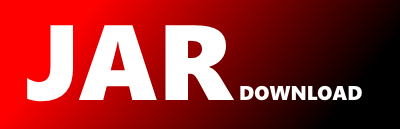
com.pulumi.awsnative.lambda.kotlin.LayerVersionArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.lambda.kotlin
import com.pulumi.awsnative.lambda.LayerVersionArgs.builder
import com.pulumi.awsnative.lambda.kotlin.inputs.LayerVersionContentArgs
import com.pulumi.awsnative.lambda.kotlin.inputs.LayerVersionContentArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Resource Type definition for AWS::Lambda::LayerVersion
* @property compatibleArchitectures A list of compatible instruction set architectures.
* @property compatibleRuntimes A list of compatible function runtimes. Used for filtering with ListLayers and ListLayerVersions.
* @property content The function layer archive.
* @property description The description of the version.
* @property layerName The name or Amazon Resource Name (ARN) of the layer.
* @property licenseInfo The layer's software license.
*/
public data class LayerVersionArgs(
public val compatibleArchitectures: Output>? = null,
public val compatibleRuntimes: Output>? = null,
public val content: Output? = null,
public val description: Output? = null,
public val layerName: Output? = null,
public val licenseInfo: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.lambda.LayerVersionArgs =
com.pulumi.awsnative.lambda.LayerVersionArgs.builder()
.compatibleArchitectures(
compatibleArchitectures?.applyValue({ args0 ->
args0.map({ args0 ->
args0
})
}),
)
.compatibleRuntimes(compatibleRuntimes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.content(content?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.description(description?.applyValue({ args0 -> args0 }))
.layerName(layerName?.applyValue({ args0 -> args0 }))
.licenseInfo(licenseInfo?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LayerVersionArgs].
*/
@PulumiTagMarker
public class LayerVersionArgsBuilder internal constructor() {
private var compatibleArchitectures: Output>? = null
private var compatibleRuntimes: Output>? = null
private var content: Output? = null
private var description: Output? = null
private var layerName: Output? = null
private var licenseInfo: Output? = null
/**
* @param value A list of compatible instruction set architectures.
*/
@JvmName("bxpsyaohdevbwacc")
public suspend fun compatibleArchitectures(`value`: Output>) {
this.compatibleArchitectures = value
}
@JvmName("xqrxjitufhcrajsh")
public suspend fun compatibleArchitectures(vararg values: Output) {
this.compatibleArchitectures = Output.all(values.asList())
}
/**
* @param values A list of compatible instruction set architectures.
*/
@JvmName("pbocdaixkfpgsmyu")
public suspend fun compatibleArchitectures(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy