see [getTargetAccountConfiguration].
* @param argument Builder for [com.pulumi.awsnative.fis.kotlin.inputs.GetTargetAccountConfigurationPlainArgs].
* @return null
*/
public suspend fun getTargetAccountConfiguration(argument: suspend GetTargetAccountConfigurationPlainArgsBuilder.() -> Unit): GetTargetAccountConfigurationResult {
val builder = GetTargetAccountConfigurationPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getTargetAccountConfigurationResultToKotlin(getTargetAccountConfigurationPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy