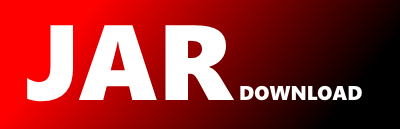
com.pulumi.awsnative.gamelift.kotlin.ContainerGroupDefinitionArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.gamelift.kotlin
import com.pulumi.awsnative.gamelift.ContainerGroupDefinitionArgs.builder
import com.pulumi.awsnative.gamelift.kotlin.enums.ContainerGroupDefinitionOperatingSystem
import com.pulumi.awsnative.gamelift.kotlin.enums.ContainerGroupDefinitionSchedulingStrategy
import com.pulumi.awsnative.gamelift.kotlin.inputs.ContainerGroupDefinitionContainerDefinitionArgs
import com.pulumi.awsnative.gamelift.kotlin.inputs.ContainerGroupDefinitionContainerDefinitionArgsBuilder
import com.pulumi.awsnative.kotlin.inputs.TagArgs
import com.pulumi.awsnative.kotlin.inputs.TagArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* The AWS::GameLift::ContainerGroupDefinition resource creates an Amazon GameLift container group definition.
* @property containerDefinitions A collection of container definitions that define the containers in this group.
* @property name A descriptive label for the container group definition.
* @property operatingSystem The operating system of the container group
* @property schedulingStrategy Specifies whether the container group includes replica or daemon containers.
* @property tags An array of key-value pairs to apply to this resource.
* @property totalCpuLimit The maximum number of CPU units reserved for this container group. The value is expressed as an integer amount of CPU units. (1 vCPU is equal to 1024 CPU units.)
* @property totalMemoryLimit The maximum amount of memory (in MiB) to allocate for this container group.
*/
public data class ContainerGroupDefinitionArgs(
public val containerDefinitions: Output>? =
null,
public val name: Output? = null,
public val operatingSystem: Output? = null,
public val schedulingStrategy: Output? = null,
public val tags: Output>? = null,
public val totalCpuLimit: Output? = null,
public val totalMemoryLimit: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.gamelift.ContainerGroupDefinitionArgs =
com.pulumi.awsnative.gamelift.ContainerGroupDefinitionArgs.builder()
.containerDefinitions(
containerDefinitions?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.name(name?.applyValue({ args0 -> args0 }))
.operatingSystem(operatingSystem?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.schedulingStrategy(
schedulingStrategy?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.tags(tags?.applyValue({ args0 -> args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) }) }))
.totalCpuLimit(totalCpuLimit?.applyValue({ args0 -> args0 }))
.totalMemoryLimit(totalMemoryLimit?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ContainerGroupDefinitionArgs].
*/
@PulumiTagMarker
public class ContainerGroupDefinitionArgsBuilder internal constructor() {
private var containerDefinitions: Output>? =
null
private var name: Output? = null
private var operatingSystem: Output? = null
private var schedulingStrategy: Output? = null
private var tags: Output>? = null
private var totalCpuLimit: Output? = null
private var totalMemoryLimit: Output? = null
/**
* @param value A collection of container definitions that define the containers in this group.
*/
@JvmName("oqgecmqvjqftxmni")
public suspend fun containerDefinitions(`value`: Output>) {
this.containerDefinitions = value
}
@JvmName("kyqrfmboxlrnjfvo")
public suspend fun containerDefinitions(vararg values: Output) {
this.containerDefinitions = Output.all(values.asList())
}
/**
* @param values A collection of container definitions that define the containers in this group.
*/
@JvmName("pcaohyibrmtrhanm")
public suspend fun containerDefinitions(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy