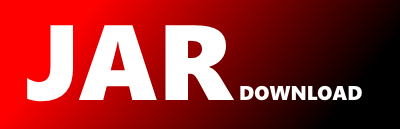
com.pulumi.awsnative.iotsitewise.kotlin.AssetModelArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.iotsitewise.kotlin
import com.pulumi.awsnative.iotsitewise.AssetModelArgs.builder
import com.pulumi.awsnative.iotsitewise.kotlin.inputs.AssetModelCompositeModelArgs
import com.pulumi.awsnative.iotsitewise.kotlin.inputs.AssetModelCompositeModelArgsBuilder
import com.pulumi.awsnative.iotsitewise.kotlin.inputs.AssetModelHierarchyArgs
import com.pulumi.awsnative.iotsitewise.kotlin.inputs.AssetModelHierarchyArgsBuilder
import com.pulumi.awsnative.iotsitewise.kotlin.inputs.AssetModelPropertyArgs
import com.pulumi.awsnative.iotsitewise.kotlin.inputs.AssetModelPropertyArgsBuilder
import com.pulumi.awsnative.kotlin.inputs.TagArgs
import com.pulumi.awsnative.kotlin.inputs.TagArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Resource schema for AWS::IoTSiteWise::AssetModel
* @property assetModelCompositeModels The composite asset models that are part of this asset model. Composite asset models are asset models that contain specific properties.
* @property assetModelDescription A description for the asset model.
* @property assetModelExternalId The external ID of the asset model.
* @property assetModelHierarchies The hierarchy definitions of the asset model. Each hierarchy specifies an asset model whose assets can be children of any other assets created from this asset model. You can specify up to 10 hierarchies per asset model.
* @property assetModelName A unique, friendly name for the asset model.
* @property assetModelProperties The property definitions of the asset model. You can specify up to 200 properties per asset model.
* @property assetModelType The type of the asset model (ASSET_MODEL OR COMPONENT_MODEL)
* @property tags A list of key-value pairs that contain metadata for the asset model.
*/
public data class AssetModelArgs(
public val assetModelCompositeModels: Output>? = null,
public val assetModelDescription: Output? = null,
public val assetModelExternalId: Output? = null,
public val assetModelHierarchies: Output>? = null,
public val assetModelName: Output? = null,
public val assetModelProperties: Output>? = null,
public val assetModelType: Output? = null,
public val tags: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.iotsitewise.AssetModelArgs =
com.pulumi.awsnative.iotsitewise.AssetModelArgs.builder()
.assetModelCompositeModels(
assetModelCompositeModels?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.assetModelDescription(assetModelDescription?.applyValue({ args0 -> args0 }))
.assetModelExternalId(assetModelExternalId?.applyValue({ args0 -> args0 }))
.assetModelHierarchies(
assetModelHierarchies?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.assetModelName(assetModelName?.applyValue({ args0 -> args0 }))
.assetModelProperties(
assetModelProperties?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.assetModelType(assetModelType?.applyValue({ args0 -> args0 }))
.tags(
tags?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [AssetModelArgs].
*/
@PulumiTagMarker
public class AssetModelArgsBuilder internal constructor() {
private var assetModelCompositeModels: Output>? = null
private var assetModelDescription: Output? = null
private var assetModelExternalId: Output? = null
private var assetModelHierarchies: Output>? = null
private var assetModelName: Output? = null
private var assetModelProperties: Output>? = null
private var assetModelType: Output? = null
private var tags: Output>? = null
/**
* @param value The composite asset models that are part of this asset model. Composite asset models are asset models that contain specific properties.
*/
@JvmName("ioubdhpldlvphurw")
public suspend fun assetModelCompositeModels(`value`: Output>) {
this.assetModelCompositeModels = value
}
@JvmName("htlubbsamwijvwff")
public suspend fun assetModelCompositeModels(vararg values: Output) {
this.assetModelCompositeModels = Output.all(values.asList())
}
/**
* @param values The composite asset models that are part of this asset model. Composite asset models are asset models that contain specific properties.
*/
@JvmName("rrbhtsyaqkjtkjlx")
public suspend fun assetModelCompositeModels(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy