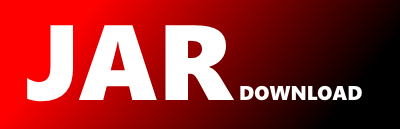
com.pulumi.awsnative.lex.kotlin.inputs.BotSlotCaptureSettingArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.lex.kotlin.inputs
import com.pulumi.awsnative.lex.inputs.BotSlotCaptureSettingArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Settings used when Amazon Lex successfully captures a slot value from a user.
* @property captureConditional A list of conditional branches to evaluate after the slot value is captured.
* @property captureNextStep Specifies the next step that the bot runs when the slot value is captured before the code hook times out.
* @property captureResponse Specifies a list of message groups that Amazon Lex uses to respond the user input.
* @property codeHook Code hook called after Amazon Lex successfully captures a slot value.
* @property elicitationCodeHook Code hook called when Amazon Lex doesn't capture a slot value.
* @property failureConditional A list of conditional branches to evaluate when the slot value isn't captured.
* @property failureNextStep Specifies the next step that the bot runs when the slot value code is not recognized.
* @property failureResponse Specifies a list of message groups that Amazon Lex uses to respond the user input.
*/
public data class BotSlotCaptureSettingArgs(
public val captureConditional: Output? = null,
public val captureNextStep: Output? = null,
public val captureResponse: Output? = null,
public val codeHook: Output? = null,
public val elicitationCodeHook: Output? = null,
public val failureConditional: Output? = null,
public val failureNextStep: Output? = null,
public val failureResponse: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.lex.inputs.BotSlotCaptureSettingArgs =
com.pulumi.awsnative.lex.inputs.BotSlotCaptureSettingArgs.builder()
.captureConditional(
captureConditional?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.captureNextStep(captureNextStep?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.captureResponse(captureResponse?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.codeHook(codeHook?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.elicitationCodeHook(
elicitationCodeHook?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.failureConditional(
failureConditional?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.failureNextStep(failureNextStep?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.failureResponse(
failureResponse?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [BotSlotCaptureSettingArgs].
*/
@PulumiTagMarker
public class BotSlotCaptureSettingArgsBuilder internal constructor() {
private var captureConditional: Output? = null
private var captureNextStep: Output? = null
private var captureResponse: Output? = null
private var codeHook: Output? = null
private var elicitationCodeHook: Output? = null
private var failureConditional: Output? = null
private var failureNextStep: Output? = null
private var failureResponse: Output? = null
/**
* @param value A list of conditional branches to evaluate after the slot value is captured.
*/
@JvmName("unpqnquvwscpcsyn")
public suspend fun captureConditional(`value`: Output) {
this.captureConditional = value
}
/**
* @param value Specifies the next step that the bot runs when the slot value is captured before the code hook times out.
*/
@JvmName("wqpcjwgyidwtthuu")
public suspend fun captureNextStep(`value`: Output) {
this.captureNextStep = value
}
/**
* @param value Specifies a list of message groups that Amazon Lex uses to respond the user input.
*/
@JvmName("qqkyywnvtnclsiqq")
public suspend fun captureResponse(`value`: Output) {
this.captureResponse = value
}
/**
* @param value Code hook called after Amazon Lex successfully captures a slot value.
*/
@JvmName("njnhkythcyhpfqum")
public suspend fun codeHook(`value`: Output) {
this.codeHook = value
}
/**
* @param value Code hook called when Amazon Lex doesn't capture a slot value.
*/
@JvmName("sywdhdxdxsockyai")
public suspend fun elicitationCodeHook(`value`: Output) {
this.elicitationCodeHook = value
}
/**
* @param value A list of conditional branches to evaluate when the slot value isn't captured.
*/
@JvmName("dxuoskltfwdfrtea")
public suspend fun failureConditional(`value`: Output) {
this.failureConditional = value
}
/**
* @param value Specifies the next step that the bot runs when the slot value code is not recognized.
*/
@JvmName("nhbwxiqvytwqtjaw")
public suspend fun failureNextStep(`value`: Output) {
this.failureNextStep = value
}
/**
* @param value Specifies a list of message groups that Amazon Lex uses to respond the user input.
*/
@JvmName("rnsveukhnvinqptb")
public suspend fun failureResponse(`value`: Output) {
this.failureResponse = value
}
/**
* @param value A list of conditional branches to evaluate after the slot value is captured.
*/
@JvmName("cmadckxlypthihxm")
public suspend fun captureConditional(`value`: BotConditionalSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.captureConditional = mapped
}
/**
* @param argument A list of conditional branches to evaluate after the slot value is captured.
*/
@JvmName("bdlrtlwybwxmyqed")
public suspend fun captureConditional(argument: suspend BotConditionalSpecificationArgsBuilder.() -> Unit) {
val toBeMapped = BotConditionalSpecificationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.captureConditional = mapped
}
/**
* @param value Specifies the next step that the bot runs when the slot value is captured before the code hook times out.
*/
@JvmName("hqrpdpfgmwbifmfi")
public suspend fun captureNextStep(`value`: BotDialogStateArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.captureNextStep = mapped
}
/**
* @param argument Specifies the next step that the bot runs when the slot value is captured before the code hook times out.
*/
@JvmName("tcstskccwsynvjhu")
public suspend fun captureNextStep(argument: suspend BotDialogStateArgsBuilder.() -> Unit) {
val toBeMapped = BotDialogStateArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.captureNextStep = mapped
}
/**
* @param value Specifies a list of message groups that Amazon Lex uses to respond the user input.
*/
@JvmName("iyhqugorihbqygff")
public suspend fun captureResponse(`value`: BotResponseSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.captureResponse = mapped
}
/**
* @param argument Specifies a list of message groups that Amazon Lex uses to respond the user input.
*/
@JvmName("vypfejqgyexuchjl")
public suspend fun captureResponse(argument: suspend BotResponseSpecificationArgsBuilder.() -> Unit) {
val toBeMapped = BotResponseSpecificationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.captureResponse = mapped
}
/**
* @param value Code hook called after Amazon Lex successfully captures a slot value.
*/
@JvmName("ttsvxvnlumcrbqph")
public suspend fun codeHook(`value`: BotDialogCodeHookInvocationSettingArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.codeHook = mapped
}
/**
* @param argument Code hook called after Amazon Lex successfully captures a slot value.
*/
@JvmName("xwpmqrehjmsajukw")
public suspend fun codeHook(argument: suspend BotDialogCodeHookInvocationSettingArgsBuilder.() -> Unit) {
val toBeMapped = BotDialogCodeHookInvocationSettingArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.codeHook = mapped
}
/**
* @param value Code hook called when Amazon Lex doesn't capture a slot value.
*/
@JvmName("ritoxltvrdmvafrj")
public suspend fun elicitationCodeHook(`value`: BotElicitationCodeHookInvocationSettingArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.elicitationCodeHook = mapped
}
/**
* @param argument Code hook called when Amazon Lex doesn't capture a slot value.
*/
@JvmName("xckyjxjfxdlqtfhh")
public suspend fun elicitationCodeHook(argument: suspend BotElicitationCodeHookInvocationSettingArgsBuilder.() -> Unit) {
val toBeMapped = BotElicitationCodeHookInvocationSettingArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.elicitationCodeHook = mapped
}
/**
* @param value A list of conditional branches to evaluate when the slot value isn't captured.
*/
@JvmName("webemumyitjndmnr")
public suspend fun failureConditional(`value`: BotConditionalSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.failureConditional = mapped
}
/**
* @param argument A list of conditional branches to evaluate when the slot value isn't captured.
*/
@JvmName("huvbdmbuptpsmfmh")
public suspend fun failureConditional(argument: suspend BotConditionalSpecificationArgsBuilder.() -> Unit) {
val toBeMapped = BotConditionalSpecificationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.failureConditional = mapped
}
/**
* @param value Specifies the next step that the bot runs when the slot value code is not recognized.
*/
@JvmName("uedlexrkffkcvsuo")
public suspend fun failureNextStep(`value`: BotDialogStateArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.failureNextStep = mapped
}
/**
* @param argument Specifies the next step that the bot runs when the slot value code is not recognized.
*/
@JvmName("pjhpvcphwafbpteu")
public suspend fun failureNextStep(argument: suspend BotDialogStateArgsBuilder.() -> Unit) {
val toBeMapped = BotDialogStateArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.failureNextStep = mapped
}
/**
* @param value Specifies a list of message groups that Amazon Lex uses to respond the user input.
*/
@JvmName("eoaxqvpecnmndlpa")
public suspend fun failureResponse(`value`: BotResponseSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.failureResponse = mapped
}
/**
* @param argument Specifies a list of message groups that Amazon Lex uses to respond the user input.
*/
@JvmName("unafceailndjoyio")
public suspend fun failureResponse(argument: suspend BotResponseSpecificationArgsBuilder.() -> Unit) {
val toBeMapped = BotResponseSpecificationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.failureResponse = mapped
}
internal fun build(): BotSlotCaptureSettingArgs = BotSlotCaptureSettingArgs(
captureConditional = captureConditional,
captureNextStep = captureNextStep,
captureResponse = captureResponse,
codeHook = codeHook,
elicitationCodeHook = elicitationCodeHook,
failureConditional = failureConditional,
failureNextStep = failureNextStep,
failureResponse = failureResponse,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy