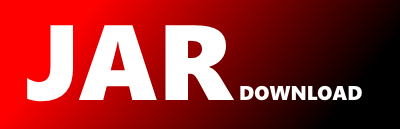
com.pulumi.awsnative.kendra.kotlin.inputs.DataSourceColumnConfigurationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.kendra.kotlin.inputs
import com.pulumi.awsnative.kendra.inputs.DataSourceColumnConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property changeDetectingColumns One to five columns that indicate when a document in the database has changed.
* @property documentDataColumnName The column that contains the contents of the document.
* @property documentIdColumnName The column that provides the document's identifier.
* @property documentTitleColumnName The column that contains the title of the document.
* @property fieldMappings An array of objects that map database column names to the corresponding fields in an index. You must first create the fields in the index using the [UpdateIndex](https://docs.aws.amazon.com/kendra/latest/dg/API_UpdateIndex.html) operation.
*/
public data class DataSourceColumnConfigurationArgs(
public val changeDetectingColumns: Output>,
public val documentDataColumnName: Output,
public val documentIdColumnName: Output,
public val documentTitleColumnName: Output? = null,
public val fieldMappings: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.kendra.inputs.DataSourceColumnConfigurationArgs =
com.pulumi.awsnative.kendra.inputs.DataSourceColumnConfigurationArgs.builder()
.changeDetectingColumns(changeDetectingColumns.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.documentDataColumnName(documentDataColumnName.applyValue({ args0 -> args0 }))
.documentIdColumnName(documentIdColumnName.applyValue({ args0 -> args0 }))
.documentTitleColumnName(documentTitleColumnName?.applyValue({ args0 -> args0 }))
.fieldMappings(
fieldMappings?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [DataSourceColumnConfigurationArgs].
*/
@PulumiTagMarker
public class DataSourceColumnConfigurationArgsBuilder internal constructor() {
private var changeDetectingColumns: Output>? = null
private var documentDataColumnName: Output? = null
private var documentIdColumnName: Output? = null
private var documentTitleColumnName: Output? = null
private var fieldMappings: Output>? = null
/**
* @param value One to five columns that indicate when a document in the database has changed.
*/
@JvmName("brhuibgrntosjyng")
public suspend fun changeDetectingColumns(`value`: Output>) {
this.changeDetectingColumns = value
}
@JvmName("pyqfdqqkfvnmvpjv")
public suspend fun changeDetectingColumns(vararg values: Output) {
this.changeDetectingColumns = Output.all(values.asList())
}
/**
* @param values One to five columns that indicate when a document in the database has changed.
*/
@JvmName("qglnuikxufnlslgh")
public suspend fun changeDetectingColumns(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy