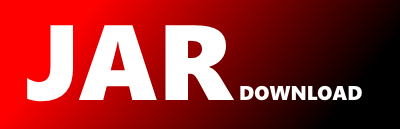
com.pulumi.awsnative.groundstation.kotlin.inputs.DataflowEndpointGroupDataflowEndpointArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.groundstation.kotlin.inputs
import com.pulumi.awsnative.groundstation.inputs.DataflowEndpointGroupDataflowEndpointArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property address The address and port of an endpoint.
* @property mtu Maximum transmission unit (MTU) size in bytes of a dataflow endpoint. Valid values are between 1400 and 1500. A default value of 1500 is used if not set.
* @property name The endpoint name.
* When listing available contacts for a satellite, Ground Station searches for a dataflow endpoint whose name matches the value specified by the dataflow endpoint config of the selected mission profile. If no matching dataflow endpoints are found then Ground Station will not display any available contacts for the satellite.
*/
public data class DataflowEndpointGroupDataflowEndpointArgs(
public val address: Output? = null,
public val mtu: Output? = null,
public val name: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.groundstation.inputs.DataflowEndpointGroupDataflowEndpointArgs =
com.pulumi.awsnative.groundstation.inputs.DataflowEndpointGroupDataflowEndpointArgs.builder()
.address(address?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.mtu(mtu?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DataflowEndpointGroupDataflowEndpointArgs].
*/
@PulumiTagMarker
public class DataflowEndpointGroupDataflowEndpointArgsBuilder internal constructor() {
private var address: Output? = null
private var mtu: Output? = null
private var name: Output? = null
/**
* @param value The address and port of an endpoint.
*/
@JvmName("djksrmlpwgkdvrxi")
public suspend fun address(`value`: Output) {
this.address = value
}
/**
* @param value Maximum transmission unit (MTU) size in bytes of a dataflow endpoint. Valid values are between 1400 and 1500. A default value of 1500 is used if not set.
*/
@JvmName("nyucdfnwpaiwxbwp")
public suspend fun mtu(`value`: Output) {
this.mtu = value
}
/**
* @param value The endpoint name.
* When listing available contacts for a satellite, Ground Station searches for a dataflow endpoint whose name matches the value specified by the dataflow endpoint config of the selected mission profile. If no matching dataflow endpoints are found then Ground Station will not display any available contacts for the satellite.
*/
@JvmName("afvhxwpfvoxtnmnj")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The address and port of an endpoint.
*/
@JvmName("joxwlgsxusnekkkf")
public suspend fun address(`value`: DataflowEndpointGroupSocketAddressArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.address = mapped
}
/**
* @param argument The address and port of an endpoint.
*/
@JvmName("kawquuosvsjguser")
public suspend fun address(argument: suspend DataflowEndpointGroupSocketAddressArgsBuilder.() -> Unit) {
val toBeMapped = DataflowEndpointGroupSocketAddressArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.address = mapped
}
/**
* @param value Maximum transmission unit (MTU) size in bytes of a dataflow endpoint. Valid values are between 1400 and 1500. A default value of 1500 is used if not set.
*/
@JvmName("vrrfiqhqvkvtqmin")
public suspend fun mtu(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mtu = mapped
}
/**
* @param value The endpoint name.
* When listing available contacts for a satellite, Ground Station searches for a dataflow endpoint whose name matches the value specified by the dataflow endpoint config of the selected mission profile. If no matching dataflow endpoints are found then Ground Station will not display any available contacts for the satellite.
*/
@JvmName("otgjkewujabxehxf")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
internal fun build(): DataflowEndpointGroupDataflowEndpointArgs =
DataflowEndpointGroupDataflowEndpointArgs(
address = address,
mtu = mtu,
name = name,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy