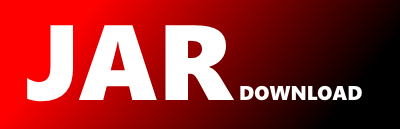
com.pulumi.awsnative.lex.kotlin.inputs.BotSlotValueSelectionSettingArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.lex.kotlin.inputs
import com.pulumi.awsnative.lex.inputs.BotSlotValueSelectionSettingArgs.builder
import com.pulumi.awsnative.lex.kotlin.enums.BotSlotValueResolutionStrategy
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Contains settings used by Amazon Lex to select a slot value.
* @property advancedRecognitionSetting Provides settings that enable advanced recognition settings for slot values. You can use this to enable using slot values as a custom vocabulary for recognizing user utterances.
* @property regexFilter A regular expression used to validate the value of a slot.
* @property resolutionStrategy Determines the slot resolution strategy that Amazon Lex uses to return slot type values. The field can be set to one of the following values:
* - `ORIGINAL_VALUE` - Returns the value entered by the user, if the user value is similar to the slot value.
* - `TOP_RESOLUTION` - If there is a resolution list for the slot, return the first value in the resolution list as the slot type value. If there is no resolution list, null is returned.
* If you don't specify the `valueSelectionStrategy` , the default is `ORIGINAL_VALUE` .
*/
public data class BotSlotValueSelectionSettingArgs(
public val advancedRecognitionSetting: Output? = null,
public val regexFilter: Output? = null,
public val resolutionStrategy: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.lex.inputs.BotSlotValueSelectionSettingArgs =
com.pulumi.awsnative.lex.inputs.BotSlotValueSelectionSettingArgs.builder()
.advancedRecognitionSetting(
advancedRecognitionSetting?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.regexFilter(regexFilter?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.resolutionStrategy(
resolutionStrategy.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [BotSlotValueSelectionSettingArgs].
*/
@PulumiTagMarker
public class BotSlotValueSelectionSettingArgsBuilder internal constructor() {
private var advancedRecognitionSetting: Output? = null
private var regexFilter: Output? = null
private var resolutionStrategy: Output? = null
/**
* @param value Provides settings that enable advanced recognition settings for slot values. You can use this to enable using slot values as a custom vocabulary for recognizing user utterances.
*/
@JvmName("gxuvjqkuhmpoojii")
public suspend fun advancedRecognitionSetting(`value`: Output) {
this.advancedRecognitionSetting = value
}
/**
* @param value A regular expression used to validate the value of a slot.
*/
@JvmName("gggrldkldfxqgmhs")
public suspend fun regexFilter(`value`: Output) {
this.regexFilter = value
}
/**
* @param value Determines the slot resolution strategy that Amazon Lex uses to return slot type values. The field can be set to one of the following values:
* - `ORIGINAL_VALUE` - Returns the value entered by the user, if the user value is similar to the slot value.
* - `TOP_RESOLUTION` - If there is a resolution list for the slot, return the first value in the resolution list as the slot type value. If there is no resolution list, null is returned.
* If you don't specify the `valueSelectionStrategy` , the default is `ORIGINAL_VALUE` .
*/
@JvmName("ouumofpmcjicxtof")
public suspend fun resolutionStrategy(`value`: Output) {
this.resolutionStrategy = value
}
/**
* @param value Provides settings that enable advanced recognition settings for slot values. You can use this to enable using slot values as a custom vocabulary for recognizing user utterances.
*/
@JvmName("itvskmvtvlbatomd")
public suspend fun advancedRecognitionSetting(`value`: BotAdvancedRecognitionSettingArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.advancedRecognitionSetting = mapped
}
/**
* @param argument Provides settings that enable advanced recognition settings for slot values. You can use this to enable using slot values as a custom vocabulary for recognizing user utterances.
*/
@JvmName("iamrrofrlmqfyngl")
public suspend fun advancedRecognitionSetting(argument: suspend BotAdvancedRecognitionSettingArgsBuilder.() -> Unit) {
val toBeMapped = BotAdvancedRecognitionSettingArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.advancedRecognitionSetting = mapped
}
/**
* @param value A regular expression used to validate the value of a slot.
*/
@JvmName("jfxubtdgxbqorrny")
public suspend fun regexFilter(`value`: BotSlotValueRegexFilterArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.regexFilter = mapped
}
/**
* @param argument A regular expression used to validate the value of a slot.
*/
@JvmName("kgxahvvxclckwuux")
public suspend fun regexFilter(argument: suspend BotSlotValueRegexFilterArgsBuilder.() -> Unit) {
val toBeMapped = BotSlotValueRegexFilterArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.regexFilter = mapped
}
/**
* @param value Determines the slot resolution strategy that Amazon Lex uses to return slot type values. The field can be set to one of the following values:
* - `ORIGINAL_VALUE` - Returns the value entered by the user, if the user value is similar to the slot value.
* - `TOP_RESOLUTION` - If there is a resolution list for the slot, return the first value in the resolution list as the slot type value. If there is no resolution list, null is returned.
* If you don't specify the `valueSelectionStrategy` , the default is `ORIGINAL_VALUE` .
*/
@JvmName("ukofobgihanxabio")
public suspend fun resolutionStrategy(`value`: BotSlotValueResolutionStrategy) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.resolutionStrategy = mapped
}
internal fun build(): BotSlotValueSelectionSettingArgs = BotSlotValueSelectionSettingArgs(
advancedRecognitionSetting = advancedRecognitionSetting,
regexFilter = regexFilter,
resolutionStrategy = resolutionStrategy ?: throw PulumiNullFieldException("resolutionStrategy"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy