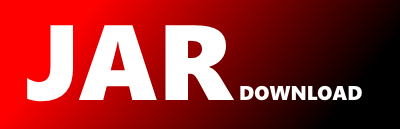
com.pulumi.awsnative.lex.kotlin.inputs.BotPostDialogCodeHookInvocationSpecificationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.lex.kotlin.inputs
import com.pulumi.awsnative.lex.inputs.BotPostDialogCodeHookInvocationSpecificationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Specifies next steps to run after the dialog code hook finishes.
* @property failureConditional A list of conditional branches to evaluate after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed.
* @property failureNextStep Specifies the next step the bot runs after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed.
* @property failureResponse Specifies a list of message groups that Amazon Lex uses to respond the user input.
* @property successConditional A list of conditional branches to evaluate after the dialog code hook finishes successfully.
* @property successNextStep Specifics the next step the bot runs after the dialog code hook finishes successfully.
* @property successResponse Specifies a list of message groups that Amazon Lex uses to respond the user input.
* @property timeoutConditional A list of conditional branches to evaluate if the code hook times out.
* @property timeoutNextStep Specifies the next step that the bot runs when the code hook times out.
* @property timeoutResponse Specifies a list of message groups that Amazon Lex uses to respond the user input.
*/
public data class BotPostDialogCodeHookInvocationSpecificationArgs(
public val failureConditional: Output? = null,
public val failureNextStep: Output? = null,
public val failureResponse: Output? = null,
public val successConditional: Output? = null,
public val successNextStep: Output? = null,
public val successResponse: Output? = null,
public val timeoutConditional: Output? = null,
public val timeoutNextStep: Output? = null,
public val timeoutResponse: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.lex.inputs.BotPostDialogCodeHookInvocationSpecificationArgs =
com.pulumi.awsnative.lex.inputs.BotPostDialogCodeHookInvocationSpecificationArgs.builder()
.failureConditional(
failureConditional?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.failureNextStep(failureNextStep?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.failureResponse(failureResponse?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.successConditional(
successConditional?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.successNextStep(successNextStep?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.successResponse(successResponse?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.timeoutConditional(
timeoutConditional?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.timeoutNextStep(timeoutNextStep?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.timeoutResponse(
timeoutResponse?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [BotPostDialogCodeHookInvocationSpecificationArgs].
*/
@PulumiTagMarker
public class BotPostDialogCodeHookInvocationSpecificationArgsBuilder internal constructor() {
private var failureConditional: Output? = null
private var failureNextStep: Output? = null
private var failureResponse: Output? = null
private var successConditional: Output? = null
private var successNextStep: Output? = null
private var successResponse: Output? = null
private var timeoutConditional: Output? = null
private var timeoutNextStep: Output? = null
private var timeoutResponse: Output? = null
/**
* @param value A list of conditional branches to evaluate after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed.
*/
@JvmName("kagjfnmjqfonxltj")
public suspend fun failureConditional(`value`: Output) {
this.failureConditional = value
}
/**
* @param value Specifies the next step the bot runs after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed.
*/
@JvmName("gwirthxoykielbyd")
public suspend fun failureNextStep(`value`: Output) {
this.failureNextStep = value
}
/**
* @param value Specifies a list of message groups that Amazon Lex uses to respond the user input.
*/
@JvmName("gduiwtnxepqnbiyh")
public suspend fun failureResponse(`value`: Output) {
this.failureResponse = value
}
/**
* @param value A list of conditional branches to evaluate after the dialog code hook finishes successfully.
*/
@JvmName("vacuqlrrkrwkhjlp")
public suspend fun successConditional(`value`: Output) {
this.successConditional = value
}
/**
* @param value Specifics the next step the bot runs after the dialog code hook finishes successfully.
*/
@JvmName("drtvmcwpsfbkotyk")
public suspend fun successNextStep(`value`: Output) {
this.successNextStep = value
}
/**
* @param value Specifies a list of message groups that Amazon Lex uses to respond the user input.
*/
@JvmName("terdyrkregwqlwif")
public suspend fun successResponse(`value`: Output) {
this.successResponse = value
}
/**
* @param value A list of conditional branches to evaluate if the code hook times out.
*/
@JvmName("tdhlyetwukifnmdu")
public suspend fun timeoutConditional(`value`: Output) {
this.timeoutConditional = value
}
/**
* @param value Specifies the next step that the bot runs when the code hook times out.
*/
@JvmName("plemmgeronwvswsg")
public suspend fun timeoutNextStep(`value`: Output) {
this.timeoutNextStep = value
}
/**
* @param value Specifies a list of message groups that Amazon Lex uses to respond the user input.
*/
@JvmName("dnmyrpwiuwbpxogl")
public suspend fun timeoutResponse(`value`: Output) {
this.timeoutResponse = value
}
/**
* @param value A list of conditional branches to evaluate after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed.
*/
@JvmName("btrpupprrfdlmrka")
public suspend fun failureConditional(`value`: BotConditionalSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.failureConditional = mapped
}
/**
* @param argument A list of conditional branches to evaluate after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed.
*/
@JvmName("oxtgoirbsirceliv")
public suspend fun failureConditional(argument: suspend BotConditionalSpecificationArgsBuilder.() -> Unit) {
val toBeMapped = BotConditionalSpecificationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.failureConditional = mapped
}
/**
* @param value Specifies the next step the bot runs after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed.
*/
@JvmName("lqxwokkolacoplel")
public suspend fun failureNextStep(`value`: BotDialogStateArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.failureNextStep = mapped
}
/**
* @param argument Specifies the next step the bot runs after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed.
*/
@JvmName("oswbphrkvyjkuyus")
public suspend fun failureNextStep(argument: suspend BotDialogStateArgsBuilder.() -> Unit) {
val toBeMapped = BotDialogStateArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.failureNextStep = mapped
}
/**
* @param value Specifies a list of message groups that Amazon Lex uses to respond the user input.
*/
@JvmName("bdokxskivojdbmms")
public suspend fun failureResponse(`value`: BotResponseSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.failureResponse = mapped
}
/**
* @param argument Specifies a list of message groups that Amazon Lex uses to respond the user input.
*/
@JvmName("rvbnockalbcvnjge")
public suspend fun failureResponse(argument: suspend BotResponseSpecificationArgsBuilder.() -> Unit) {
val toBeMapped = BotResponseSpecificationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.failureResponse = mapped
}
/**
* @param value A list of conditional branches to evaluate after the dialog code hook finishes successfully.
*/
@JvmName("wprbqvdyichosnhd")
public suspend fun successConditional(`value`: BotConditionalSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.successConditional = mapped
}
/**
* @param argument A list of conditional branches to evaluate after the dialog code hook finishes successfully.
*/
@JvmName("oevunoyueaibhpoe")
public suspend fun successConditional(argument: suspend BotConditionalSpecificationArgsBuilder.() -> Unit) {
val toBeMapped = BotConditionalSpecificationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.successConditional = mapped
}
/**
* @param value Specifics the next step the bot runs after the dialog code hook finishes successfully.
*/
@JvmName("xhxekwgptfftxebo")
public suspend fun successNextStep(`value`: BotDialogStateArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.successNextStep = mapped
}
/**
* @param argument Specifics the next step the bot runs after the dialog code hook finishes successfully.
*/
@JvmName("wudieylsxdqsmuvd")
public suspend fun successNextStep(argument: suspend BotDialogStateArgsBuilder.() -> Unit) {
val toBeMapped = BotDialogStateArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.successNextStep = mapped
}
/**
* @param value Specifies a list of message groups that Amazon Lex uses to respond the user input.
*/
@JvmName("evyfymlxvnkwubhc")
public suspend fun successResponse(`value`: BotResponseSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.successResponse = mapped
}
/**
* @param argument Specifies a list of message groups that Amazon Lex uses to respond the user input.
*/
@JvmName("xcsfrfciqyadftwg")
public suspend fun successResponse(argument: suspend BotResponseSpecificationArgsBuilder.() -> Unit) {
val toBeMapped = BotResponseSpecificationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.successResponse = mapped
}
/**
* @param value A list of conditional branches to evaluate if the code hook times out.
*/
@JvmName("nartsrjkrhkjsikw")
public suspend fun timeoutConditional(`value`: BotConditionalSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeoutConditional = mapped
}
/**
* @param argument A list of conditional branches to evaluate if the code hook times out.
*/
@JvmName("wyxbfuwebciydetr")
public suspend fun timeoutConditional(argument: suspend BotConditionalSpecificationArgsBuilder.() -> Unit) {
val toBeMapped = BotConditionalSpecificationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.timeoutConditional = mapped
}
/**
* @param value Specifies the next step that the bot runs when the code hook times out.
*/
@JvmName("yerpujfdsjhxnbdq")
public suspend fun timeoutNextStep(`value`: BotDialogStateArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeoutNextStep = mapped
}
/**
* @param argument Specifies the next step that the bot runs when the code hook times out.
*/
@JvmName("kykcclhehqfsrwon")
public suspend fun timeoutNextStep(argument: suspend BotDialogStateArgsBuilder.() -> Unit) {
val toBeMapped = BotDialogStateArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.timeoutNextStep = mapped
}
/**
* @param value Specifies a list of message groups that Amazon Lex uses to respond the user input.
*/
@JvmName("sqxexvegjsbbegtg")
public suspend fun timeoutResponse(`value`: BotResponseSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeoutResponse = mapped
}
/**
* @param argument Specifies a list of message groups that Amazon Lex uses to respond the user input.
*/
@JvmName("vhlbnyprrormyreo")
public suspend fun timeoutResponse(argument: suspend BotResponseSpecificationArgsBuilder.() -> Unit) {
val toBeMapped = BotResponseSpecificationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.timeoutResponse = mapped
}
internal fun build(): BotPostDialogCodeHookInvocationSpecificationArgs =
BotPostDialogCodeHookInvocationSpecificationArgs(
failureConditional = failureConditional,
failureNextStep = failureNextStep,
failureResponse = failureResponse,
successConditional = successConditional,
successNextStep = successNextStep,
successResponse = successResponse,
timeoutConditional = timeoutConditional,
timeoutNextStep = timeoutNextStep,
timeoutResponse = timeoutResponse,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy