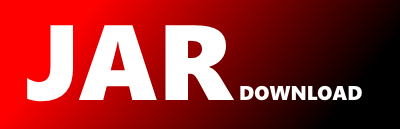
com.pulumi.awsnative.location.kotlin.ApiKeyArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.location.kotlin
import com.pulumi.awsnative.kotlin.inputs.TagArgs
import com.pulumi.awsnative.kotlin.inputs.TagArgsBuilder
import com.pulumi.awsnative.location.ApiKeyArgs.builder
import com.pulumi.awsnative.location.kotlin.inputs.ApiKeyRestrictionsArgs
import com.pulumi.awsnative.location.kotlin.inputs.ApiKeyRestrictionsArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Definition of AWS::Location::APIKey Resource Type
* @property description Updates the description for the API key resource.
* @property expireTime The optional timestamp for when the API key resource will expire in [ISO 8601 format](https://docs.aws.amazon.com/https://www.iso.org/iso-8601-date-and-time-format.html) .
* @property forceDelete ForceDelete bypasses an API key's expiry conditions and deletes the key. Set the parameter `true` to delete the key or to `false` to not preemptively delete the API key.
* Valid values: `true` , or `false` .
* > This action is irreversible. Only use ForceDelete if you are certain the key is no longer in use.
* @property forceUpdate The boolean flag to be included for updating `ExpireTime` or Restrictions details.
* Must be set to `true` to update an API key resource that has been used in the past 7 days. `False` if force update is not preferred.
* @property keyName A custom name for the API key resource.
* Requirements:
* - Contain only alphanumeric characters (A–Z, a–z, 0–9), hyphens (-), periods (.), and underscores (_).
* - Must be a unique API key name.
* - No spaces allowed. For example, `ExampleAPIKey` .
* @property noExpiry Whether the API key should expire. Set to `true` to set the API key to have no expiration time.
* @property restrictions The API key restrictions for the API key resource.
* @property tags An array of key-value pairs to apply to this resource.
*/
public data class ApiKeyArgs(
public val description: Output? = null,
public val expireTime: Output? = null,
public val forceDelete: Output? = null,
public val forceUpdate: Output? = null,
public val keyName: Output? = null,
public val noExpiry: Output? = null,
public val restrictions: Output? = null,
public val tags: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.location.ApiKeyArgs =
com.pulumi.awsnative.location.ApiKeyArgs.builder()
.description(description?.applyValue({ args0 -> args0 }))
.expireTime(expireTime?.applyValue({ args0 -> args0 }))
.forceDelete(forceDelete?.applyValue({ args0 -> args0 }))
.forceUpdate(forceUpdate?.applyValue({ args0 -> args0 }))
.keyName(keyName?.applyValue({ args0 -> args0 }))
.noExpiry(noExpiry?.applyValue({ args0 -> args0 }))
.restrictions(restrictions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.tags(
tags?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [ApiKeyArgs].
*/
@PulumiTagMarker
public class ApiKeyArgsBuilder internal constructor() {
private var description: Output? = null
private var expireTime: Output? = null
private var forceDelete: Output? = null
private var forceUpdate: Output? = null
private var keyName: Output? = null
private var noExpiry: Output? = null
private var restrictions: Output? = null
private var tags: Output>? = null
/**
* @param value Updates the description for the API key resource.
*/
@JvmName("vnxnnyeqcablekuw")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The optional timestamp for when the API key resource will expire in [ISO 8601 format](https://docs.aws.amazon.com/https://www.iso.org/iso-8601-date-and-time-format.html) .
*/
@JvmName("ccaimmjnaxwxotuw")
public suspend fun expireTime(`value`: Output) {
this.expireTime = value
}
/**
* @param value ForceDelete bypasses an API key's expiry conditions and deletes the key. Set the parameter `true` to delete the key or to `false` to not preemptively delete the API key.
* Valid values: `true` , or `false` .
* > This action is irreversible. Only use ForceDelete if you are certain the key is no longer in use.
*/
@JvmName("whrxhokqpfpursnh")
public suspend fun forceDelete(`value`: Output) {
this.forceDelete = value
}
/**
* @param value The boolean flag to be included for updating `ExpireTime` or Restrictions details.
* Must be set to `true` to update an API key resource that has been used in the past 7 days. `False` if force update is not preferred.
*/
@JvmName("tmxwinimjfuherng")
public suspend fun forceUpdate(`value`: Output) {
this.forceUpdate = value
}
/**
* @param value A custom name for the API key resource.
* Requirements:
* - Contain only alphanumeric characters (A–Z, a–z, 0–9), hyphens (-), periods (.), and underscores (_).
* - Must be a unique API key name.
* - No spaces allowed. For example, `ExampleAPIKey` .
*/
@JvmName("xsakjpoukpbkdnvf")
public suspend fun keyName(`value`: Output) {
this.keyName = value
}
/**
* @param value Whether the API key should expire. Set to `true` to set the API key to have no expiration time.
*/
@JvmName("vifrcarimppmahnm")
public suspend fun noExpiry(`value`: Output) {
this.noExpiry = value
}
/**
* @param value The API key restrictions for the API key resource.
*/
@JvmName("mtxkyucavjlejlpo")
public suspend fun restrictions(`value`: Output) {
this.restrictions = value
}
/**
* @param value An array of key-value pairs to apply to this resource.
*/
@JvmName("mhpnodiegsmwxkdw")
public suspend fun tags(`value`: Output>) {
this.tags = value
}
@JvmName("ytbmxyliljohjpmy")
public suspend fun tags(vararg values: Output) {
this.tags = Output.all(values.asList())
}
/**
* @param values An array of key-value pairs to apply to this resource.
*/
@JvmName("vcbwmjhgdbqhbwnr")
public suspend fun tags(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy