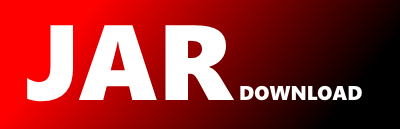
com.pulumi.awsnative.glue.kotlin.inputs.TriggerConditionArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.glue.kotlin.inputs
import com.pulumi.awsnative.glue.inputs.TriggerConditionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Defines a condition under which a trigger fires.
* @property crawlState The state of the crawler to which this condition applies.
* @property crawlerName The name of the crawler to which this condition applies.
* @property jobName The name of the job whose JobRuns this condition applies to, and on which this trigger waits.
* @property logicalOperator A logical operator.
* @property state The condition state. Currently, the values supported are SUCCEEDED, STOPPED, TIMEOUT, and FAILED.
*/
public data class TriggerConditionArgs(
public val crawlState: Output? = null,
public val crawlerName: Output? = null,
public val jobName: Output? = null,
public val logicalOperator: Output? = null,
public val state: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.glue.inputs.TriggerConditionArgs =
com.pulumi.awsnative.glue.inputs.TriggerConditionArgs.builder()
.crawlState(crawlState?.applyValue({ args0 -> args0 }))
.crawlerName(crawlerName?.applyValue({ args0 -> args0 }))
.jobName(jobName?.applyValue({ args0 -> args0 }))
.logicalOperator(logicalOperator?.applyValue({ args0 -> args0 }))
.state(state?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [TriggerConditionArgs].
*/
@PulumiTagMarker
public class TriggerConditionArgsBuilder internal constructor() {
private var crawlState: Output? = null
private var crawlerName: Output? = null
private var jobName: Output? = null
private var logicalOperator: Output? = null
private var state: Output? = null
/**
* @param value The state of the crawler to which this condition applies.
*/
@JvmName("aovxtbbkmfsiirhh")
public suspend fun crawlState(`value`: Output) {
this.crawlState = value
}
/**
* @param value The name of the crawler to which this condition applies.
*/
@JvmName("nomgyqjlxyakjdww")
public suspend fun crawlerName(`value`: Output) {
this.crawlerName = value
}
/**
* @param value The name of the job whose JobRuns this condition applies to, and on which this trigger waits.
*/
@JvmName("qxymeagpwhdwhnoq")
public suspend fun jobName(`value`: Output) {
this.jobName = value
}
/**
* @param value A logical operator.
*/
@JvmName("wyldserpjkhkxxpp")
public suspend fun logicalOperator(`value`: Output) {
this.logicalOperator = value
}
/**
* @param value The condition state. Currently, the values supported are SUCCEEDED, STOPPED, TIMEOUT, and FAILED.
*/
@JvmName("phvftxehtwnovgoj")
public suspend fun state(`value`: Output) {
this.state = value
}
/**
* @param value The state of the crawler to which this condition applies.
*/
@JvmName("nlunckmcpshkqkeu")
public suspend fun crawlState(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.crawlState = mapped
}
/**
* @param value The name of the crawler to which this condition applies.
*/
@JvmName("oanmuhikoecctqvy")
public suspend fun crawlerName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.crawlerName = mapped
}
/**
* @param value The name of the job whose JobRuns this condition applies to, and on which this trigger waits.
*/
@JvmName("guclewjnntdswvck")
public suspend fun jobName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.jobName = mapped
}
/**
* @param value A logical operator.
*/
@JvmName("oinrhqorlptfgnbj")
public suspend fun logicalOperator(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.logicalOperator = mapped
}
/**
* @param value The condition state. Currently, the values supported are SUCCEEDED, STOPPED, TIMEOUT, and FAILED.
*/
@JvmName("lqfimtqmfvqhwkdm")
public suspend fun state(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.state = mapped
}
internal fun build(): TriggerConditionArgs = TriggerConditionArgs(
crawlState = crawlState,
crawlerName = crawlerName,
jobName = jobName,
logicalOperator = logicalOperator,
state = state,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy