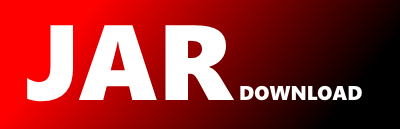
com.pulumi.awsnative.iotevents.kotlin.outputs.DetectorModelAction.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.iotevents.kotlin.outputs
import kotlin.Suppress
/**
* An action to be performed when the ``condition`` is TRUE.
* @property clearTimer Information needed to clear the timer.
* @property dynamoDBv2 Writes to the DynamoDB table that you created. The default action payload contains all attribute-value pairs that have the information about the detector model instance and the event that triggered the action. You can customize the [payload](https://docs.aws.amazon.com/iotevents/latest/apireference/API_Payload.html). A separate column of the DynamoDB table receives one attribute-value pair in the payload that you specify. For more information, see [Actions](https://docs.aws.amazon.com/iotevents/latest/developerguide/iotevents-event-actions.html) in *Developer Guide*.
* @property dynamoDb Writes to the DynamoDB table that you created. The default action payload contains all attribute-value pairs that have the information about the detector model instance and the event that triggered the action. You can customize the [payload](https://docs.aws.amazon.com/iotevents/latest/apireference/API_Payload.html). One column of the DynamoDB table receives all attribute-value pairs in the payload that you specify. For more information, see [Actions](https://docs.aws.amazon.com/iotevents/latest/developerguide/iotevents-event-actions.html) in *Developer Guide*.
* @property firehose Sends information about the detector model instance and the event that triggered the action to an Amazon Kinesis Data Firehose delivery stream.
* @property iotEvents Sends ITE input, which passes information about the detector model instance and the event that triggered the action.
* @property iotSiteWise Sends information about the detector model instance and the event that triggered the action to an asset property in ITSW .
* @property iotTopicPublish Publishes an MQTT message with the given topic to the IoT message broker.
* @property lambda Calls a Lambda function, passing in information about the detector model instance and the event that triggered the action.
* @property resetTimer Information needed to reset the timer.
* @property setTimer Information needed to set the timer.
* @property setVariable Sets a variable to a specified value.
* @property sns Sends an Amazon SNS message.
* @property sqs Sends an Amazon SNS message.
*/
public data class DetectorModelAction(
public val clearTimer: DetectorModelClearTimer? = null,
public val dynamoDBv2: DetectorModelDynamoDBv2? = null,
public val dynamoDb: DetectorModelDynamoDb? = null,
public val firehose: DetectorModelFirehose? = null,
public val iotEvents: DetectorModelIotEvents? = null,
public val iotSiteWise: DetectorModelIotSiteWise? = null,
public val iotTopicPublish: DetectorModelIotTopicPublish? = null,
public val lambda: DetectorModelLambda? = null,
public val resetTimer: DetectorModelResetTimer? = null,
public val setTimer: DetectorModelSetTimer? = null,
public val setVariable: DetectorModelSetVariable? = null,
public val sns: DetectorModelSns? = null,
public val sqs: DetectorModelSqs? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.awsnative.iotevents.outputs.DetectorModelAction): DetectorModelAction = DetectorModelAction(
clearTimer = javaType.clearTimer().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iotevents.kotlin.outputs.DetectorModelClearTimer.Companion.toKotlin(args0)
})
}).orElse(null),
dynamoDBv2 = javaType.dynamoDBv2().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iotevents.kotlin.outputs.DetectorModelDynamoDBv2.Companion.toKotlin(args0)
})
}).orElse(null),
dynamoDb = javaType.dynamoDb().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iotevents.kotlin.outputs.DetectorModelDynamoDb.Companion.toKotlin(args0)
})
}).orElse(null),
firehose = javaType.firehose().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iotevents.kotlin.outputs.DetectorModelFirehose.Companion.toKotlin(args0)
})
}).orElse(null),
iotEvents = javaType.iotEvents().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iotevents.kotlin.outputs.DetectorModelIotEvents.Companion.toKotlin(args0)
})
}).orElse(null),
iotSiteWise = javaType.iotSiteWise().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iotevents.kotlin.outputs.DetectorModelIotSiteWise.Companion.toKotlin(args0)
})
}).orElse(null),
iotTopicPublish = javaType.iotTopicPublish().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iotevents.kotlin.outputs.DetectorModelIotTopicPublish.Companion.toKotlin(args0)
})
}).orElse(null),
lambda = javaType.lambda().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iotevents.kotlin.outputs.DetectorModelLambda.Companion.toKotlin(args0)
})
}).orElse(null),
resetTimer = javaType.resetTimer().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iotevents.kotlin.outputs.DetectorModelResetTimer.Companion.toKotlin(args0)
})
}).orElse(null),
setTimer = javaType.setTimer().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iotevents.kotlin.outputs.DetectorModelSetTimer.Companion.toKotlin(args0)
})
}).orElse(null),
setVariable = javaType.setVariable().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iotevents.kotlin.outputs.DetectorModelSetVariable.Companion.toKotlin(args0)
})
}).orElse(null),
sns = javaType.sns().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iotevents.kotlin.outputs.DetectorModelSns.Companion.toKotlin(args0)
})
}).orElse(null),
sqs = javaType.sqs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.iotevents.kotlin.outputs.DetectorModelSqs.Companion.toKotlin(args0)
})
}).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy