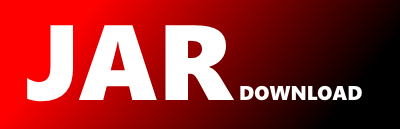
com.pulumi.awsnative.kinesisfirehose.kotlin.inputs.DeliveryStreamIcebergDestinationConfigurationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.kinesisfirehose.kotlin.inputs
import com.pulumi.awsnative.kinesisfirehose.inputs.DeliveryStreamIcebergDestinationConfigurationArgs.builder
import com.pulumi.awsnative.kinesisfirehose.kotlin.enums.DeliveryStreamIcebergDestinationConfigurations3BackupMode
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property bufferingHints
* @property catalogConfiguration Configuration describing where the destination Apache Iceberg Tables are persisted.
* Amazon Data Firehose is in preview release and is subject to change.
* @property cloudWatchLoggingOptions
* @property destinationTableConfigurationList Provides a list of `DestinationTableConfigurations` which Firehose uses to deliver data to Apache Iceberg Tables. Firehose will write data with insert if table specific configuration is not provided here.
* Amazon Data Firehose is in preview release and is subject to change.
* @property processingConfiguration
* @property retryOptions
* @property roleArn The Amazon Resource Name (ARN) of the the IAM role to be assumed by Firehose for calling Apache Iceberg Tables.
* Amazon Data Firehose is in preview release and is subject to change.
* @property s3BackupMode Describes how Firehose will backup records. Currently,S3 backup only supports `FailedDataOnly` for preview.
* Amazon Data Firehose is in preview release and is subject to change.
* @property s3Configuration
*/
public data class DeliveryStreamIcebergDestinationConfigurationArgs(
public val bufferingHints: Output? = null,
public val catalogConfiguration: Output,
public val cloudWatchLoggingOptions: Output? = null,
public val destinationTableConfigurationList: Output>? = null,
public val processingConfiguration: Output? = null,
public val retryOptions: Output? = null,
public val roleArn: Output,
public val s3BackupMode: Output? =
null,
public val s3Configuration: Output,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.kinesisfirehose.inputs.DeliveryStreamIcebergDestinationConfigurationArgs =
com.pulumi.awsnative.kinesisfirehose.inputs.DeliveryStreamIcebergDestinationConfigurationArgs.builder()
.bufferingHints(bufferingHints?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.catalogConfiguration(
catalogConfiguration.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.cloudWatchLoggingOptions(
cloudWatchLoggingOptions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.destinationTableConfigurationList(
destinationTableConfigurationList?.applyValue({ args0 ->
args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.processingConfiguration(
processingConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.retryOptions(retryOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.roleArn(roleArn.applyValue({ args0 -> args0 }))
.s3BackupMode(s3BackupMode?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.s3Configuration(
s3Configuration.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [DeliveryStreamIcebergDestinationConfigurationArgs].
*/
@PulumiTagMarker
public class DeliveryStreamIcebergDestinationConfigurationArgsBuilder internal constructor() {
private var bufferingHints: Output? = null
private var catalogConfiguration: Output? = null
private var cloudWatchLoggingOptions: Output? = null
private var destinationTableConfigurationList:
Output>? = null
private var processingConfiguration: Output? = null
private var retryOptions: Output? = null
private var roleArn: Output? = null
private var s3BackupMode: Output? =
null
private var s3Configuration: Output? = null
/**
* @param value
*/
@JvmName("jwlilujatxlrrhdv")
public suspend fun bufferingHints(`value`: Output) {
this.bufferingHints = value
}
/**
* @param value Configuration describing where the destination Apache Iceberg Tables are persisted.
* Amazon Data Firehose is in preview release and is subject to change.
*/
@JvmName("utkjilwsbntqtlax")
public suspend fun catalogConfiguration(`value`: Output) {
this.catalogConfiguration = value
}
/**
* @param value
*/
@JvmName("xsgldxgohdstnxtc")
public suspend fun cloudWatchLoggingOptions(`value`: Output) {
this.cloudWatchLoggingOptions = value
}
/**
* @param value Provides a list of `DestinationTableConfigurations` which Firehose uses to deliver data to Apache Iceberg Tables. Firehose will write data with insert if table specific configuration is not provided here.
* Amazon Data Firehose is in preview release and is subject to change.
*/
@JvmName("jabgvkgjnowcgmhl")
public suspend fun destinationTableConfigurationList(`value`: Output>) {
this.destinationTableConfigurationList = value
}
@JvmName("sahjwjnwbcmpjgmk")
public suspend fun destinationTableConfigurationList(vararg values: Output) {
this.destinationTableConfigurationList = Output.all(values.asList())
}
/**
* @param values Provides a list of `DestinationTableConfigurations` which Firehose uses to deliver data to Apache Iceberg Tables. Firehose will write data with insert if table specific configuration is not provided here.
* Amazon Data Firehose is in preview release and is subject to change.
*/
@JvmName("duqbooodtgwfbbnb")
public suspend fun destinationTableConfigurationList(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy