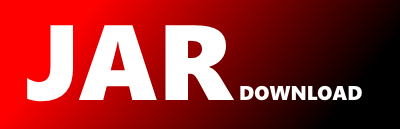
com.pulumi.awsnative.lex.kotlin.inputs.BotCustomVocabularyItemArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.lex.kotlin.inputs
import com.pulumi.awsnative.lex.inputs.BotCustomVocabularyItemArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A custom vocabulary item that contains the phrase to recognize and a weight to give the boost.
* @property displayAs Defines how you want your phrase to look in your transcription output.
* @property phrase Phrase that should be recognized.
* @property weight The degree to which the phrase recognition is boosted. The weight 0 means that no boosting will be applied and the entry will only be used for performing replacements using the displayAs field.
*/
public data class BotCustomVocabularyItemArgs(
public val displayAs: Output? = null,
public val phrase: Output,
public val weight: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.lex.inputs.BotCustomVocabularyItemArgs =
com.pulumi.awsnative.lex.inputs.BotCustomVocabularyItemArgs.builder()
.displayAs(displayAs?.applyValue({ args0 -> args0 }))
.phrase(phrase.applyValue({ args0 -> args0 }))
.weight(weight?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BotCustomVocabularyItemArgs].
*/
@PulumiTagMarker
public class BotCustomVocabularyItemArgsBuilder internal constructor() {
private var displayAs: Output? = null
private var phrase: Output? = null
private var weight: Output? = null
/**
* @param value Defines how you want your phrase to look in your transcription output.
*/
@JvmName("ljbobhrmgfseckgl")
public suspend fun displayAs(`value`: Output) {
this.displayAs = value
}
/**
* @param value Phrase that should be recognized.
*/
@JvmName("ytntubjyrpqsrgof")
public suspend fun phrase(`value`: Output) {
this.phrase = value
}
/**
* @param value The degree to which the phrase recognition is boosted. The weight 0 means that no boosting will be applied and the entry will only be used for performing replacements using the displayAs field.
*/
@JvmName("qwtqdfrfufciasbg")
public suspend fun weight(`value`: Output) {
this.weight = value
}
/**
* @param value Defines how you want your phrase to look in your transcription output.
*/
@JvmName("pyqirhkfdjtwscjs")
public suspend fun displayAs(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.displayAs = mapped
}
/**
* @param value Phrase that should be recognized.
*/
@JvmName("bmhdbqxjufhgvqks")
public suspend fun phrase(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.phrase = mapped
}
/**
* @param value The degree to which the phrase recognition is boosted. The weight 0 means that no boosting will be applied and the entry will only be used for performing replacements using the displayAs field.
*/
@JvmName("optyxnqayuefwhhl")
public suspend fun weight(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.weight = mapped
}
internal fun build(): BotCustomVocabularyItemArgs = BotCustomVocabularyItemArgs(
displayAs = displayAs,
phrase = phrase ?: throw PulumiNullFieldException("phrase"),
weight = weight,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy