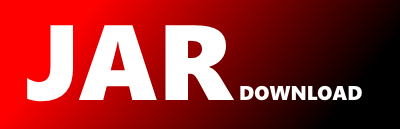
com.pulumi.awsnative.iotevents.kotlin.inputs.AlarmModelSimpleRuleArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.iotevents.kotlin.inputs
import com.pulumi.awsnative.iotevents.inputs.AlarmModelSimpleRuleArgs.builder
import com.pulumi.awsnative.iotevents.kotlin.enums.AlarmModelSimpleRuleComparisonOperator
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A rule that compares an input property value to a threshold value with a comparison operator.
* @property comparisonOperator The comparison operator.
* @property inputProperty The value on the left side of the comparison operator. You can specify an ITE input attribute as an input property.
* @property threshold The value on the right side of the comparison operator. You can enter a number or specify an ITE input attribute.
*/
public data class AlarmModelSimpleRuleArgs(
public val comparisonOperator: Output,
public val inputProperty: Output,
public val threshold: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.iotevents.inputs.AlarmModelSimpleRuleArgs =
com.pulumi.awsnative.iotevents.inputs.AlarmModelSimpleRuleArgs.builder()
.comparisonOperator(
comparisonOperator.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.inputProperty(inputProperty.applyValue({ args0 -> args0 }))
.threshold(threshold.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AlarmModelSimpleRuleArgs].
*/
@PulumiTagMarker
public class AlarmModelSimpleRuleArgsBuilder internal constructor() {
private var comparisonOperator: Output? = null
private var inputProperty: Output? = null
private var threshold: Output? = null
/**
* @param value The comparison operator.
*/
@JvmName("nixhfmfdpxrvmrqe")
public suspend fun comparisonOperator(`value`: Output) {
this.comparisonOperator = value
}
/**
* @param value The value on the left side of the comparison operator. You can specify an ITE input attribute as an input property.
*/
@JvmName("whbfdbfsdoinqnwk")
public suspend fun inputProperty(`value`: Output) {
this.inputProperty = value
}
/**
* @param value The value on the right side of the comparison operator. You can enter a number or specify an ITE input attribute.
*/
@JvmName("ybupxyjvjojpgmro")
public suspend fun threshold(`value`: Output) {
this.threshold = value
}
/**
* @param value The comparison operator.
*/
@JvmName("ymqmbxymamrcnkih")
public suspend fun comparisonOperator(`value`: AlarmModelSimpleRuleComparisonOperator) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.comparisonOperator = mapped
}
/**
* @param value The value on the left side of the comparison operator. You can specify an ITE input attribute as an input property.
*/
@JvmName("topwmeygeovqjwvr")
public suspend fun inputProperty(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.inputProperty = mapped
}
/**
* @param value The value on the right side of the comparison operator. You can enter a number or specify an ITE input attribute.
*/
@JvmName("rrjlsyvoypurqqcq")
public suspend fun threshold(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.threshold = mapped
}
internal fun build(): AlarmModelSimpleRuleArgs = AlarmModelSimpleRuleArgs(
comparisonOperator = comparisonOperator ?: throw PulumiNullFieldException("comparisonOperator"),
inputProperty = inputProperty ?: throw PulumiNullFieldException("inputProperty"),
threshold = threshold ?: throw PulumiNullFieldException("threshold"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy