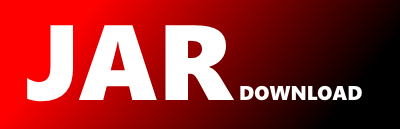
com.pulumi.awsnative.lex.kotlin.inputs.BotS3LocationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.lex.kotlin.inputs
import com.pulumi.awsnative.lex.inputs.BotS3LocationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* S3 location of bot definitions zip file, if it's not defined inline in CloudFormation.
* @property s3Bucket An Amazon S3 bucket in the same AWS Region as your function. The bucket can be in a different AWS account.
* @property s3ObjectKey The Amazon S3 key of the deployment package.
* @property s3ObjectVersion For versioned objects, the version of the deployment package object to use. If not specified, the current object version will be used.
*/
public data class BotS3LocationArgs(
public val s3Bucket: Output,
public val s3ObjectKey: Output,
public val s3ObjectVersion: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.lex.inputs.BotS3LocationArgs =
com.pulumi.awsnative.lex.inputs.BotS3LocationArgs.builder()
.s3Bucket(s3Bucket.applyValue({ args0 -> args0 }))
.s3ObjectKey(s3ObjectKey.applyValue({ args0 -> args0 }))
.s3ObjectVersion(s3ObjectVersion?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BotS3LocationArgs].
*/
@PulumiTagMarker
public class BotS3LocationArgsBuilder internal constructor() {
private var s3Bucket: Output? = null
private var s3ObjectKey: Output? = null
private var s3ObjectVersion: Output? = null
/**
* @param value An Amazon S3 bucket in the same AWS Region as your function. The bucket can be in a different AWS account.
*/
@JvmName("repqxlqyojdiqjwu")
public suspend fun s3Bucket(`value`: Output) {
this.s3Bucket = value
}
/**
* @param value The Amazon S3 key of the deployment package.
*/
@JvmName("qjvscwdergexmnft")
public suspend fun s3ObjectKey(`value`: Output) {
this.s3ObjectKey = value
}
/**
* @param value For versioned objects, the version of the deployment package object to use. If not specified, the current object version will be used.
*/
@JvmName("vlwguumpycfosdjj")
public suspend fun s3ObjectVersion(`value`: Output) {
this.s3ObjectVersion = value
}
/**
* @param value An Amazon S3 bucket in the same AWS Region as your function. The bucket can be in a different AWS account.
*/
@JvmName("yfpjsgesipxphyan")
public suspend fun s3Bucket(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.s3Bucket = mapped
}
/**
* @param value The Amazon S3 key of the deployment package.
*/
@JvmName("qyhjjqnjcvdflxcm")
public suspend fun s3ObjectKey(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.s3ObjectKey = mapped
}
/**
* @param value For versioned objects, the version of the deployment package object to use. If not specified, the current object version will be used.
*/
@JvmName("jrvgebdsysklmcfk")
public suspend fun s3ObjectVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.s3ObjectVersion = mapped
}
internal fun build(): BotS3LocationArgs = BotS3LocationArgs(
s3Bucket = s3Bucket ?: throw PulumiNullFieldException("s3Bucket"),
s3ObjectKey = s3ObjectKey ?: throw PulumiNullFieldException("s3ObjectKey"),
s3ObjectVersion = s3ObjectVersion,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy