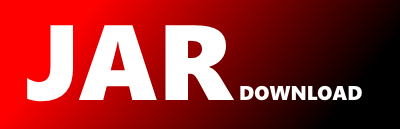
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleAction.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.iot.kotlin.outputs
import kotlin.Suppress
/**
*
* @property cloudwatchAlarm Change the state of a CloudWatch alarm.
* @property cloudwatchLogs Sends data to CloudWatch.
* @property cloudwatchMetric Capture a CloudWatch metric.
* @property dynamoDBv2 Write to a DynamoDB table. This is a new version of the DynamoDB action. It allows you to write each attribute in an MQTT message payload into a separate DynamoDB column.
* @property dynamoDb Write to a DynamoDB table.
* @property elasticsearch Write data to an Amazon OpenSearch Service domain.
* > The `Elasticsearch` action can only be used by existing rule actions. To create a new rule action or to update an existing rule action, use the `OpenSearch` rule action instead. For more information, see [OpenSearchAction](https://docs.aws.amazon.com//iot/latest/apireference/API_OpenSearchAction.html) .
* @property firehose Write to an Amazon Kinesis Firehose stream.
* @property http Send data to an HTTPS endpoint.
* @property iotAnalytics Sends message data to an AWS IoT Analytics channel.
* @property iotEvents Sends an input to an AWS IoT Events detector.
* @property iotSiteWise Sends data from the MQTT message that triggered the rule to AWS IoT SiteWise asset properties.
* @property kafka Send messages to an Amazon Managed Streaming for Apache Kafka (Amazon MSK) or self-managed Apache Kafka cluster.
* @property kinesis Write data to an Amazon Kinesis stream.
* @property lambda Invoke a Lambda function.
* @property location Sends device location data to [Amazon Location Service](https://docs.aws.amazon.com//location/latest/developerguide/welcome.html) .
* @property openSearch Write data to an Amazon OpenSearch Service domain.
* @property republish Publish to another MQTT topic.
* @property s3 Write to an Amazon S3 bucket.
* @property sns Publish to an Amazon SNS topic.
* @property sqs Publish to an Amazon SQS queue.
* @property stepFunctions Starts execution of a Step Functions state machine.
* @property timestream Writes attributes from an MQTT message.
*/
public data class TopicRuleAction(
public val cloudwatchAlarm: TopicRuleCloudwatchAlarmAction? = null,
public val cloudwatchLogs: TopicRuleCloudwatchLogsAction? = null,
public val cloudwatchMetric: TopicRuleCloudwatchMetricAction? = null,
public val dynamoDBv2: TopicRuleDynamoDBv2Action? = null,
public val dynamoDb: TopicRuleDynamoDbAction? = null,
public val elasticsearch: TopicRuleElasticsearchAction? = null,
public val firehose: TopicRuleFirehoseAction? = null,
public val http: TopicRuleHttpAction? = null,
public val iotAnalytics: TopicRuleIotAnalyticsAction? = null,
public val iotEvents: TopicRuleIotEventsAction? = null,
public val iotSiteWise: TopicRuleIotSiteWiseAction? = null,
public val kafka: TopicRuleKafkaAction? = null,
public val kinesis: TopicRuleKinesisAction? = null,
public val lambda: TopicRuleLambdaAction? = null,
public val location: TopicRuleLocationAction? = null,
public val openSearch: TopicRuleOpenSearchAction? = null,
public val republish: TopicRuleRepublishAction? = null,
public val s3: TopicRuleS3Action? = null,
public val sns: TopicRuleSnsAction? = null,
public val sqs: TopicRuleSqsAction? = null,
public val stepFunctions: TopicRuleStepFunctionsAction? = null,
public val timestream: TopicRuleTimestreamAction? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.awsnative.iot.outputs.TopicRuleAction): TopicRuleAction = TopicRuleAction(
cloudwatchAlarm = javaType.cloudwatchAlarm().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleCloudwatchAlarmAction.Companion.toKotlin(args0)
})
}).orElse(null),
cloudwatchLogs = javaType.cloudwatchLogs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleCloudwatchLogsAction.Companion.toKotlin(args0)
})
}).orElse(null),
cloudwatchMetric = javaType.cloudwatchMetric().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleCloudwatchMetricAction.Companion.toKotlin(args0)
})
}).orElse(null),
dynamoDBv2 = javaType.dynamoDBv2().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleDynamoDBv2Action.Companion.toKotlin(args0)
})
}).orElse(null),
dynamoDb = javaType.dynamoDb().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleDynamoDbAction.Companion.toKotlin(args0)
})
}).orElse(null),
elasticsearch = javaType.elasticsearch().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleElasticsearchAction.Companion.toKotlin(args0)
})
}).orElse(null),
firehose = javaType.firehose().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleFirehoseAction.Companion.toKotlin(args0)
})
}).orElse(null),
http = javaType.http().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleHttpAction.Companion.toKotlin(args0)
})
}).orElse(null),
iotAnalytics = javaType.iotAnalytics().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleIotAnalyticsAction.Companion.toKotlin(args0)
})
}).orElse(null),
iotEvents = javaType.iotEvents().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleIotEventsAction.Companion.toKotlin(args0)
})
}).orElse(null),
iotSiteWise = javaType.iotSiteWise().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleIotSiteWiseAction.Companion.toKotlin(args0)
})
}).orElse(null),
kafka = javaType.kafka().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleKafkaAction.Companion.toKotlin(args0)
})
}).orElse(null),
kinesis = javaType.kinesis().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleKinesisAction.Companion.toKotlin(args0)
})
}).orElse(null),
lambda = javaType.lambda().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleLambdaAction.Companion.toKotlin(args0)
})
}).orElse(null),
location = javaType.location().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleLocationAction.Companion.toKotlin(args0)
})
}).orElse(null),
openSearch = javaType.openSearch().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleOpenSearchAction.Companion.toKotlin(args0)
})
}).orElse(null),
republish = javaType.republish().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleRepublishAction.Companion.toKotlin(args0)
})
}).orElse(null),
s3 = javaType.s3().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleS3Action.Companion.toKotlin(args0)
})
}).orElse(null),
sns = javaType.sns().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleSnsAction.Companion.toKotlin(args0)
})
}).orElse(null),
sqs = javaType.sqs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleSqsAction.Companion.toKotlin(args0)
})
}).orElse(null),
stepFunctions = javaType.stepFunctions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleStepFunctionsAction.Companion.toKotlin(args0)
})
}).orElse(null),
timestream = javaType.timestream().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iot.kotlin.outputs.TopicRuleTimestreamAction.Companion.toKotlin(args0)
})
}).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy