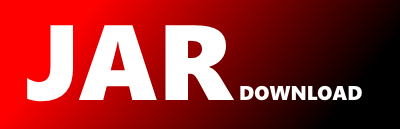
com.pulumi.awsnative.lightsail.kotlin.ContainerArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.lightsail.kotlin
import com.pulumi.awsnative.kotlin.inputs.TagArgs
import com.pulumi.awsnative.kotlin.inputs.TagArgsBuilder
import com.pulumi.awsnative.lightsail.ContainerArgs.builder
import com.pulumi.awsnative.lightsail.kotlin.inputs.ContainerPrivateRegistryAccessArgs
import com.pulumi.awsnative.lightsail.kotlin.inputs.ContainerPrivateRegistryAccessArgsBuilder
import com.pulumi.awsnative.lightsail.kotlin.inputs.ContainerPublicDomainNameArgs
import com.pulumi.awsnative.lightsail.kotlin.inputs.ContainerPublicDomainNameArgsBuilder
import com.pulumi.awsnative.lightsail.kotlin.inputs.ContainerServiceDeploymentArgs
import com.pulumi.awsnative.lightsail.kotlin.inputs.ContainerServiceDeploymentArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Resource Type definition for AWS::Lightsail::Container
* @property containerServiceDeployment Describes a container deployment configuration of an Amazon Lightsail container service.
* @property isDisabled A Boolean value to indicate whether the container service is disabled.
* @property power The power specification for the container service.
* @property privateRegistryAccess A Boolean value to indicate whether the container service has access to private container image repositories, such as Amazon Elastic Container Registry (Amazon ECR) private repositories.
* @property publicDomainNames The public domain names to use with the container service, such as example.com and www.example.com.
* @property scale The scale specification for the container service.
* @property serviceName The name for the container service.
* @property tags An array of key-value pairs to apply to this resource.
*/
public data class ContainerArgs(
public val containerServiceDeployment: Output? = null,
public val isDisabled: Output? = null,
public val power: Output? = null,
public val privateRegistryAccess: Output? = null,
public val publicDomainNames: Output>? = null,
public val scale: Output? = null,
public val serviceName: Output? = null,
public val tags: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.lightsail.ContainerArgs =
com.pulumi.awsnative.lightsail.ContainerArgs.builder()
.containerServiceDeployment(
containerServiceDeployment?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.isDisabled(isDisabled?.applyValue({ args0 -> args0 }))
.power(power?.applyValue({ args0 -> args0 }))
.privateRegistryAccess(
privateRegistryAccess?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.publicDomainNames(
publicDomainNames?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.scale(scale?.applyValue({ args0 -> args0 }))
.serviceName(serviceName?.applyValue({ args0 -> args0 }))
.tags(
tags?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [ContainerArgs].
*/
@PulumiTagMarker
public class ContainerArgsBuilder internal constructor() {
private var containerServiceDeployment: Output? = null
private var isDisabled: Output? = null
private var power: Output? = null
private var privateRegistryAccess: Output? = null
private var publicDomainNames: Output>? = null
private var scale: Output? = null
private var serviceName: Output? = null
private var tags: Output>? = null
/**
* @param value Describes a container deployment configuration of an Amazon Lightsail container service.
*/
@JvmName("cmlbqiirmmhkrmip")
public suspend fun containerServiceDeployment(`value`: Output) {
this.containerServiceDeployment = value
}
/**
* @param value A Boolean value to indicate whether the container service is disabled.
*/
@JvmName("ofrdqaxqssdmapyw")
public suspend fun isDisabled(`value`: Output) {
this.isDisabled = value
}
/**
* @param value The power specification for the container service.
*/
@JvmName("jgqyrcrmtdruidxd")
public suspend fun power(`value`: Output) {
this.power = value
}
/**
* @param value A Boolean value to indicate whether the container service has access to private container image repositories, such as Amazon Elastic Container Registry (Amazon ECR) private repositories.
*/
@JvmName("mxkgkasjbpwvcbiu")
public suspend fun privateRegistryAccess(`value`: Output) {
this.privateRegistryAccess = value
}
/**
* @param value The public domain names to use with the container service, such as example.com and www.example.com.
*/
@JvmName("vqsdparwtxmipodw")
public suspend fun publicDomainNames(`value`: Output>) {
this.publicDomainNames = value
}
@JvmName("kaanfmfvyeyslqti")
public suspend fun publicDomainNames(vararg values: Output) {
this.publicDomainNames = Output.all(values.asList())
}
/**
* @param values The public domain names to use with the container service, such as example.com and www.example.com.
*/
@JvmName("jasjjwhuagqivcvg")
public suspend fun publicDomainNames(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy