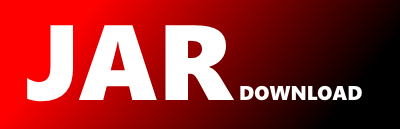
com.pulumi.awsnative.gamelift.kotlin.inputs.FleetLocationCapacityArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.gamelift.kotlin.inputs
import com.pulumi.awsnative.gamelift.inputs.FleetLocationCapacityArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Current resource capacity settings in a specified fleet or location. The location value might refer to a fleet's remote location or its home Region.
* @property desiredEc2Instances The number of EC2 instances you want to maintain in the specified fleet location. This value must fall between the minimum and maximum size limits.
* @property maxSize The maximum value that is allowed for the fleet's instance count for a location. When creating a new fleet, GameLift automatically sets this value to "1". Once the fleet is active, you can change this value.
* @property minSize The minimum value allowed for the fleet's instance count for a location. When creating a new fleet, GameLift automatically sets this value to "0". After the fleet is active, you can change this value.
*/
public data class FleetLocationCapacityArgs(
public val desiredEc2Instances: Output,
public val maxSize: Output,
public val minSize: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.gamelift.inputs.FleetLocationCapacityArgs =
com.pulumi.awsnative.gamelift.inputs.FleetLocationCapacityArgs.builder()
.desiredEc2Instances(desiredEc2Instances.applyValue({ args0 -> args0 }))
.maxSize(maxSize.applyValue({ args0 -> args0 }))
.minSize(minSize.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FleetLocationCapacityArgs].
*/
@PulumiTagMarker
public class FleetLocationCapacityArgsBuilder internal constructor() {
private var desiredEc2Instances: Output? = null
private var maxSize: Output? = null
private var minSize: Output? = null
/**
* @param value The number of EC2 instances you want to maintain in the specified fleet location. This value must fall between the minimum and maximum size limits.
*/
@JvmName("ldrwebosepmnkirx")
public suspend fun desiredEc2Instances(`value`: Output) {
this.desiredEc2Instances = value
}
/**
* @param value The maximum value that is allowed for the fleet's instance count for a location. When creating a new fleet, GameLift automatically sets this value to "1". Once the fleet is active, you can change this value.
*/
@JvmName("vdjrdcqyhpoambhv")
public suspend fun maxSize(`value`: Output) {
this.maxSize = value
}
/**
* @param value The minimum value allowed for the fleet's instance count for a location. When creating a new fleet, GameLift automatically sets this value to "0". After the fleet is active, you can change this value.
*/
@JvmName("ulpgpgtxeugpqblo")
public suspend fun minSize(`value`: Output) {
this.minSize = value
}
/**
* @param value The number of EC2 instances you want to maintain in the specified fleet location. This value must fall between the minimum and maximum size limits.
*/
@JvmName("iqceciomhmdcnkvh")
public suspend fun desiredEc2Instances(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.desiredEc2Instances = mapped
}
/**
* @param value The maximum value that is allowed for the fleet's instance count for a location. When creating a new fleet, GameLift automatically sets this value to "1". Once the fleet is active, you can change this value.
*/
@JvmName("tncyogfdruymvytr")
public suspend fun maxSize(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.maxSize = mapped
}
/**
* @param value The minimum value allowed for the fleet's instance count for a location. When creating a new fleet, GameLift automatically sets this value to "0". After the fleet is active, you can change this value.
*/
@JvmName("cncjtwprqutigbge")
public suspend fun minSize(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.minSize = mapped
}
internal fun build(): FleetLocationCapacityArgs = FleetLocationCapacityArgs(
desiredEc2Instances = desiredEc2Instances ?: throw PulumiNullFieldException("desiredEc2Instances"),
maxSize = maxSize ?: throw PulumiNullFieldException("maxSize"),
minSize = minSize ?: throw PulumiNullFieldException("minSize"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy