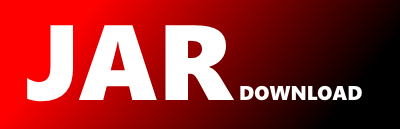
com.pulumi.awsnative.lex.kotlin.outputs.BotIntent.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.lex.kotlin.outputs
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
* Represents an action that the user wants to perform.
* @property description Description of thr intent.
* @property dialogCodeHook Specifies that Amazon Lex invokes the alias Lambda function for each user input. You can invoke this Lambda function to personalize user interaction.
* @property fulfillmentCodeHook Specifies that Amazon Lex invokes the alias Lambda function when the intent is ready for fulfillment. You can invoke this function to complete the bot's transaction with the user.
* @property initialResponseSetting Configuration setting for a response sent to the user before Amazon Lex starts eliciting slots.
* @property inputContexts A list of contexts that must be active for this intent to be considered by Amazon Lex .
* @property intentClosingSetting Sets the response that Amazon Lex sends to the user when the intent is closed.
* @property intentConfirmationSetting Provides prompts that Amazon Lex sends to the user to confirm the completion of an intent. If the user answers "no," the settings contain a statement that is sent to the user to end the intent.
* @property kendraConfiguration Provides configuration information for the `AMAZON.KendraSearchIntent` intent. When you use this intent, Amazon Lex searches the specified Amazon Kendra index and returns documents from the index that match the user's utterance.
* @property name The name of the intent.
* @property outputContexts A list of contexts that the intent activates when it is fulfilled.
* @property parentIntentSignature A unique identifier for the built-in intent to base this intent on.
* @property sampleUtterances A sample utterance that invokes an intent or respond to a slot elicitation prompt.
* @property slotPriorities Indicates the priority for slots. Amazon Lex prompts the user for slot values in priority order.
* @property slots List of slots
*/
public data class BotIntent(
public val description: String? = null,
public val dialogCodeHook: BotDialogCodeHookSetting? = null,
public val fulfillmentCodeHook: BotFulfillmentCodeHookSetting? = null,
public val initialResponseSetting: BotInitialResponseSetting? = null,
public val inputContexts: List? = null,
public val intentClosingSetting: BotIntentClosingSetting? = null,
public val intentConfirmationSetting: BotIntentConfirmationSetting? = null,
public val kendraConfiguration: BotKendraConfiguration? = null,
public val name: String,
public val outputContexts: List? = null,
public val parentIntentSignature: String? = null,
public val sampleUtterances: List? = null,
public val slotPriorities: List? = null,
public val slots: List? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.awsnative.lex.outputs.BotIntent): BotIntent =
BotIntent(
description = javaType.description().map({ args0 -> args0 }).orElse(null),
dialogCodeHook = javaType.dialogCodeHook().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.lex.kotlin.outputs.BotDialogCodeHookSetting.Companion.toKotlin(args0)
})
}).orElse(null),
fulfillmentCodeHook = javaType.fulfillmentCodeHook().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.lex.kotlin.outputs.BotFulfillmentCodeHookSetting.Companion.toKotlin(args0)
})
}).orElse(null),
initialResponseSetting = javaType.initialResponseSetting().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.lex.kotlin.outputs.BotInitialResponseSetting.Companion.toKotlin(args0)
})
}).orElse(null),
inputContexts = javaType.inputContexts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.lex.kotlin.outputs.BotInputContext.Companion.toKotlin(args0)
})
}),
intentClosingSetting = javaType.intentClosingSetting().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.lex.kotlin.outputs.BotIntentClosingSetting.Companion.toKotlin(args0)
})
}).orElse(null),
intentConfirmationSetting = javaType.intentConfirmationSetting().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.lex.kotlin.outputs.BotIntentConfirmationSetting.Companion.toKotlin(args0)
})
}).orElse(null),
kendraConfiguration = javaType.kendraConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.lex.kotlin.outputs.BotKendraConfiguration.Companion.toKotlin(args0)
})
}).orElse(null),
name = javaType.name(),
outputContexts = javaType.outputContexts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.lex.kotlin.outputs.BotOutputContext.Companion.toKotlin(args0)
})
}),
parentIntentSignature = javaType.parentIntentSignature().map({ args0 -> args0 }).orElse(null),
sampleUtterances = javaType.sampleUtterances().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.lex.kotlin.outputs.BotSampleUtterance.Companion.toKotlin(args0)
})
}),
slotPriorities = javaType.slotPriorities().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.lex.kotlin.outputs.BotSlotPriority.Companion.toKotlin(args0)
})
}),
slots = javaType.slots().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.lex.kotlin.outputs.BotSlot.Companion.toKotlin(args0)
})
}),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy