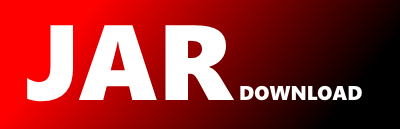
com.pulumi.awsnative.kinesisfirehose.kotlin.inputs.DeliveryStreamExtendedS3DestinationConfigurationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.kinesisfirehose.kotlin.inputs
import com.pulumi.awsnative.kinesisfirehose.inputs.DeliveryStreamExtendedS3DestinationConfigurationArgs.builder
import com.pulumi.awsnative.kinesisfirehose.kotlin.enums.DeliveryStreamExtendedS3DestinationConfigurationCompressionFormat
import com.pulumi.awsnative.kinesisfirehose.kotlin.enums.DeliveryStreamExtendedS3DestinationConfigurationS3BackupMode
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property bucketArn The Amazon Resource Name (ARN) of the Amazon S3 bucket. For constraints, see [ExtendedS3DestinationConfiguration](https://docs.aws.amazon.com/firehose/latest/APIReference/API_ExtendedS3DestinationConfiguration.html) in the *Amazon Kinesis Data Firehose API Reference* .
* @property bufferingHints The buffering option.
* @property cloudWatchLoggingOptions The Amazon CloudWatch logging options for your delivery stream.
* @property compressionFormat The compression format. If no value is specified, the default is `UNCOMPRESSED` .
* @property customTimeZone The time zone you prefer. UTC is the default.
* @property dataFormatConversionConfiguration The serializer, deserializer, and schema for converting data from the JSON format to the Parquet or ORC format before writing it to Amazon S3.
* @property dynamicPartitioningConfiguration The configuration of the dynamic partitioning mechanism that creates targeted data sets from the streaming data by partitioning it based on partition keys.
* @property encryptionConfiguration The encryption configuration for the Kinesis Data Firehose delivery stream. The default value is `NoEncryption` .
* @property errorOutputPrefix A prefix that Kinesis Data Firehose evaluates and adds to failed records before writing them to S3. This prefix appears immediately following the bucket name. For information about how to specify this prefix, see [Custom Prefixes for Amazon S3 Objects](https://docs.aws.amazon.com/firehose/latest/dev/s3-prefixes.html) .
* @property fileExtension Specify a file extension. It will override the default file extension
* @property prefix The `YYYY/MM/DD/HH` time format prefix is automatically used for delivered Amazon S3 files. For more information, see [ExtendedS3DestinationConfiguration](https://docs.aws.amazon.com/firehose/latest/APIReference/API_ExtendedS3DestinationConfiguration.html) in the *Amazon Kinesis Data Firehose API Reference* .
* @property processingConfiguration The data processing configuration for the Kinesis Data Firehose delivery stream.
* @property roleArn The Amazon Resource Name (ARN) of the AWS credentials. For constraints, see [ExtendedS3DestinationConfiguration](https://docs.aws.amazon.com/firehose/latest/APIReference/API_ExtendedS3DestinationConfiguration.html) in the *Amazon Kinesis Data Firehose API Reference* .
* @property s3BackupConfiguration The configuration for backup in Amazon S3.
* @property s3BackupMode The Amazon S3 backup mode. After you create a delivery stream, you can update it to enable Amazon S3 backup if it is disabled. If backup is enabled, you can't update the delivery stream to disable it.
*/
public data class DeliveryStreamExtendedS3DestinationConfigurationArgs(
public val bucketArn: Output,
public val bufferingHints: Output? = null,
public val cloudWatchLoggingOptions: Output? = null,
public val compressionFormat: Output? = null,
public val customTimeZone: Output? = null,
public val dataFormatConversionConfiguration: Output? = null,
public val dynamicPartitioningConfiguration: Output? = null,
public val encryptionConfiguration: Output? = null,
public val errorOutputPrefix: Output? = null,
public val fileExtension: Output? = null,
public val prefix: Output? = null,
public val processingConfiguration: Output? = null,
public val roleArn: Output,
public val s3BackupConfiguration: Output? = null,
public val s3BackupMode: Output? =
null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.kinesisfirehose.inputs.DeliveryStreamExtendedS3DestinationConfigurationArgs =
com.pulumi.awsnative.kinesisfirehose.inputs.DeliveryStreamExtendedS3DestinationConfigurationArgs.builder()
.bucketArn(bucketArn.applyValue({ args0 -> args0 }))
.bufferingHints(bufferingHints?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.cloudWatchLoggingOptions(
cloudWatchLoggingOptions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.compressionFormat(compressionFormat?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.customTimeZone(customTimeZone?.applyValue({ args0 -> args0 }))
.dataFormatConversionConfiguration(
dataFormatConversionConfiguration?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.dynamicPartitioningConfiguration(
dynamicPartitioningConfiguration?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.encryptionConfiguration(
encryptionConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.errorOutputPrefix(errorOutputPrefix?.applyValue({ args0 -> args0 }))
.fileExtension(fileExtension?.applyValue({ args0 -> args0 }))
.prefix(prefix?.applyValue({ args0 -> args0 }))
.processingConfiguration(
processingConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.roleArn(roleArn.applyValue({ args0 -> args0 }))
.s3BackupConfiguration(
s3BackupConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.s3BackupMode(s3BackupMode?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [DeliveryStreamExtendedS3DestinationConfigurationArgs].
*/
@PulumiTagMarker
public class DeliveryStreamExtendedS3DestinationConfigurationArgsBuilder internal constructor() {
private var bucketArn: Output? = null
private var bufferingHints: Output? = null
private var cloudWatchLoggingOptions: Output? = null
private var compressionFormat:
Output? = null
private var customTimeZone: Output? = null
private var dataFormatConversionConfiguration:
Output? = null
private var dynamicPartitioningConfiguration:
Output? = null
private var encryptionConfiguration: Output? = null
private var errorOutputPrefix: Output? = null
private var fileExtension: Output? = null
private var prefix: Output? = null
private var processingConfiguration: Output? = null
private var roleArn: Output? = null
private var s3BackupConfiguration: Output? = null
private var s3BackupMode: Output? =
null
/**
* @param value The Amazon Resource Name (ARN) of the Amazon S3 bucket. For constraints, see [ExtendedS3DestinationConfiguration](https://docs.aws.amazon.com/firehose/latest/APIReference/API_ExtendedS3DestinationConfiguration.html) in the *Amazon Kinesis Data Firehose API Reference* .
*/
@JvmName("jrpqwnjdcfdryuuk")
public suspend fun bucketArn(`value`: Output) {
this.bucketArn = value
}
/**
* @param value The buffering option.
*/
@JvmName("ynhviwrarpbkftyt")
public suspend fun bufferingHints(`value`: Output) {
this.bufferingHints = value
}
/**
* @param value The Amazon CloudWatch logging options for your delivery stream.
*/
@JvmName("qgaubfsngbwjterf")
public suspend fun cloudWatchLoggingOptions(`value`: Output) {
this.cloudWatchLoggingOptions = value
}
/**
* @param value The compression format. If no value is specified, the default is `UNCOMPRESSED` .
*/
@JvmName("srfildgsorwnskka")
public suspend fun compressionFormat(`value`: Output) {
this.compressionFormat = value
}
/**
* @param value The time zone you prefer. UTC is the default.
*/
@JvmName("dhumxddgqdlubctq")
public suspend fun customTimeZone(`value`: Output) {
this.customTimeZone = value
}
/**
* @param value The serializer, deserializer, and schema for converting data from the JSON format to the Parquet or ORC format before writing it to Amazon S3.
*/
@JvmName("sqcorfummstquybc")
public suspend fun dataFormatConversionConfiguration(`value`: Output) {
this.dataFormatConversionConfiguration = value
}
/**
* @param value The configuration of the dynamic partitioning mechanism that creates targeted data sets from the streaming data by partitioning it based on partition keys.
*/
@JvmName("tdmqpmxypuddhgmv")
public suspend fun dynamicPartitioningConfiguration(`value`: Output) {
this.dynamicPartitioningConfiguration = value
}
/**
* @param value The encryption configuration for the Kinesis Data Firehose delivery stream. The default value is `NoEncryption` .
*/
@JvmName("wekkolmyiunfhoko")
public suspend fun encryptionConfiguration(`value`: Output) {
this.encryptionConfiguration = value
}
/**
* @param value A prefix that Kinesis Data Firehose evaluates and adds to failed records before writing them to S3. This prefix appears immediately following the bucket name. For information about how to specify this prefix, see [Custom Prefixes for Amazon S3 Objects](https://docs.aws.amazon.com/firehose/latest/dev/s3-prefixes.html) .
*/
@JvmName("poluvkpobixeijbd")
public suspend fun errorOutputPrefix(`value`: Output) {
this.errorOutputPrefix = value
}
/**
* @param value Specify a file extension. It will override the default file extension
*/
@JvmName("yhcavmqmmyjfasof")
public suspend fun fileExtension(`value`: Output) {
this.fileExtension = value
}
/**
* @param value The `YYYY/MM/DD/HH` time format prefix is automatically used for delivered Amazon S3 files. For more information, see [ExtendedS3DestinationConfiguration](https://docs.aws.amazon.com/firehose/latest/APIReference/API_ExtendedS3DestinationConfiguration.html) in the *Amazon Kinesis Data Firehose API Reference* .
*/
@JvmName("gctawyieviyopcet")
public suspend fun prefix(`value`: Output) {
this.prefix = value
}
/**
* @param value The data processing configuration for the Kinesis Data Firehose delivery stream.
*/
@JvmName("xkepovryvjqfatlv")
public suspend fun processingConfiguration(`value`: Output) {
this.processingConfiguration = value
}
/**
* @param value The Amazon Resource Name (ARN) of the AWS credentials. For constraints, see [ExtendedS3DestinationConfiguration](https://docs.aws.amazon.com/firehose/latest/APIReference/API_ExtendedS3DestinationConfiguration.html) in the *Amazon Kinesis Data Firehose API Reference* .
*/
@JvmName("pahstxmfluryrgqi")
public suspend fun roleArn(`value`: Output) {
this.roleArn = value
}
/**
* @param value The configuration for backup in Amazon S3.
*/
@JvmName("lmbeibpsvcqhavxt")
public suspend fun s3BackupConfiguration(`value`: Output) {
this.s3BackupConfiguration = value
}
/**
* @param value The Amazon S3 backup mode. After you create a delivery stream, you can update it to enable Amazon S3 backup if it is disabled. If backup is enabled, you can't update the delivery stream to disable it.
*/
@JvmName("ognqhkxbsdniyavy")
public suspend fun s3BackupMode(`value`: Output) {
this.s3BackupMode = value
}
/**
* @param value The Amazon Resource Name (ARN) of the Amazon S3 bucket. For constraints, see [ExtendedS3DestinationConfiguration](https://docs.aws.amazon.com/firehose/latest/APIReference/API_ExtendedS3DestinationConfiguration.html) in the *Amazon Kinesis Data Firehose API Reference* .
*/
@JvmName("tgxhbtxmlikphhee")
public suspend fun bucketArn(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.bucketArn = mapped
}
/**
* @param value The buffering option.
*/
@JvmName("lvghcdauhwvndufh")
public suspend fun bufferingHints(`value`: DeliveryStreamBufferingHintsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bufferingHints = mapped
}
/**
* @param argument The buffering option.
*/
@JvmName("jnfsjtnuqcrfeicr")
public suspend fun bufferingHints(argument: suspend DeliveryStreamBufferingHintsArgsBuilder.() -> Unit) {
val toBeMapped = DeliveryStreamBufferingHintsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.bufferingHints = mapped
}
/**
* @param value The Amazon CloudWatch logging options for your delivery stream.
*/
@JvmName("bgjdukhhvgndkhky")
public suspend fun cloudWatchLoggingOptions(`value`: DeliveryStreamCloudWatchLoggingOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cloudWatchLoggingOptions = mapped
}
/**
* @param argument The Amazon CloudWatch logging options for your delivery stream.
*/
@JvmName("hwrjaacvlnmgavha")
public suspend fun cloudWatchLoggingOptions(argument: suspend DeliveryStreamCloudWatchLoggingOptionsArgsBuilder.() -> Unit) {
val toBeMapped = DeliveryStreamCloudWatchLoggingOptionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.cloudWatchLoggingOptions = mapped
}
/**
* @param value The compression format. If no value is specified, the default is `UNCOMPRESSED` .
*/
@JvmName("tfidcaaomyfkuack")
public suspend fun compressionFormat(`value`: DeliveryStreamExtendedS3DestinationConfigurationCompressionFormat?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.compressionFormat = mapped
}
/**
* @param value The time zone you prefer. UTC is the default.
*/
@JvmName("feptgjblwxfokwgd")
public suspend fun customTimeZone(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customTimeZone = mapped
}
/**
* @param value The serializer, deserializer, and schema for converting data from the JSON format to the Parquet or ORC format before writing it to Amazon S3.
*/
@JvmName("cdlbpxbclwrqokrf")
public suspend fun dataFormatConversionConfiguration(`value`: DeliveryStreamDataFormatConversionConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dataFormatConversionConfiguration = mapped
}
/**
* @param argument The serializer, deserializer, and schema for converting data from the JSON format to the Parquet or ORC format before writing it to Amazon S3.
*/
@JvmName("pdiaktbtrdyqkvxw")
public suspend fun dataFormatConversionConfiguration(argument: suspend DeliveryStreamDataFormatConversionConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DeliveryStreamDataFormatConversionConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.dataFormatConversionConfiguration = mapped
}
/**
* @param value The configuration of the dynamic partitioning mechanism that creates targeted data sets from the streaming data by partitioning it based on partition keys.
*/
@JvmName("ukgnrogjhpjrdhss")
public suspend fun dynamicPartitioningConfiguration(`value`: DeliveryStreamDynamicPartitioningConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dynamicPartitioningConfiguration = mapped
}
/**
* @param argument The configuration of the dynamic partitioning mechanism that creates targeted data sets from the streaming data by partitioning it based on partition keys.
*/
@JvmName("qcrnfrxdkxsnxuby")
public suspend fun dynamicPartitioningConfiguration(argument: suspend DeliveryStreamDynamicPartitioningConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DeliveryStreamDynamicPartitioningConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.dynamicPartitioningConfiguration = mapped
}
/**
* @param value The encryption configuration for the Kinesis Data Firehose delivery stream. The default value is `NoEncryption` .
*/
@JvmName("lapfsxfhyvvdjujo")
public suspend fun encryptionConfiguration(`value`: DeliveryStreamEncryptionConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encryptionConfiguration = mapped
}
/**
* @param argument The encryption configuration for the Kinesis Data Firehose delivery stream. The default value is `NoEncryption` .
*/
@JvmName("ypiepsxcmeijdeyn")
public suspend fun encryptionConfiguration(argument: suspend DeliveryStreamEncryptionConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DeliveryStreamEncryptionConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.encryptionConfiguration = mapped
}
/**
* @param value A prefix that Kinesis Data Firehose evaluates and adds to failed records before writing them to S3. This prefix appears immediately following the bucket name. For information about how to specify this prefix, see [Custom Prefixes for Amazon S3 Objects](https://docs.aws.amazon.com/firehose/latest/dev/s3-prefixes.html) .
*/
@JvmName("krhfettkacmqneom")
public suspend fun errorOutputPrefix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.errorOutputPrefix = mapped
}
/**
* @param value Specify a file extension. It will override the default file extension
*/
@JvmName("jkqxjnrsxtjhflyk")
public suspend fun fileExtension(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fileExtension = mapped
}
/**
* @param value The `YYYY/MM/DD/HH` time format prefix is automatically used for delivered Amazon S3 files. For more information, see [ExtendedS3DestinationConfiguration](https://docs.aws.amazon.com/firehose/latest/APIReference/API_ExtendedS3DestinationConfiguration.html) in the *Amazon Kinesis Data Firehose API Reference* .
*/
@JvmName("ntwaoxujptapqave")
public suspend fun prefix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.prefix = mapped
}
/**
* @param value The data processing configuration for the Kinesis Data Firehose delivery stream.
*/
@JvmName("caajoguaykuusapb")
public suspend fun processingConfiguration(`value`: DeliveryStreamProcessingConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.processingConfiguration = mapped
}
/**
* @param argument The data processing configuration for the Kinesis Data Firehose delivery stream.
*/
@JvmName("doduhouqngqnodqq")
public suspend fun processingConfiguration(argument: suspend DeliveryStreamProcessingConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DeliveryStreamProcessingConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.processingConfiguration = mapped
}
/**
* @param value The Amazon Resource Name (ARN) of the AWS credentials. For constraints, see [ExtendedS3DestinationConfiguration](https://docs.aws.amazon.com/firehose/latest/APIReference/API_ExtendedS3DestinationConfiguration.html) in the *Amazon Kinesis Data Firehose API Reference* .
*/
@JvmName("mnebfdwimmxjjgvd")
public suspend fun roleArn(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.roleArn = mapped
}
/**
* @param value The configuration for backup in Amazon S3.
*/
@JvmName("odmokaolpnwukpvr")
public suspend fun s3BackupConfiguration(`value`: DeliveryStreamS3DestinationConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.s3BackupConfiguration = mapped
}
/**
* @param argument The configuration for backup in Amazon S3.
*/
@JvmName("lxvvjoiomfkdhfof")
public suspend fun s3BackupConfiguration(argument: suspend DeliveryStreamS3DestinationConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DeliveryStreamS3DestinationConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.s3BackupConfiguration = mapped
}
/**
* @param value The Amazon S3 backup mode. After you create a delivery stream, you can update it to enable Amazon S3 backup if it is disabled. If backup is enabled, you can't update the delivery stream to disable it.
*/
@JvmName("jdcknaqkrapbwuqy")
public suspend fun s3BackupMode(`value`: DeliveryStreamExtendedS3DestinationConfigurationS3BackupMode?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.s3BackupMode = mapped
}
internal fun build(): DeliveryStreamExtendedS3DestinationConfigurationArgs =
DeliveryStreamExtendedS3DestinationConfigurationArgs(
bucketArn = bucketArn ?: throw PulumiNullFieldException("bucketArn"),
bufferingHints = bufferingHints,
cloudWatchLoggingOptions = cloudWatchLoggingOptions,
compressionFormat = compressionFormat,
customTimeZone = customTimeZone,
dataFormatConversionConfiguration = dataFormatConversionConfiguration,
dynamicPartitioningConfiguration = dynamicPartitioningConfiguration,
encryptionConfiguration = encryptionConfiguration,
errorOutputPrefix = errorOutputPrefix,
fileExtension = fileExtension,
prefix = prefix,
processingConfiguration = processingConfiguration,
roleArn = roleArn ?: throw PulumiNullFieldException("roleArn"),
s3BackupConfiguration = s3BackupConfiguration,
s3BackupMode = s3BackupMode,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy