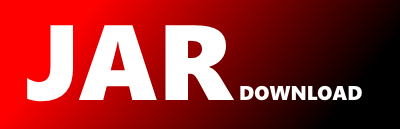
com.pulumi.awsnative.lambda.kotlin.UrlArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.lambda.kotlin
import com.pulumi.awsnative.lambda.UrlArgs.builder
import com.pulumi.awsnative.lambda.kotlin.enums.UrlAuthType
import com.pulumi.awsnative.lambda.kotlin.enums.UrlInvokeMode
import com.pulumi.awsnative.lambda.kotlin.inputs.UrlCorsArgs
import com.pulumi.awsnative.lambda.kotlin.inputs.UrlCorsArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Resource Type definition for AWS::Lambda::Url
* @property authType Can be either AWS_IAM if the requests are authorized via IAM, or NONE if no authorization is configured on the Function URL.
* @property cors The [Cross-Origin Resource Sharing (CORS)](https://docs.aws.amazon.com/https://developer.mozilla.org/en-US/docs/Web/HTTP/CORS) settings for your function URL.
* @property invokeMode The invocation mode for the function's URL. Set to BUFFERED if you want to buffer responses before returning them to the client. Set to RESPONSE_STREAM if you want to stream responses, allowing faster time to first byte and larger response payload sizes. If not set, defaults to BUFFERED.
* @property qualifier The alias qualifier for the target function. If TargetFunctionArn is unqualified then Qualifier must be passed.
* @property targetFunctionArn The Amazon Resource Name (ARN) of the function associated with the Function URL.
*/
public data class UrlArgs(
public val authType: Output? = null,
public val cors: Output? = null,
public val invokeMode: Output? = null,
public val qualifier: Output? = null,
public val targetFunctionArn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.lambda.UrlArgs =
com.pulumi.awsnative.lambda.UrlArgs.builder()
.authType(authType?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.cors(cors?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.invokeMode(invokeMode?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.qualifier(qualifier?.applyValue({ args0 -> args0 }))
.targetFunctionArn(targetFunctionArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [UrlArgs].
*/
@PulumiTagMarker
public class UrlArgsBuilder internal constructor() {
private var authType: Output? = null
private var cors: Output? = null
private var invokeMode: Output? = null
private var qualifier: Output? = null
private var targetFunctionArn: Output? = null
/**
* @param value Can be either AWS_IAM if the requests are authorized via IAM, or NONE if no authorization is configured on the Function URL.
*/
@JvmName("cnftmphycoasjpfv")
public suspend fun authType(`value`: Output) {
this.authType = value
}
/**
* @param value The [Cross-Origin Resource Sharing (CORS)](https://docs.aws.amazon.com/https://developer.mozilla.org/en-US/docs/Web/HTTP/CORS) settings for your function URL.
*/
@JvmName("pjymhgrmxdwmivkf")
public suspend fun cors(`value`: Output) {
this.cors = value
}
/**
* @param value The invocation mode for the function's URL. Set to BUFFERED if you want to buffer responses before returning them to the client. Set to RESPONSE_STREAM if you want to stream responses, allowing faster time to first byte and larger response payload sizes. If not set, defaults to BUFFERED.
*/
@JvmName("cgcqrlhtqgvkwpro")
public suspend fun invokeMode(`value`: Output) {
this.invokeMode = value
}
/**
* @param value The alias qualifier for the target function. If TargetFunctionArn is unqualified then Qualifier must be passed.
*/
@JvmName("niqjbxielkvhofhf")
public suspend fun qualifier(`value`: Output) {
this.qualifier = value
}
/**
* @param value The Amazon Resource Name (ARN) of the function associated with the Function URL.
*/
@JvmName("kathdhlctkqknngt")
public suspend fun targetFunctionArn(`value`: Output) {
this.targetFunctionArn = value
}
/**
* @param value Can be either AWS_IAM if the requests are authorized via IAM, or NONE if no authorization is configured on the Function URL.
*/
@JvmName("otvuubjiftqdlxyq")
public suspend fun authType(`value`: UrlAuthType?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.authType = mapped
}
/**
* @param value The [Cross-Origin Resource Sharing (CORS)](https://docs.aws.amazon.com/https://developer.mozilla.org/en-US/docs/Web/HTTP/CORS) settings for your function URL.
*/
@JvmName("dpqgddlybmwlhhxw")
public suspend fun cors(`value`: UrlCorsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cors = mapped
}
/**
* @param argument The [Cross-Origin Resource Sharing (CORS)](https://docs.aws.amazon.com/https://developer.mozilla.org/en-US/docs/Web/HTTP/CORS) settings for your function URL.
*/
@JvmName("trlihuwgnrsjxrji")
public suspend fun cors(argument: suspend UrlCorsArgsBuilder.() -> Unit) {
val toBeMapped = UrlCorsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.cors = mapped
}
/**
* @param value The invocation mode for the function's URL. Set to BUFFERED if you want to buffer responses before returning them to the client. Set to RESPONSE_STREAM if you want to stream responses, allowing faster time to first byte and larger response payload sizes. If not set, defaults to BUFFERED.
*/
@JvmName("prnggespcsuscaua")
public suspend fun invokeMode(`value`: UrlInvokeMode?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.invokeMode = mapped
}
/**
* @param value The alias qualifier for the target function. If TargetFunctionArn is unqualified then Qualifier must be passed.
*/
@JvmName("huocjjroetqdjkau")
public suspend fun qualifier(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.qualifier = mapped
}
/**
* @param value The Amazon Resource Name (ARN) of the function associated with the Function URL.
*/
@JvmName("gtjevqftqsrnmvgd")
public suspend fun targetFunctionArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetFunctionArn = mapped
}
internal fun build(): UrlArgs = UrlArgs(
authType = authType,
cors = cors,
invokeMode = invokeMode,
qualifier = qualifier,
targetFunctionArn = targetFunctionArn,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy