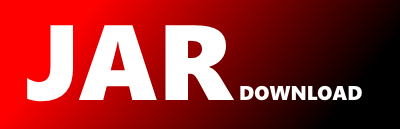
com.pulumi.awsnative.lambda.kotlin.EventInvokeConfigArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.lambda.kotlin
import com.pulumi.awsnative.lambda.EventInvokeConfigArgs.builder
import com.pulumi.awsnative.lambda.kotlin.inputs.EventInvokeConfigDestinationConfigArgs
import com.pulumi.awsnative.lambda.kotlin.inputs.EventInvokeConfigDestinationConfigArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* The AWS::Lambda::EventInvokeConfig resource configures options for asynchronous invocation on a version or an alias.
* @property destinationConfig A destination for events after they have been sent to a function for processing.
* **Destinations** - *Function* - The Amazon Resource Name (ARN) of a Lambda function.
* - *Queue* - The ARN of a standard SQS queue.
* - *Topic* - The ARN of a standard SNS topic.
* - *Event Bus* - The ARN of an Amazon EventBridge event bus.
* @property functionName The name of the Lambda function.
* @property maximumEventAgeInSeconds The maximum age of a request that Lambda sends to a function for processing.
* @property maximumRetryAttempts The maximum number of times to retry when the function returns an error.
* @property qualifier The identifier of a version or alias.
*/
public data class EventInvokeConfigArgs(
public val destinationConfig: Output? = null,
public val functionName: Output? = null,
public val maximumEventAgeInSeconds: Output? = null,
public val maximumRetryAttempts: Output? = null,
public val qualifier: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.lambda.EventInvokeConfigArgs =
com.pulumi.awsnative.lambda.EventInvokeConfigArgs.builder()
.destinationConfig(destinationConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.functionName(functionName?.applyValue({ args0 -> args0 }))
.maximumEventAgeInSeconds(maximumEventAgeInSeconds?.applyValue({ args0 -> args0 }))
.maximumRetryAttempts(maximumRetryAttempts?.applyValue({ args0 -> args0 }))
.qualifier(qualifier?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [EventInvokeConfigArgs].
*/
@PulumiTagMarker
public class EventInvokeConfigArgsBuilder internal constructor() {
private var destinationConfig: Output? = null
private var functionName: Output? = null
private var maximumEventAgeInSeconds: Output? = null
private var maximumRetryAttempts: Output? = null
private var qualifier: Output? = null
/**
* @param value A destination for events after they have been sent to a function for processing.
* **Destinations** - *Function* - The Amazon Resource Name (ARN) of a Lambda function.
* - *Queue* - The ARN of a standard SQS queue.
* - *Topic* - The ARN of a standard SNS topic.
* - *Event Bus* - The ARN of an Amazon EventBridge event bus.
*/
@JvmName("pconuykievlkorld")
public suspend fun destinationConfig(`value`: Output) {
this.destinationConfig = value
}
/**
* @param value The name of the Lambda function.
*/
@JvmName("fbdsbqxmsoeaqxqf")
public suspend fun functionName(`value`: Output) {
this.functionName = value
}
/**
* @param value The maximum age of a request that Lambda sends to a function for processing.
*/
@JvmName("mvpkgypbupxqubra")
public suspend fun maximumEventAgeInSeconds(`value`: Output) {
this.maximumEventAgeInSeconds = value
}
/**
* @param value The maximum number of times to retry when the function returns an error.
*/
@JvmName("aojuesvplylyytia")
public suspend fun maximumRetryAttempts(`value`: Output) {
this.maximumRetryAttempts = value
}
/**
* @param value The identifier of a version or alias.
*/
@JvmName("yhmbcrxcqyvjbksu")
public suspend fun qualifier(`value`: Output) {
this.qualifier = value
}
/**
* @param value A destination for events after they have been sent to a function for processing.
* **Destinations** - *Function* - The Amazon Resource Name (ARN) of a Lambda function.
* - *Queue* - The ARN of a standard SQS queue.
* - *Topic* - The ARN of a standard SNS topic.
* - *Event Bus* - The ARN of an Amazon EventBridge event bus.
*/
@JvmName("nddsifwxmucnpuuc")
public suspend fun destinationConfig(`value`: EventInvokeConfigDestinationConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.destinationConfig = mapped
}
/**
* @param argument A destination for events after they have been sent to a function for processing.
* **Destinations** - *Function* - The Amazon Resource Name (ARN) of a Lambda function.
* - *Queue* - The ARN of a standard SQS queue.
* - *Topic* - The ARN of a standard SNS topic.
* - *Event Bus* - The ARN of an Amazon EventBridge event bus.
*/
@JvmName("pbilsyefbusqqnim")
public suspend fun destinationConfig(argument: suspend EventInvokeConfigDestinationConfigArgsBuilder.() -> Unit) {
val toBeMapped = EventInvokeConfigDestinationConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.destinationConfig = mapped
}
/**
* @param value The name of the Lambda function.
*/
@JvmName("iadsvdjkruyircxu")
public suspend fun functionName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.functionName = mapped
}
/**
* @param value The maximum age of a request that Lambda sends to a function for processing.
*/
@JvmName("kqjcuibhjhvaudwx")
public suspend fun maximumEventAgeInSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maximumEventAgeInSeconds = mapped
}
/**
* @param value The maximum number of times to retry when the function returns an error.
*/
@JvmName("fdothbpagnapcsve")
public suspend fun maximumRetryAttempts(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maximumRetryAttempts = mapped
}
/**
* @param value The identifier of a version or alias.
*/
@JvmName("ifeetbvxylarpfol")
public suspend fun qualifier(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.qualifier = mapped
}
internal fun build(): EventInvokeConfigArgs = EventInvokeConfigArgs(
destinationConfig = destinationConfig,
functionName = functionName,
maximumEventAgeInSeconds = maximumEventAgeInSeconds,
maximumRetryAttempts = maximumRetryAttempts,
qualifier = qualifier,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy