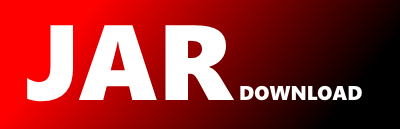
com.pulumi.awsnative.iot.kotlin.inputs.MitigationActionActionParamsArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.iot.kotlin.inputs
import com.pulumi.awsnative.iot.inputs.MitigationActionActionParamsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* The set of parameters for this mitigation action. You can specify only one type of parameter (in other words, you can apply only one action for each defined mitigation action).
* @property addThingsToThingGroupParams Specifies the group to which you want to add the devices.
* @property enableIoTLoggingParams Specifies the logging level and the role with permissions for logging. You cannot specify a logging level of `DISABLED` .
* @property publishFindingToSnsParams Specifies the topic to which the finding should be published.
* @property replaceDefaultPolicyVersionParams Replaces the policy version with a default or blank policy. You specify the template name. Only a value of `BLANK_POLICY` is currently supported.
* @property updateCaCertificateParams Specifies the new state for the CA certificate. Only a value of `DEACTIVATE` is currently supported.
* @property updateDeviceCertificateParams Specifies the new state for a device certificate. Only a value of `DEACTIVATE` is currently supported.
*/
public data class MitigationActionActionParamsArgs(
public val addThingsToThingGroupParams: Output? =
null,
public val enableIoTLoggingParams: Output? = null,
public val publishFindingToSnsParams: Output? =
null,
public val replaceDefaultPolicyVersionParams: Output? = null,
public val updateCaCertificateParams: Output? =
null,
public val updateDeviceCertificateParams: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.iot.inputs.MitigationActionActionParamsArgs =
com.pulumi.awsnative.iot.inputs.MitigationActionActionParamsArgs.builder()
.addThingsToThingGroupParams(
addThingsToThingGroupParams?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.enableIoTLoggingParams(
enableIoTLoggingParams?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.publishFindingToSnsParams(
publishFindingToSnsParams?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.replaceDefaultPolicyVersionParams(
replaceDefaultPolicyVersionParams?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.updateCaCertificateParams(
updateCaCertificateParams?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.updateDeviceCertificateParams(
updateDeviceCertificateParams?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
).build()
}
/**
* Builder for [MitigationActionActionParamsArgs].
*/
@PulumiTagMarker
public class MitigationActionActionParamsArgsBuilder internal constructor() {
private var addThingsToThingGroupParams: Output? =
null
private var enableIoTLoggingParams: Output? = null
private var publishFindingToSnsParams: Output? =
null
private var replaceDefaultPolicyVersionParams:
Output? = null
private var updateCaCertificateParams: Output? =
null
private var updateDeviceCertificateParams:
Output? = null
/**
* @param value Specifies the group to which you want to add the devices.
*/
@JvmName("njvnopepjhndndnu")
public suspend fun addThingsToThingGroupParams(`value`: Output) {
this.addThingsToThingGroupParams = value
}
/**
* @param value Specifies the logging level and the role with permissions for logging. You cannot specify a logging level of `DISABLED` .
*/
@JvmName("ijwlttviledxiwwj")
public suspend fun enableIoTLoggingParams(`value`: Output) {
this.enableIoTLoggingParams = value
}
/**
* @param value Specifies the topic to which the finding should be published.
*/
@JvmName("hsneffqsiofsapwc")
public suspend fun publishFindingToSnsParams(`value`: Output) {
this.publishFindingToSnsParams = value
}
/**
* @param value Replaces the policy version with a default or blank policy. You specify the template name. Only a value of `BLANK_POLICY` is currently supported.
*/
@JvmName("fpwwbiopuknxhcmn")
public suspend fun replaceDefaultPolicyVersionParams(`value`: Output) {
this.replaceDefaultPolicyVersionParams = value
}
/**
* @param value Specifies the new state for the CA certificate. Only a value of `DEACTIVATE` is currently supported.
*/
@JvmName("uygeedlvrdhgohgu")
public suspend fun updateCaCertificateParams(`value`: Output) {
this.updateCaCertificateParams = value
}
/**
* @param value Specifies the new state for a device certificate. Only a value of `DEACTIVATE` is currently supported.
*/
@JvmName("tibrmhtlfqpbxrku")
public suspend fun updateDeviceCertificateParams(`value`: Output) {
this.updateDeviceCertificateParams = value
}
/**
* @param value Specifies the group to which you want to add the devices.
*/
@JvmName("aarsxiwbsftomvlx")
public suspend fun addThingsToThingGroupParams(`value`: MitigationActionAddThingsToThingGroupParamsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.addThingsToThingGroupParams = mapped
}
/**
* @param argument Specifies the group to which you want to add the devices.
*/
@JvmName("dxtoshqhcudeoxfy")
public suspend fun addThingsToThingGroupParams(argument: suspend MitigationActionAddThingsToThingGroupParamsArgsBuilder.() -> Unit) {
val toBeMapped = MitigationActionAddThingsToThingGroupParamsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.addThingsToThingGroupParams = mapped
}
/**
* @param value Specifies the logging level and the role with permissions for logging. You cannot specify a logging level of `DISABLED` .
*/
@JvmName("mobfnirtdttlodvw")
public suspend fun enableIoTLoggingParams(`value`: MitigationActionEnableIoTLoggingParamsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableIoTLoggingParams = mapped
}
/**
* @param argument Specifies the logging level and the role with permissions for logging. You cannot specify a logging level of `DISABLED` .
*/
@JvmName("tkcaabolprdwqyxd")
public suspend fun enableIoTLoggingParams(argument: suspend MitigationActionEnableIoTLoggingParamsArgsBuilder.() -> Unit) {
val toBeMapped = MitigationActionEnableIoTLoggingParamsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.enableIoTLoggingParams = mapped
}
/**
* @param value Specifies the topic to which the finding should be published.
*/
@JvmName("kiudrtybirujogev")
public suspend fun publishFindingToSnsParams(`value`: MitigationActionPublishFindingToSnsParamsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.publishFindingToSnsParams = mapped
}
/**
* @param argument Specifies the topic to which the finding should be published.
*/
@JvmName("mxisjaeoyfeypbit")
public suspend fun publishFindingToSnsParams(argument: suspend MitigationActionPublishFindingToSnsParamsArgsBuilder.() -> Unit) {
val toBeMapped = MitigationActionPublishFindingToSnsParamsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.publishFindingToSnsParams = mapped
}
/**
* @param value Replaces the policy version with a default or blank policy. You specify the template name. Only a value of `BLANK_POLICY` is currently supported.
*/
@JvmName("iupbvejgflviwwef")
public suspend fun replaceDefaultPolicyVersionParams(`value`: MitigationActionReplaceDefaultPolicyVersionParamsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.replaceDefaultPolicyVersionParams = mapped
}
/**
* @param argument Replaces the policy version with a default or blank policy. You specify the template name. Only a value of `BLANK_POLICY` is currently supported.
*/
@JvmName("bauhrjoxavvpkrpc")
public suspend fun replaceDefaultPolicyVersionParams(argument: suspend MitigationActionReplaceDefaultPolicyVersionParamsArgsBuilder.() -> Unit) {
val toBeMapped = MitigationActionReplaceDefaultPolicyVersionParamsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.replaceDefaultPolicyVersionParams = mapped
}
/**
* @param value Specifies the new state for the CA certificate. Only a value of `DEACTIVATE` is currently supported.
*/
@JvmName("shhuiofuowdopoll")
public suspend fun updateCaCertificateParams(`value`: MitigationActionUpdateCaCertificateParamsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.updateCaCertificateParams = mapped
}
/**
* @param argument Specifies the new state for the CA certificate. Only a value of `DEACTIVATE` is currently supported.
*/
@JvmName("hggokqsntvuolfng")
public suspend fun updateCaCertificateParams(argument: suspend MitigationActionUpdateCaCertificateParamsArgsBuilder.() -> Unit) {
val toBeMapped = MitigationActionUpdateCaCertificateParamsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.updateCaCertificateParams = mapped
}
/**
* @param value Specifies the new state for a device certificate. Only a value of `DEACTIVATE` is currently supported.
*/
@JvmName("alkelyocjrmkfuee")
public suspend fun updateDeviceCertificateParams(`value`: MitigationActionUpdateDeviceCertificateParamsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.updateDeviceCertificateParams = mapped
}
/**
* @param argument Specifies the new state for a device certificate. Only a value of `DEACTIVATE` is currently supported.
*/
@JvmName("qeyeaaebwrfajhsl")
public suspend fun updateDeviceCertificateParams(argument: suspend MitigationActionUpdateDeviceCertificateParamsArgsBuilder.() -> Unit) {
val toBeMapped = MitigationActionUpdateDeviceCertificateParamsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.updateDeviceCertificateParams = mapped
}
internal fun build(): MitigationActionActionParamsArgs = MitigationActionActionParamsArgs(
addThingsToThingGroupParams = addThingsToThingGroupParams,
enableIoTLoggingParams = enableIoTLoggingParams,
publishFindingToSnsParams = publishFindingToSnsParams,
replaceDefaultPolicyVersionParams = replaceDefaultPolicyVersionParams,
updateCaCertificateParams = updateCaCertificateParams,
updateDeviceCertificateParams = updateDeviceCertificateParams,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy