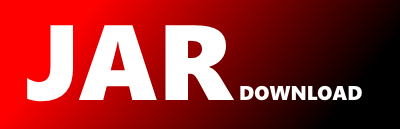
com.pulumi.awsnative.frauddetector.kotlin.FrauddetectorFunctions.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.frauddetector.kotlin
import com.pulumi.awsnative.frauddetector.FrauddetectorFunctions.getDetectorPlain
import com.pulumi.awsnative.frauddetector.FrauddetectorFunctions.getEntityTypePlain
import com.pulumi.awsnative.frauddetector.FrauddetectorFunctions.getEventTypePlain
import com.pulumi.awsnative.frauddetector.FrauddetectorFunctions.getLabelPlain
import com.pulumi.awsnative.frauddetector.FrauddetectorFunctions.getListPlain
import com.pulumi.awsnative.frauddetector.FrauddetectorFunctions.getOutcomePlain
import com.pulumi.awsnative.frauddetector.FrauddetectorFunctions.getVariablePlain
import com.pulumi.awsnative.frauddetector.kotlin.inputs.GetDetectorPlainArgs
import com.pulumi.awsnative.frauddetector.kotlin.inputs.GetDetectorPlainArgsBuilder
import com.pulumi.awsnative.frauddetector.kotlin.inputs.GetEntityTypePlainArgs
import com.pulumi.awsnative.frauddetector.kotlin.inputs.GetEntityTypePlainArgsBuilder
import com.pulumi.awsnative.frauddetector.kotlin.inputs.GetEventTypePlainArgs
import com.pulumi.awsnative.frauddetector.kotlin.inputs.GetEventTypePlainArgsBuilder
import com.pulumi.awsnative.frauddetector.kotlin.inputs.GetLabelPlainArgs
import com.pulumi.awsnative.frauddetector.kotlin.inputs.GetLabelPlainArgsBuilder
import com.pulumi.awsnative.frauddetector.kotlin.inputs.GetListPlainArgs
import com.pulumi.awsnative.frauddetector.kotlin.inputs.GetListPlainArgsBuilder
import com.pulumi.awsnative.frauddetector.kotlin.inputs.GetOutcomePlainArgs
import com.pulumi.awsnative.frauddetector.kotlin.inputs.GetOutcomePlainArgsBuilder
import com.pulumi.awsnative.frauddetector.kotlin.inputs.GetVariablePlainArgs
import com.pulumi.awsnative.frauddetector.kotlin.inputs.GetVariablePlainArgsBuilder
import com.pulumi.awsnative.frauddetector.kotlin.outputs.GetDetectorResult
import com.pulumi.awsnative.frauddetector.kotlin.outputs.GetEntityTypeResult
import com.pulumi.awsnative.frauddetector.kotlin.outputs.GetEventTypeResult
import com.pulumi.awsnative.frauddetector.kotlin.outputs.GetLabelResult
import com.pulumi.awsnative.frauddetector.kotlin.outputs.GetListResult
import com.pulumi.awsnative.frauddetector.kotlin.outputs.GetOutcomeResult
import com.pulumi.awsnative.frauddetector.kotlin.outputs.GetVariableResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.frauddetector.kotlin.outputs.GetDetectorResult.Companion.toKotlin as getDetectorResultToKotlin
import com.pulumi.awsnative.frauddetector.kotlin.outputs.GetEntityTypeResult.Companion.toKotlin as getEntityTypeResultToKotlin
import com.pulumi.awsnative.frauddetector.kotlin.outputs.GetEventTypeResult.Companion.toKotlin as getEventTypeResultToKotlin
import com.pulumi.awsnative.frauddetector.kotlin.outputs.GetLabelResult.Companion.toKotlin as getLabelResultToKotlin
import com.pulumi.awsnative.frauddetector.kotlin.outputs.GetListResult.Companion.toKotlin as getListResultToKotlin
import com.pulumi.awsnative.frauddetector.kotlin.outputs.GetOutcomeResult.Companion.toKotlin as getOutcomeResultToKotlin
import com.pulumi.awsnative.frauddetector.kotlin.outputs.GetVariableResult.Companion.toKotlin as getVariableResultToKotlin
public object FrauddetectorFunctions {
/**
* A resource schema for a Detector in Amazon Fraud Detector.
* @param argument null
* @return null
*/
public suspend fun getDetector(argument: GetDetectorPlainArgs): GetDetectorResult =
getDetectorResultToKotlin(getDetectorPlain(argument.toJava()).await())
/**
* @see [getDetector].
* @param arn The ARN of the detector.
* @return null
*/
public suspend fun getDetector(arn: String): GetDetectorResult {
val argument = GetDetectorPlainArgs(
arn = arn,
)
return getDetectorResultToKotlin(getDetectorPlain(argument.toJava()).await())
}
/**
* @see [getDetector].
* @param argument Builder for [com.pulumi.awsnative.frauddetector.kotlin.inputs.GetDetectorPlainArgs].
* @return null
*/
public suspend fun getDetector(argument: suspend GetDetectorPlainArgsBuilder.() -> Unit): GetDetectorResult {
val builder = GetDetectorPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getDetectorResultToKotlin(getDetectorPlain(builtArgument.toJava()).await())
}
/**
* An entity type for fraud detector.
* @param argument null
* @return null
*/
public suspend fun getEntityType(argument: GetEntityTypePlainArgs): GetEntityTypeResult =
getEntityTypeResultToKotlin(getEntityTypePlain(argument.toJava()).await())
/**
* @see [getEntityType].
* @param arn The entity type ARN.
* @return null
*/
public suspend fun getEntityType(arn: String): GetEntityTypeResult {
val argument = GetEntityTypePlainArgs(
arn = arn,
)
return getEntityTypeResultToKotlin(getEntityTypePlain(argument.toJava()).await())
}
/**
* @see [getEntityType].
* @param argument Builder for [com.pulumi.awsnative.frauddetector.kotlin.inputs.GetEntityTypePlainArgs].
* @return null
*/
public suspend fun getEntityType(argument: suspend GetEntityTypePlainArgsBuilder.() -> Unit): GetEntityTypeResult {
val builder = GetEntityTypePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getEntityTypeResultToKotlin(getEntityTypePlain(builtArgument.toJava()).await())
}
/**
* A resource schema for an EventType in Amazon Fraud Detector.
* @param argument null
* @return null
*/
public suspend fun getEventType(argument: GetEventTypePlainArgs): GetEventTypeResult =
getEventTypeResultToKotlin(getEventTypePlain(argument.toJava()).await())
/**
* @see [getEventType].
* @param arn The ARN of the event type.
* @return null
*/
public suspend fun getEventType(arn: String): GetEventTypeResult {
val argument = GetEventTypePlainArgs(
arn = arn,
)
return getEventTypeResultToKotlin(getEventTypePlain(argument.toJava()).await())
}
/**
* @see [getEventType].
* @param argument Builder for [com.pulumi.awsnative.frauddetector.kotlin.inputs.GetEventTypePlainArgs].
* @return null
*/
public suspend fun getEventType(argument: suspend GetEventTypePlainArgsBuilder.() -> Unit): GetEventTypeResult {
val builder = GetEventTypePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getEventTypeResultToKotlin(getEventTypePlain(builtArgument.toJava()).await())
}
/**
* An label for fraud detector.
* @param argument null
* @return null
*/
public suspend fun getLabel(argument: GetLabelPlainArgs): GetLabelResult =
getLabelResultToKotlin(getLabelPlain(argument.toJava()).await())
/**
* @see [getLabel].
* @param arn The label ARN.
* @return null
*/
public suspend fun getLabel(arn: String): GetLabelResult {
val argument = GetLabelPlainArgs(
arn = arn,
)
return getLabelResultToKotlin(getLabelPlain(argument.toJava()).await())
}
/**
* @see [getLabel].
* @param argument Builder for [com.pulumi.awsnative.frauddetector.kotlin.inputs.GetLabelPlainArgs].
* @return null
*/
public suspend fun getLabel(argument: suspend GetLabelPlainArgsBuilder.() -> Unit): GetLabelResult {
val builder = GetLabelPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getLabelResultToKotlin(getLabelPlain(builtArgument.toJava()).await())
}
/**
* A resource schema for a List in Amazon Fraud Detector.
* @param argument null
* @return null
*/
public suspend fun getList(argument: GetListPlainArgs): GetListResult =
getListResultToKotlin(getListPlain(argument.toJava()).await())
/**
* @see [getList].
* @param arn The list ARN.
* @return null
*/
public suspend fun getList(arn: String): GetListResult {
val argument = GetListPlainArgs(
arn = arn,
)
return getListResultToKotlin(getListPlain(argument.toJava()).await())
}
/**
* @see [getList].
* @param argument Builder for [com.pulumi.awsnative.frauddetector.kotlin.inputs.GetListPlainArgs].
* @return null
*/
public suspend fun getList(argument: suspend GetListPlainArgsBuilder.() -> Unit): GetListResult {
val builder = GetListPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getListResultToKotlin(getListPlain(builtArgument.toJava()).await())
}
/**
* An outcome for rule evaluation.
* @param argument null
* @return null
*/
public suspend fun getOutcome(argument: GetOutcomePlainArgs): GetOutcomeResult =
getOutcomeResultToKotlin(getOutcomePlain(argument.toJava()).await())
/**
* @see [getOutcome].
* @param arn The outcome ARN.
* @return null
*/
public suspend fun getOutcome(arn: String): GetOutcomeResult {
val argument = GetOutcomePlainArgs(
arn = arn,
)
return getOutcomeResultToKotlin(getOutcomePlain(argument.toJava()).await())
}
/**
* @see [getOutcome].
* @param argument Builder for [com.pulumi.awsnative.frauddetector.kotlin.inputs.GetOutcomePlainArgs].
* @return null
*/
public suspend fun getOutcome(argument: suspend GetOutcomePlainArgsBuilder.() -> Unit): GetOutcomeResult {
val builder = GetOutcomePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getOutcomeResultToKotlin(getOutcomePlain(builtArgument.toJava()).await())
}
/**
* A resource schema for a Variable in Amazon Fraud Detector.
* @param argument null
* @return null
*/
public suspend fun getVariable(argument: GetVariablePlainArgs): GetVariableResult =
getVariableResultToKotlin(getVariablePlain(argument.toJava()).await())
/**
* @see [getVariable].
* @param arn The ARN of the variable.
* @return null
*/
public suspend fun getVariable(arn: String): GetVariableResult {
val argument = GetVariablePlainArgs(
arn = arn,
)
return getVariableResultToKotlin(getVariablePlain(argument.toJava()).await())
}
/**
* @see [getVariable].
* @param argument Builder for [com.pulumi.awsnative.frauddetector.kotlin.inputs.GetVariablePlainArgs].
* @return null
*/
public suspend fun getVariable(argument: suspend GetVariablePlainArgsBuilder.() -> Unit): GetVariableResult {
val builder = GetVariablePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getVariableResultToKotlin(getVariablePlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy